How Does The TypeScript Omit Type Work?
TypeScript offers many different utility types that help transform other types. One of the most helpful utility types provided by TypeScript is the Omit type.
The Omit type creates a new type by excluding one or multiple properties from a type.
This article will go through everything to know about the TypeScript Omit type and provide answers to common questions.
Let's get to it 😎.
What is the Omit type?
The Omit<Type, Keys> type creates a new type by picking all the properties from Type and excluding the Keys.
Using this type, you can omit properties from both types and interfaces.
The Keys argument can accept single or multiple strings (union type).
The opposite of the Omit type is the Pick type.
Here is an example of how to omit a property from a type:
typescripttype UserModel = {
firstName: string;
lastName: string;
age: number;
}
type UserModelNameOnly = Omit<UserModel, 'age'>;
const nameOnly: UserModelNameOnly = {
firstName: 'Tim',
lastName: 'Mousk'
};
Here is an example of how to omit a property from an interface:
typescriptinterface IUserModel {
firstName: string;
lastName: string;
password: string;
}
type UserModelNameOnly = Omit<IUserModel, 'password'>;
The Omit type was released in TypeScript version 3.5.
Read more: How do interfaces work in TypeScript?
How do I omit multiple keys in TypeScript?
To omit multiple keys from a type, you can use the Omit type with a list of keys separated by the pipe operator (union type).
Here is an example of how to accomplish this:
typescriptinterface UserModel {
firstName: string;
lastName: string;
password: string;
age: number;
}
type UserModelNameOnly = Omit<UserModel, 'password' | 'age'>;
What is the difference between Omit and Exclude?
The most significant differences between Omit and Exclude are:
- The Omit type creates a new type by removing a property from a type.
- The Exclude type creates a new type by removing a constituent from a union type.
- The Omit type doesn't work on enums, but Exclude does.
- The Omit type is a combination of the Pick and the Exclude types.
Read more: The exclude utility type in TypeScript
How to omit values from an enum?
To omit values from an enum, you can use the Exclude type.
Here is an example of omitting values from an enum:
typescriptenum Animal {
Dog,
Cat,
Fish
}
type WithoutFish = Exclude<Animal, Animal.Fish>;
Note: Use the pipe operator to create a union type of animals to remove.
Final thoughts
As you can see, omitting properties from a type or an interface is no rocket science in TypeScript.
Just use the Omit type.
If you want to remove a value from a union literal, use the Exclude type.
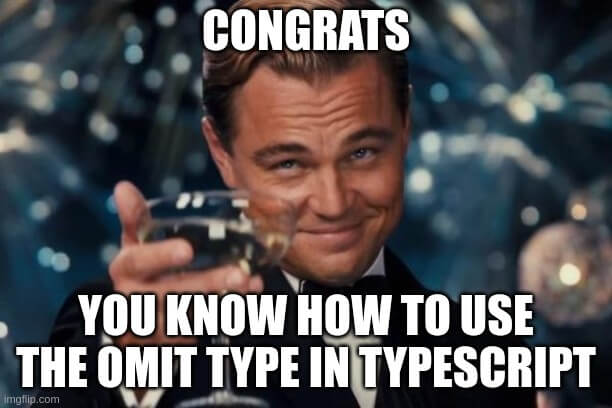
Here is another TypeScript tutorial for you to enjoy: