Top 12 Most Useful Typescript Utility Types
TypeScript adds a lot of useful utility types that help the developer do common type transformations.
Whether it's to make properties from a type optional, to create a new tuple, or to pick some properties from a type TypeScript has a utility type for that use-case.
In this article, I have compiled a list of the most useful utility types that TypeScript has to offer to a developer.
Let's get to it 😎.
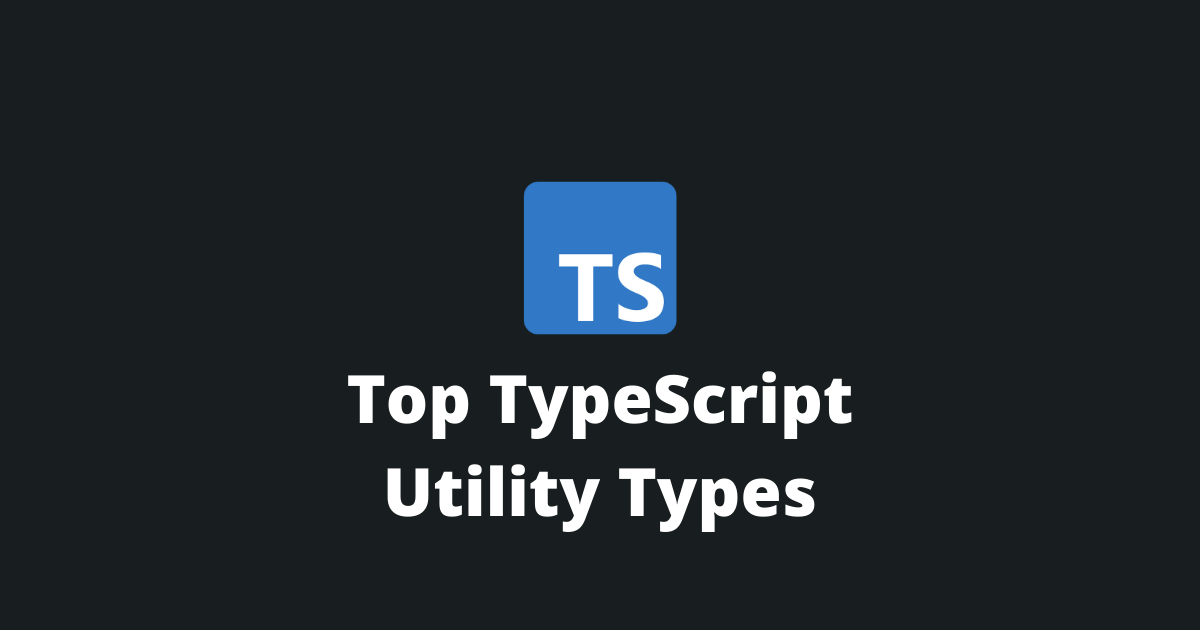
1. Pick<Type, Keys>
The Pick utility type lets you create a new type by picking properties from a Type.
You can select the properties that you want to pick by using a union type inside Keys.
Here is an example of the Pick utility type in action.
typescriptinterface IUser {
name: string;
userName: string;
password: string;
}
type Credentials = Pick<IUser, 'userName' | 'password'>;
const credentials: Credentials = {
password: 'pass123',
userName: 'tim',
};
In this example, we have created a new type Credentials from the type IUser and we only kept the password and userName fields.
If you are interested in learning more, I have written an extensive article about how does the pick type work in TypeScript.
2. Omit<Type, Keys>
The TypeScript omit utility type is the opposite of the Pick type.
It lets you construct a new type by omitting certain properties from a Type.
Here is an example of how the Omit utility type works.
typescriptinterface IUser {
firstName: string;
lastName: string;
userName: string;
password: string;
}
type FullName = Omit<IUser, 'password' | 'userName'>;
const fullName: FullName = {
firstName: 'Tim',
lastName: 'Mousk',
};
In this example, we have created a new type FullName from the type IUser and we removed the password and userName fields.
3. Required<Type>
The Required utility type lets you create a new type with all Type properties set to required.
Here is an example of how the Required utility type works.
typescriptinterface IUser {
firstName?: string;
lastName?: string;
}
type FullName = Required<IUser>;
const user: FullName = {
firstName: 'Tim',
lastName: 'Mousk',
};
In this example, we have created a new type FullName from the type IUser and we set all of its properties to required.
Read more: How do interfaces work in TypeScript?
4. Partial<Type>
The Partial utility type lets you create a new type with all Type properties set to optional.
The Partial utility type is very useful for mocking when you need to unit test your code. Also, it is useful when you want to update an object without updating all of its fields.
Here is an example the Partial utility type in action.
typescriptinterface IArticle {
content: string;
category: string;
date: Date;
}
type UpdatedArticle = Partial<IArticle>;
const updatedArticle: UpdatedArticle = {
content: 'updated content'
};
In this example, we have created a new type UpdatedArticle from the type IArticle and we set all of its properties to optional.
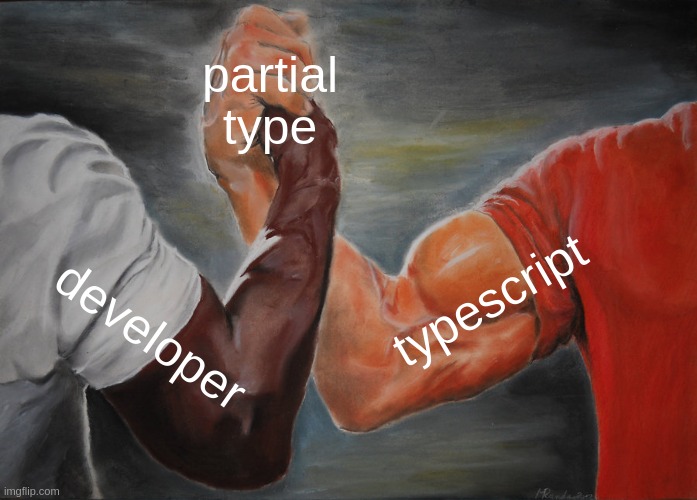
If you are interested in learning more, I have written an extensive article about how does the partial type work in TypeScript.
5. Record<Keys, Type>
The Record utility type lets you create a new type whose properties are Keys and values are Type.
Here is an example of how the Record utility type works.
typescripttype Status = 'info' | 'fail' | 'success';
type ImagesByStatus = Record<Status, string>;
const imagesByStatus: ImagesByStatus = {
fail: 'fail.png',
info: 'info.png',
success: 'success.png'
};
In this example, we have created a new type ImagesByStatus with properties from the union type Status. The type of each property value is string.
If you are interested in learning more, I have written an article about how does the record type work in TypeScript.
6. Readonly<Type>
The Readonly utility type lets you create a new type with all Type properties set to read-only.
This means that you will not be able to modify a property value.
Here is an example of the Readonly in action.
typescriptinterface IUser {
firstName: string;
lastName: string;
}
const user: Readonly<IUser> = {
firstName: 'Tim',
lastName: 'Mousk',
};
// Error
user.firstName = 'Tim';
In this example, when we try to reassign the firstName value we get a TypeScript compiler error since the object is read-only.
7. Parameters<Type>
The Parameters utility type lets you create a new tuple from the parameters types of a passed function.
Here is an example of the Parameters utility type.
typescript// Creates a tuple [ string, number]
type T1 = Parameters<(p1: string, p2: number) => void>;
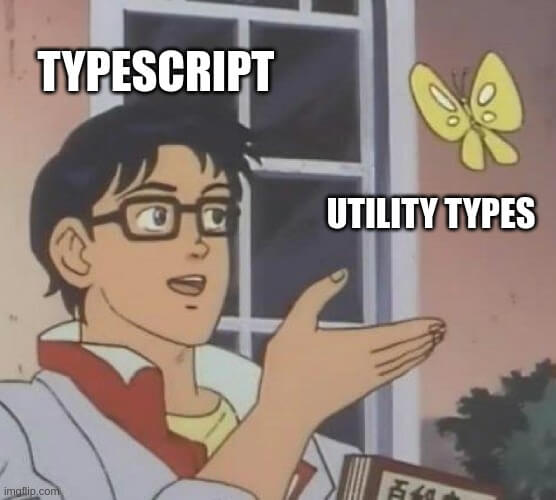
In this example, we have created a tuple from the parameters types of the passed function.
8. NonNullable<Type>
The NonNullable utility type lets you create a new type by excluding null and undefined from a passed Type.
Here is an example of the NonNullable utility type in action.
typescript// Returns number
type T1 = NonNullable<number | null | undefined>;
In this example, we create a new type T1 by excluding null and undefined.
9. Extract<Type, Union>
The extract utility type lets you extract all declared keys inside Union from Type .
Here is an example:
typescript// Returns 'a' | 'b'
type T1 = Extract<'a' | 'b' | 'c', 'a' | 'z' | 'b'>;
10. Exclude<Type, Union>
The Exclude utility type is the opposite of the Extract utility type.
The Exclude utility type lets you exclude all declared keys inside Union from Type .
Here is an example:
typescript// Returns 'c'
type T1 = Exclude<'a' | 'b' | 'c', 'a' | 'z' | 'b'>;
11. ReturnType<Type>
The ReturnType utility type lets you create a new type from the returned type of Type.
Here is an example:
typescript// Returns string
type T1 = ReturnType<() => string>;
In this example, the return type of Type is string. This means that T1 is of type string.
12. InstanceType<Type>
The InstanceType utility type lets you create a new type from the constructor function of Type.
Here is an example:
typescriptclass Coordinates {
x = 0;
y = 0;
z = 0;
}
// Returns Coordinates
type T1 = InstanceType<typeof Coordinates>;
I have written an in-depth guide about the TypeScript typeof keyword here.
Bonus - Intrinsic String Manipulation Types (Uppercase, Lowercase)
TypeScript also provides types for string manipulation.
Here is the list of the provided types:
- Uppercase
- Lowercase
- Capitalize
- Uncapitalize
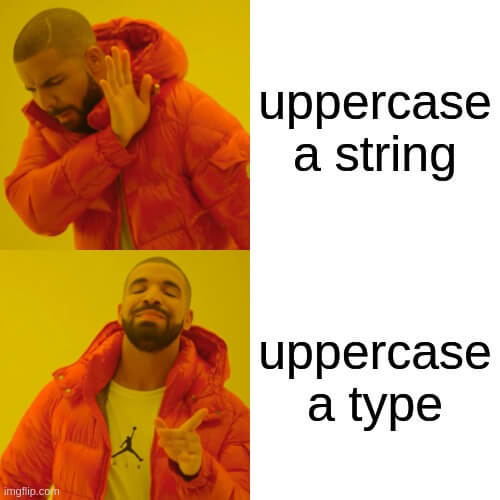
Here is an example of those utility types in action.
typescripttype FirstName = 'tim';
type LastName = 'MOUSK';
// TIM
type Uppercased = Uppercase<FirstName>;
// mousk
type Lowercased = Lowercase<LastName>;
// Tim
type Capitalized = Capitalize<FirstName>;
// mOUSK
type Uncapitalized = Uncapitalize<LastName>;
Final thoughts
As you can see, TypeScript offers utility types for pretty much all use-cases that a developer may encounter. And, the good thing is that TypeScript keeps adding more utility types with new updates!
Personally, the utility types that I use the most are the Partial and the Pick utility types. What are yours? 🤔.
I hope you liked the article, please share it with your fellow developers.