How Does The Exclude Utility Type Work In TypeScript?
Sometimes, when developing a TypeScript project, developers need to exclude a member from an existing union type. Luckily, this operation is easy to do, using the Exclude utility type.
The Exclude<UnionType, ExcludedMembers> utility type, constructs a new type by excluding members defined in ExcludedMembers from a union type.
This article explains the Exclude utility type in TypeScript with code examples.
Let's get to it 😎.
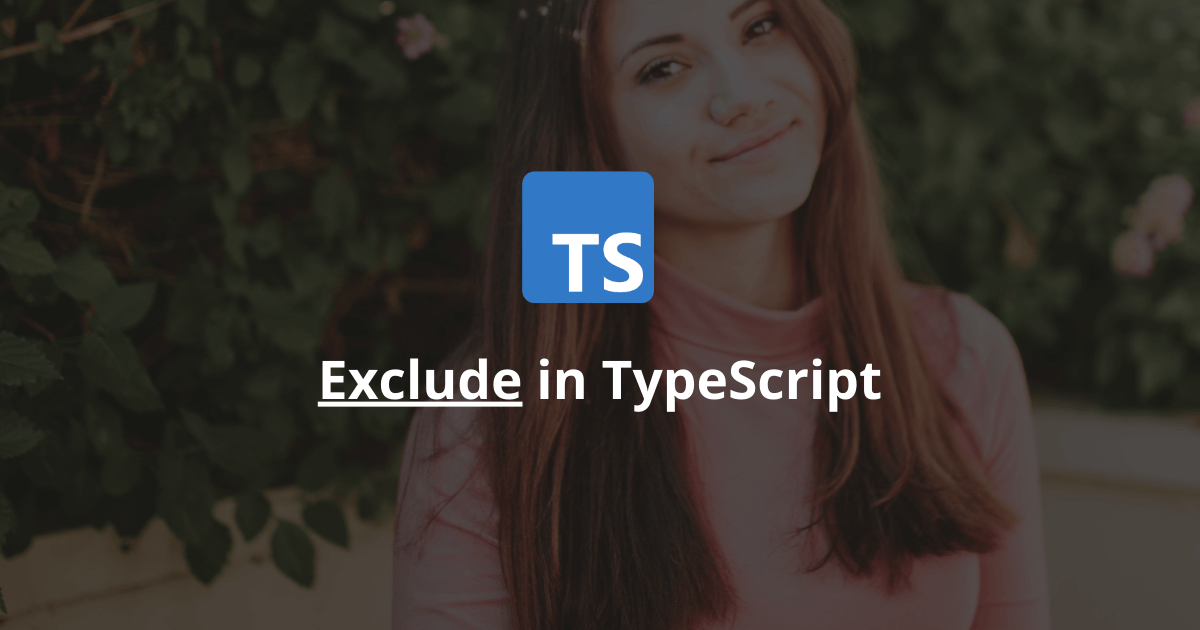
TypeScript provides many utility types, to help developers do common type transformations. One of those types is the Exclude utility type.
What is the Exclude type?
The Exclude<UnionType, ExcludedMembers> utility type, creates a new type by removing members defined in ExcludedMembers from a union type.
Let's review an example to understand this utility type usage better.
Let's say you have this union type:
typescripttype Role = 'ADMIN' | 'MANAGER' | 'CONTRIBUTOR';
And, you want to exclude CONTRIBUTOR from this union type.
One way of doing this task is to manually copy the union type WITHOUT the member that we want to exclude, like so:
typescripttype RoleExcluded = 'ADMIN' | 'MANAGER';
As you can see, this method is inefficient. What if the members change in the first union type? There must be a better way.
And there is, using the Exclude union type.
typescript// Type is: 'ADMIN' | 'MANAGER'
type RoleExcluded = Exclude<Role, "CONTRIBUTOR">;
As you can see, in this example we use the Exclude utility type to remove CONTRIBUTOR from the Role union type.
Cool right?
Here is the full example:
typescripttype Role = 'ADMIN' | 'MANAGER' | 'CONTRIBUTOR';
// Type is: 'ADMIN' | 'MANAGER'
type RoleExcluded = Exclude<Role, "CONTRIBUTOR">;
Final thoughts
As you can see, the Exclude utility type is simple to use and important to understand to use TypeScript to its full potential.
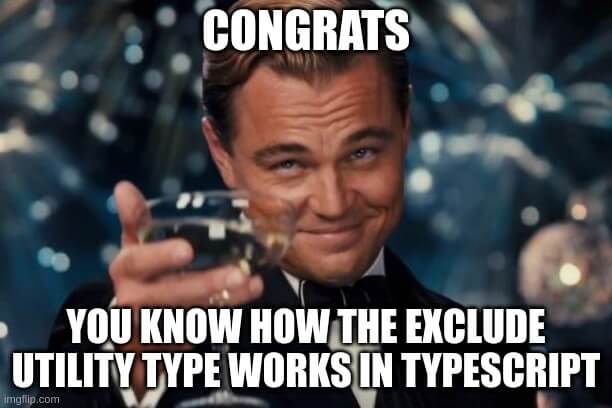
Here are some other TypeScript tutorials for you to enjoy: