How Does An Abstract Class Work In TypeScript?
TypeScript, just like such languages as C# or Java, provides support for abstract classes. It is a valuable feature since JavaScript doesn't support the abstract keyword. This brings the question of what is an abstract class and how it works in TypeScript.
An abstract class defines common methods for child classes. You cannot directly instantiate an abstract class.
This article will go over everything to know about abstract classes in TypeScript and answer some of the most common questions.
Let's get to it 😎.
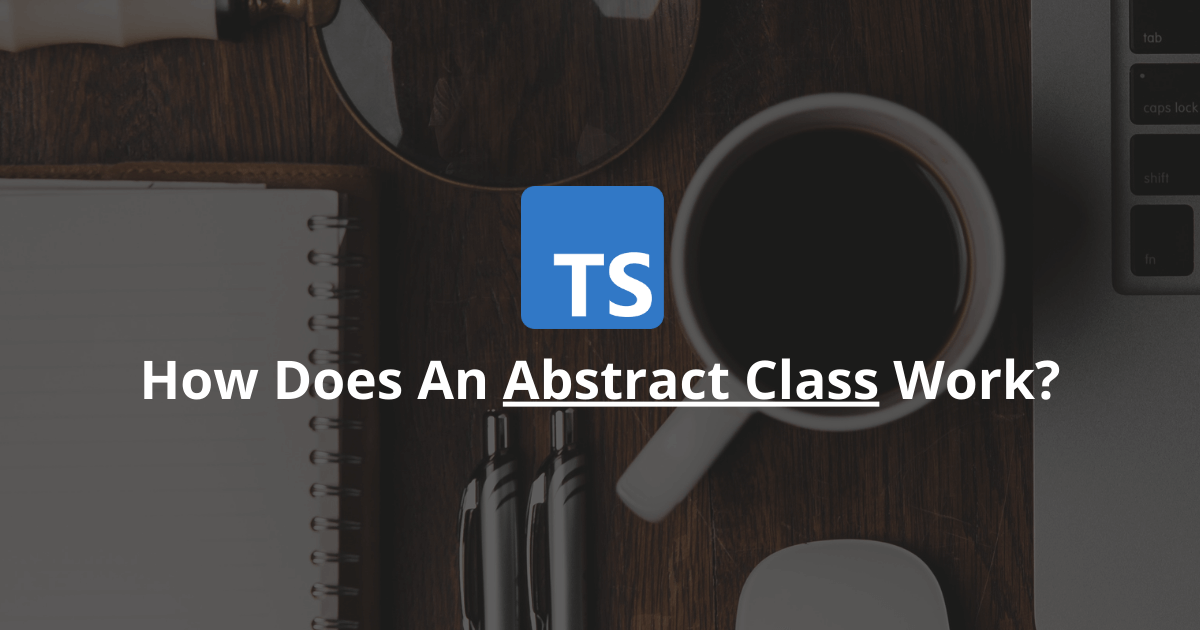
The definition
An abstract class is a sealed class that cannot be instantiated directly.
To use an abstract class, you must extend it.
In TypeScript, a developer defines an abstract class using the abstract keyword.
An abstract class can contain:
- One or multiple abstract methods.
- One or numerous property declarations.
- One class constructor.
If the abstract class contains abstract methods, the child class must define all of them.
An abstract class is useful when:
- Different closely related child classes will use the functionality inside a class.
- You don't want developers to be able to instantiate a class.
Here is an example implementation of a custom abstract class:
typescriptabstract class Animal {
public constructor() {
console.log('animal init');
}
}
class Dog extends Animal {
public makeSound(): void {
console.log('bark');
}
}
// Outputs: "animal init"
const dog = new Dog();
In this example, we define a simple Animal abstract class with a constructor.
Then we create a child Dog class that extends the Animal class.
Note: You can only instantiate the Dog class. If you try to instantiate the Animal class, the TypeScript compiler will output an error.
How to define an abstract method?
An abstract method is a method the child class HAS to implement.
An abstract method must be declared with the abstract keyword and must have no body.
Here is an example of an abstract method in TypeScript:
typescriptabstract class Animal {
public abstract makeSound(): void;
}
class Dog extends Animal {
public override makeSound(): void {
console.log('bark');
}
}
const dog = new Dog();
// Outputs: 'bark'
dog.makeSound();
In the child class, I recommend using the override keyword before defining an abstract method to make it explicit about what you are doing for other developers.
How to define an abstract property?
An abstract property is a property the child class HAS to implement.
Here is an example of an abstract property in TypeScript:
typescriptabstract class Animal {
public abstract type: string;
}
class Dog extends Animal {
public override type = 'dog';
}
const dog = new Dog();
// Outputs: 'dog'
console.log(dog.type);
In this example, the Dog class extends the Animal class and defines the type property.
Difference between abstract class vs interface
The most significant differences between an abstract class and an interface are:
- An interface is a contract that defines the properties and methods that an object has to implement. It does not exist at runtime.
- An abstract class is a sealed class that cannot be instantiated directly. It can contain real method implementations and abstract methods and properties. It exists at runtime.
Think of it this way:
- An interface is a contract of methods and properties that we PROMISE to define later.
- An abstract class is a definition of what an object is.
Final thoughts
As you can see, it is easy to define an abstract class in TypeScript.
Simply use the abstract keyword, and voila.
When defining abstract methods in child classes, I recommend using the override keyword. This helps with code clarity and refactoring.
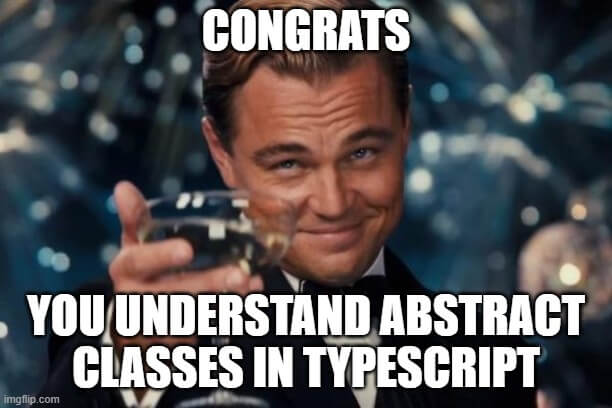
Here are some other TypeScript tutorials for you to enjoy: