How To Send The Bearer Token With Axios?
Nowadays, many APIs are not accessible without first authenticating. This helps to provide a safe and secure environment for the API users. After the authentication, the user can safely make calls to the API. But how do you authorize calls after the authentication? One way of achieving it involves sending a bearer token with your request to the API.
This article explains how to send the bearer token with the npm axios library and shows code examples.
Let's get to it 😎.
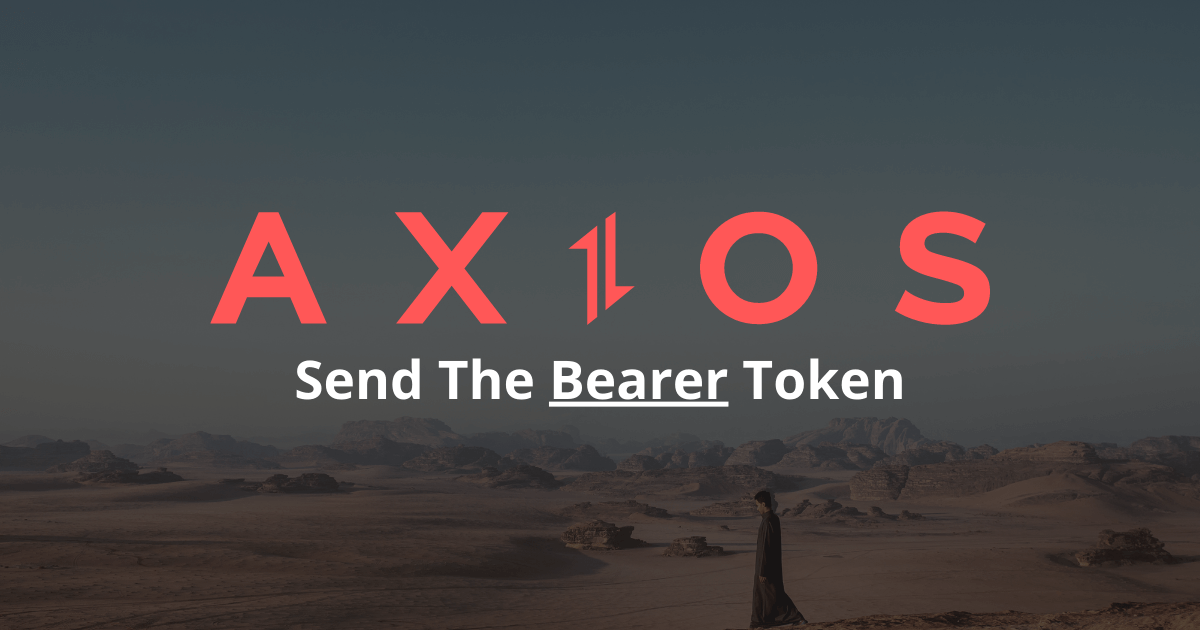
How to send the bearer token with an axios request?
To send a bearer token with an axios request, you need to pass an Authorization header to the request.
Here is the header you need to add to your request:
javascript{ Authorization: `Bearer ${token}` }
Here is an example of how to do this:
javascriptimport axios from 'axios';
const token = '{TOKEN}';
axios.request({
headers: {
Authorization: `Bearer ${token}`
},
method: "GET",
url: `https://jsonplaceholder.typicode.com/posts`
}).then(response => {
console.log(response.data);
});
As you can see, in this example we pass the bearer token to an axios GET request.
Note: As you can see, we are using jsonplaceholder.typicode.com, a free fake API that provides mocks.
But what if you need to pass the bearer token to every request? Is there a way to globally pass it to every axios request? Yes, there is!
Passing the bearer token to every request
To pass the bearer token to every axios request, you need to add a default header option to the axios global object.
Here is how you do it:
javascriptimport axios from 'axios';
const token = '{TOKEN}';
axios.defaults.headers.common = {
'Authorization': `Bearer ${token}`
};
All the axios requests will now have the Authorization bearer header!
You can also pass the authorization token in an axios interceptor.
javascriptimport axios from 'axios';
const axiosInstance = axios.create({
baseURL: process.env.REACT_APP_BASE_URL,
});
axiosInstance.interceptors.request.use(
(config) => {
const token = '{TOKEN}'
const auth = token ? `Bearer ${token}` : '';
config.headers.common['Authorization'] = auth;
return config;
},
(error) => Promise.reject(error),
);
In this case, only requests made with axiosInstance will have the Authorization bearer header.
Final thoughts
As you can see, passing an Authorization bearer header to an axios request is easy, but important to understand to complete all your projects and pass coding interviews.
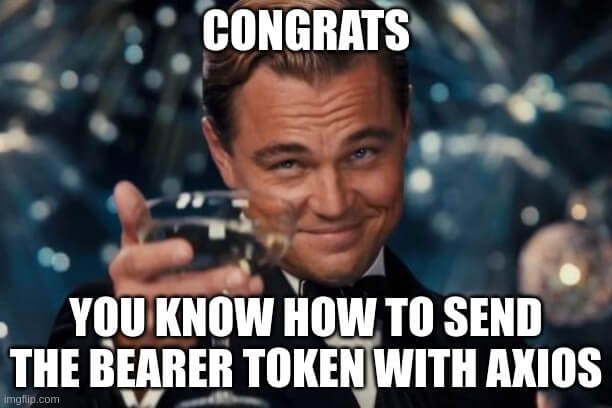
Here are some other JavaScript tutorials for you to enjoy: