How To Set Up A Request Timeout In Axios?
Sometimes, when making requests with the axios library, the server to which you are making the call hangs, and the request takes forever to complete. For those cases, you need to set up a request timeout so your uses are not left hanging indefinitely.
This article explains setting up a request timeout with the npm axios library and shows code examples.
Let's get to it 😎.
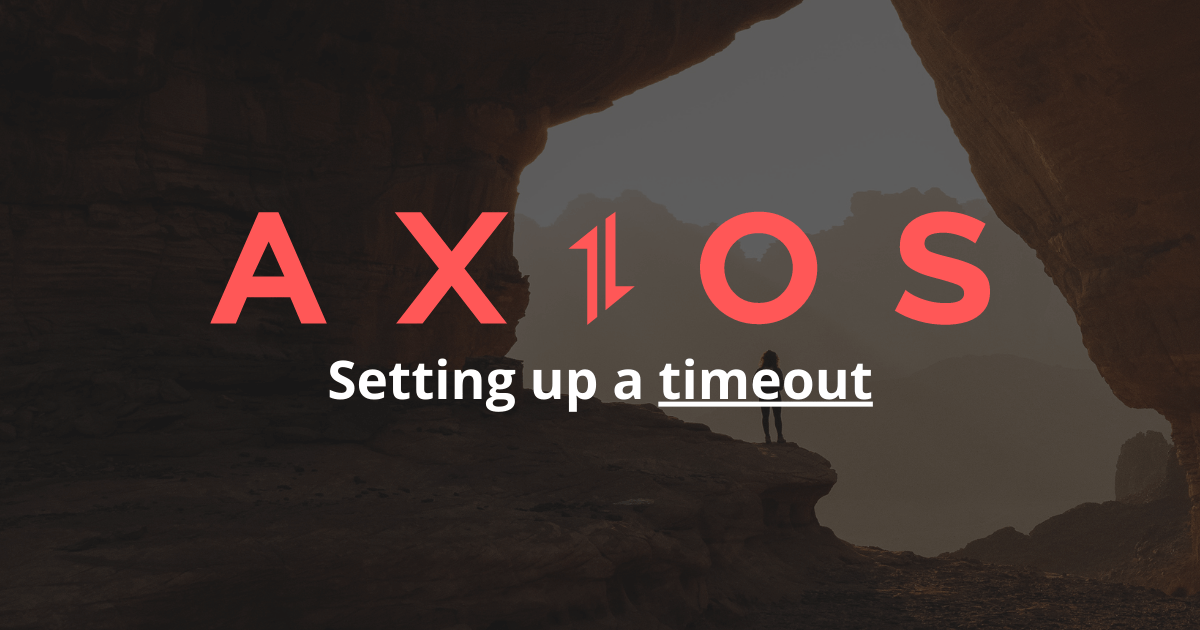
Setting up a response timeout
To set up the response timeout for an axios request, you need to pass the timeout property to the request configuration.
Here is an example of this:
javascriptimport axios from "axios";
axios
.request({
timeout: 2000,
method: "GET",
url: `https://jsonplaceholder.typicode.com/posts`
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
The timeout property accepts milliseconds (1000 milliseconds = 1 second).
In that example, we pass a timeout of 2000 milliseconds or 2 seconds.
If the server doesn't return a response within the time frame set by the timeout, axios will throw an error.
However, the timeout property only takes care of response timeouts. But, what about connection timeouts?
Note: By default, the timeout property value is 0, meaning no timeout at all.
Setting up a connection timeout
To set up the connection timeout for an axios request, you need to pass the signal property to the request configuration.
Here is an example of this:
javascriptimport axios from "axios";
axios
.request({
signal: AbortSignal.timeout(2000),
method: "GET",
url: `https://jsonplaceholder.typicode.com/posts`
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
In this example, we pass a connection timeout of 2000 milliseconds using the AbortSignal.timeout method (API provided in Node.js v17.3).
Or you can create a helper function like this:
javascriptimport axios from "axios";
function newAbortSignal(timeout) {
const abortController = new AbortController();
setTimeout(() => abortController.abort(), timeout || 0);
return abortController.signal;
}
axios
.request({
signal: newAbortSignal(2000),
method: "GET",
url: `https://jsonplaceholder.typicode.com/posts`
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
This example uses the AbortController API.
Combining it all
Now let's combine everything that we learned.
javascriptimport axios from "axios";
axios
.request({
timeout: 2000,
signal: AbortSignal.timeout(2000),
method: "GET",
url: `https://jsonplaceholder.typicode.com/posts`
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
In this example, we provide response AND connection timeouts to the axios request!
How to set up a default timeout globally?
If you want to set up a default timeout for every axios request, you need to assign a timeout to the axios global object.
Here is an example of this:
javascriptimport axios from "axios";
axios.defaults.timeout = 2000;
Now, every request will inherit this timeout.
Final thoughts
As you can see, passing a timeout to an axios request is easy! Use this knowledge well in your next project.
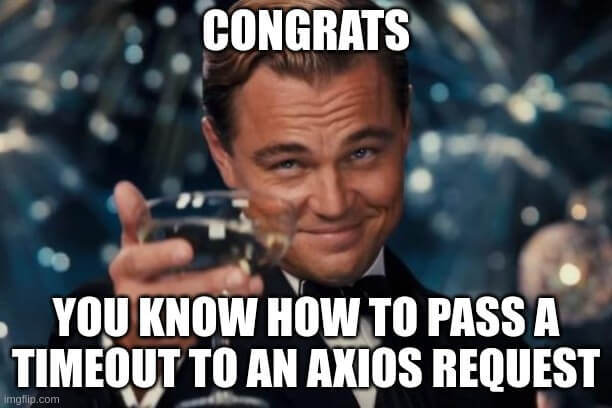
Here are some other JavaScript tutorials for you to enjoy: