How to get the time in JavaScript?
To get the time in JavaScript, you will need to work with the built-in Date object.
The Date object represents a moment in time in a platform-independent manner and has a lot of helper functions that will help the developer better manage the date and the time.
By the end of this article, you will know:
- How to get the current time.
- How to get the difference between two times.
- What library to use to format time.
Let's get to it 🙃!
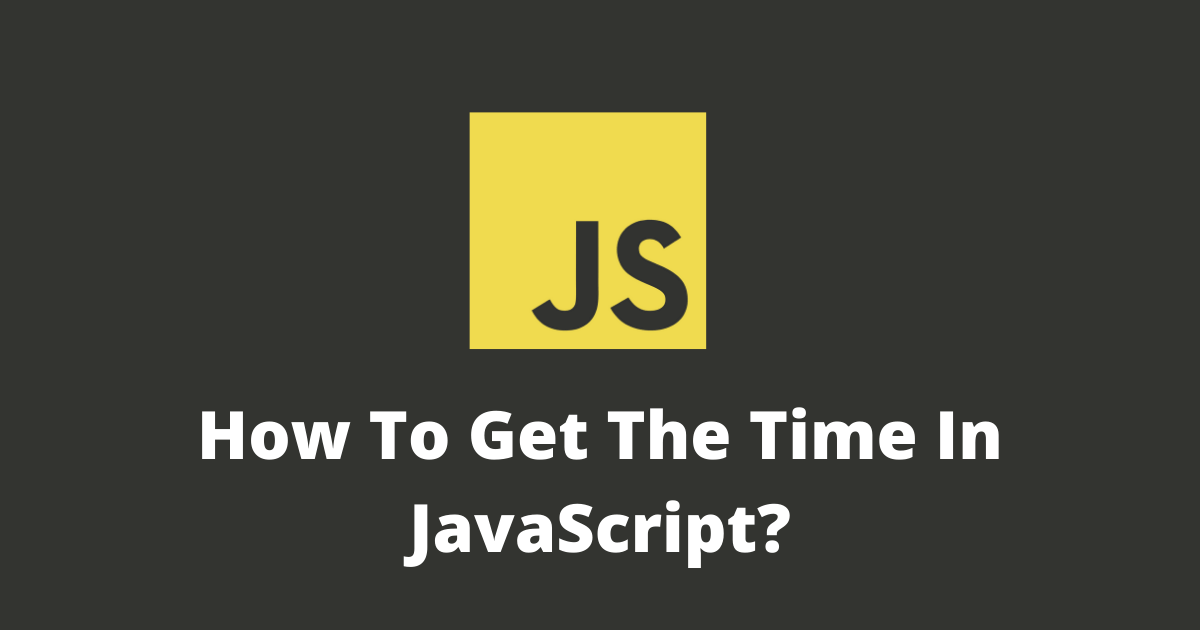
How to get the current timestamp?
To get the current timestamp you need to use the Date object.
You have a few options:
- Use the now() function.
javascriptconsole.log(Date.now());
- Utilize a date shorthand.
javascriptconsole.log(+ new Date());
- Use the getTime() function.
javascriptconsole.log(new Date().getTime());
How to get the current time in milliseconds?
In JavaScript, to get the current time in milliseconds, you need to use the getTime() built-in function of the Date object.
Since we want the current time, first we will need to get a new instance of the Date object.
Then we need to call the getTime() function.
javascriptconst current = new Date();
console.log(current.getTime());
This code will return the current time in milliseconds.
Notes:
The getTime() function is an ES1 feature and is supported by all browsers.
getTime() will return the milliseconds since Unix Epoch.
getTime() will always use UTC, this means that the result is timezone independent and will be the same across different parts of the world.
How to get the current time in seconds?
Since 1 second equals 1000 milliseconds, to get the current time in seconds you will need to divide the getTime() result by 1000.
javascriptconst current = new Date();
console.log(current.getTime() / 1000);
This code will return the current time in seconds.
How to get the difference between two times?
To get the difference between two times you need:
To get both the start and the end time in milliseconds (with the getTime() function).
Subtract the start time from the end time.
javascript// this is just an example.
const startDate = new Date('2021-12-01');
const endDate = new Date();
console.log(endDate.getTime() - startDate.getTime());
If you want the difference to be in seconds, just divide the result by 1000.
javascript// this is just an example.
const startDate = new Date('2021-12-01');
const endDate = new Date();
console.log((endDate.getTime() - startDate.getTime()) / 1000);
How to format time?
Formatting time in JavaScript can be a complex task. This is why I always use an npm library when I need to work with dates.
Lately, my favorite date npm library has become day-js.
I do not recommend using moment.js since it is a legacy project.
Here is an example of how to format a date in day-js.
javascriptdayjs('2021-12-25').format('DD/MM/YYYY');
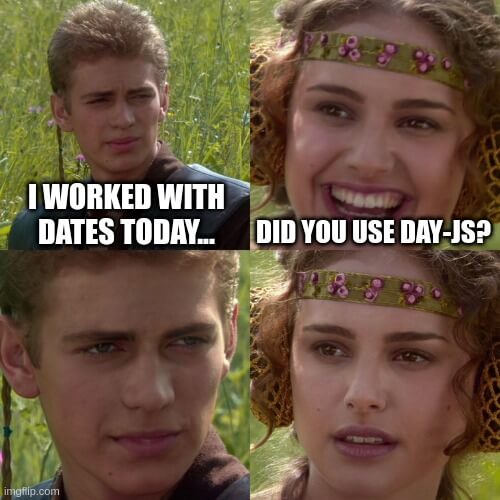
Day.js is:
- Very small (2kB)
- Has great support for internationalization.
- Is very simple to use.
Day.js supports a lot of different date formats and a lot of different locales, so you should definitely check it out.
Final Thoughts
Working with dates can be challenging especially when you need to support different locales.
That's why I always prefer to use a library like day-js when I need to work with dates.
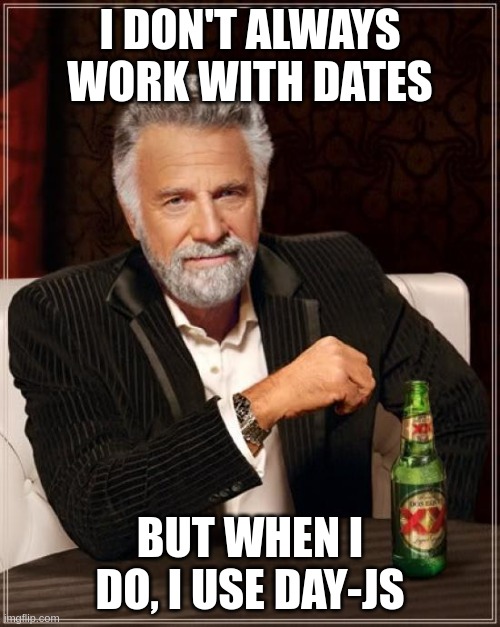
But, if you don't need locale support, the Date object is just fine.
Thank you for reading this article, please share it!