How Does The Extract Utility Type Work In TypeScript?
Sometimes, when developing a TypeScript project, a developer may need to extract types from a union type. Luckily, this operation is easy to do with the built-in Extract utility type.
The Extract<Type, Keys> utility type constructs a new type by extracting members assignable to Keys from the union Type.
This article explains the Extract utility type in TypeScript with code examples.
Let's get to it 😎.
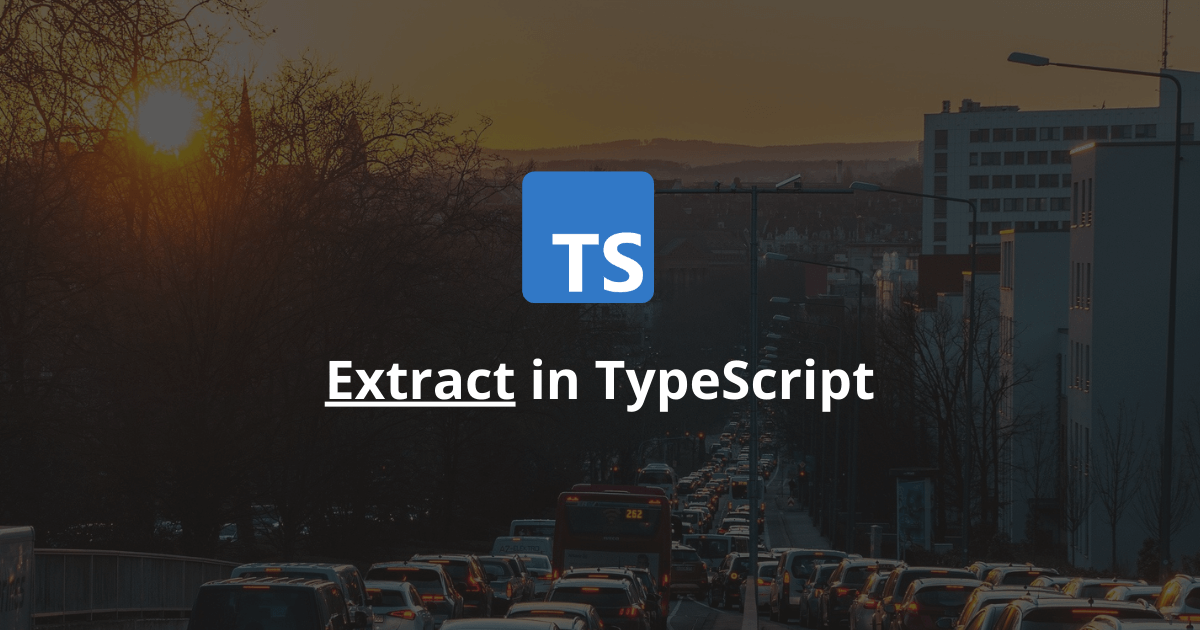
TypeScript provides many utility types to help developers manipulate and work with types. One of those utilities is the Extract utility type.
What is the Extract type?
The Extract<Type, Keys> utility type, added in TypeScript version 2.8, creates a new type by extracting members assignable to Keys from the union Type.
Confused? Let's review some examples to understand this utility better.
Let's say you have this union type:
typescripttype Role = 'ADMIN' | 'MANAGER' | 'USER';
Now let's say you want to extract some keys from that union type. One way of achieving this operation is to copy that union type and include only the keys you want. Of course, this method is not optimal. What if the original union type changes? That's why the Extract utility type exists.
Here is how you can extract the admin and manager keys from that union type:
typescript// type contains: 'ADMIN' | 'MANAGER';
type RoleExtracted = Extract<Role, 'ADMIN' | 'MANAGER'>;
And here is the full example:
typescripttype Role = 'ADMIN' | 'MANAGER' | 'USER';
// type contains: 'ADMIN' | 'MANAGER';
type RoleExtracted = Extract<Role, 'ADMIN' | 'MANAGER'>;
Cool right?
Note: Using the Extract utility type doesn't affect the original union type.
Note: The Extract utility type works similarly to the exclude utility type.
Another use case
Another use case of the Extract utility type is when you need to keep only SPECIFIC types from a union and filter out the rest.
Here is an example of this:
typescripttype Args = string | number | (() => string) | (() => number);
// type contains: (() => string) | (() => number)
type FunctionArgs = Extract<Args, Function>;
In this example, we filter out types that are not functions.
Final thoughts
As you can see, the Extract utility type is easy to use and important to understand to use TypeScript to its full potential.
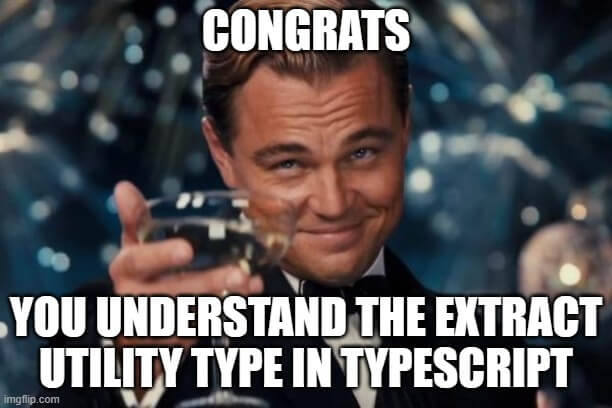
Here are some other TypeScript tutorials for you to enjoy: