How To Override A Method In TypeScript?
TypeScript is a superset of JavaScript, and just like its counterpart, it supports object-oriented principles such as class inheritance. When a class inherits another class, a developer may want to override a parent class method. Luckily, this is easy to accomplish in TypeScript.
This article will focus on explaining how to override a class method in TypeScript and give answers to common questions.
Let's get to it 😎.
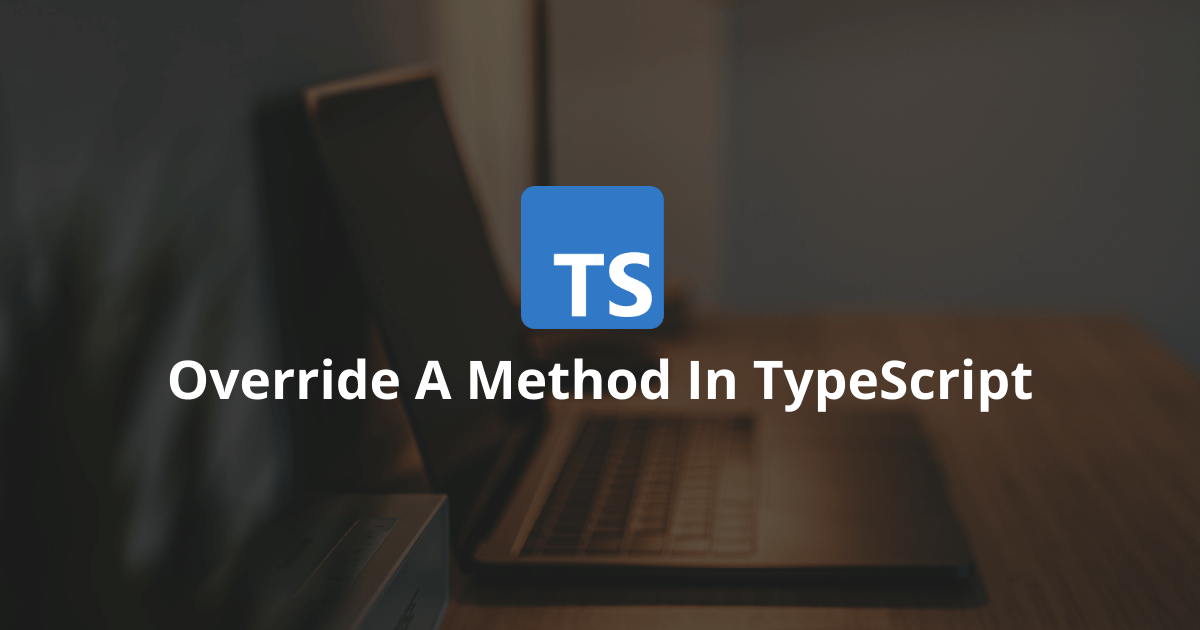
What is class inheritance?
In object-oriented languages, class inheritance refers to the ability of a child class to inherit properties and methods from a parent class (or super class).
In JavaScript, to inherit a class, you need to use the extends keyword.
Also, you can point to the parent class from the child class using the super keyword.
Here is an example of class inheritance in TypeScript:
typescriptclass Car {
public constructor() {
console.log('init car');
}
public drive(): void {
console.log('drive');
}
}
class Ford extends Car {
public constructor() {
super();
}
public getName(): void {
console.log('Ford');
}
}
In this example, we create a child class called Ford that extends a parent class called Car.
How to override a method?
TypeScript allows overriding a parent's method from a child's class easily.
However, both function declarations must match (return type and parameters).
Otherwise, the TypeScript compiler will give an error.
Here is an example of overriding a method in TypeScript:
typescriptclass Car {
public drive(km: number): void {
console.log(`Your car drove ${km}km`);
}
}
class Ford extends Car {
public drive(km: number): void {
console.log(`Your Ford drove ${km}km`);
console.log('override method')
}
}
const ford = new Ford();
// Output: "Your Ford drove 2km"
// Output: "override method"
ford.drive(2);
As you can see, we override the parent drive function in the child class called Ford.
The drive function of the parent Car class is not called.
However, if you wish to call the parent's function implementation inside the child's class, you can use super.drive.
Note: Here we are using string interpolation to concat the strings.
What is the override keyword?
The override keyword ensures that the function exists in the parent's class.
It is particularly useful with an abstract class.
It also helps reduce typos and helps the readability of your code.
This is an optional keyword. You can override a function without it.
Here is the same example but using the override keyword this time:
typescriptclass Car {
public drive(km: number): void {
console.log(`Your car drove ${km}km`);
}
}
class Ford extends Car {
public override drive(km: number): void {
console.log(`Your Ford drove ${km}km`);
console.log('override method')
}
}
If you want to make this keyword required, enable the noImplicitOverride options in the tsconfig.json file of your project.
How to override a private method?
If you want to override a parent's function, its access modifier must be public or protected.
If the parent's function is private, you will not be able to override it.
Change the function access modifier to protected or public to override it.
Final thoughts
As you can see, a developer can easily override a parent's method in TypeScript.
I always recommend using the override keyword when you override a function.
This helps with readability and reduces code errors and typos.
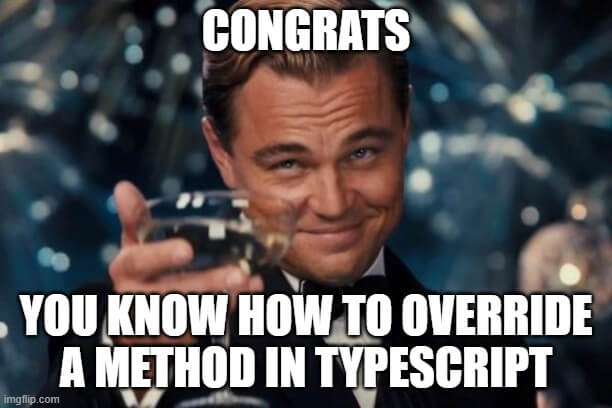
Thank you for reading.