How Does A Static Class Work In TypeScript?
TypeScript, just like JavaScript, provides support for static methods, properties, and initialization blocks. This brings the question of what a static class is and how it works in TypeScript.
A static class contains static properties and methods and is sealed. The developer cannot instantiate it.
This article will go through everything to know about static classes in TypeScript and provide answers to common questions.
Let's get to it 😎.
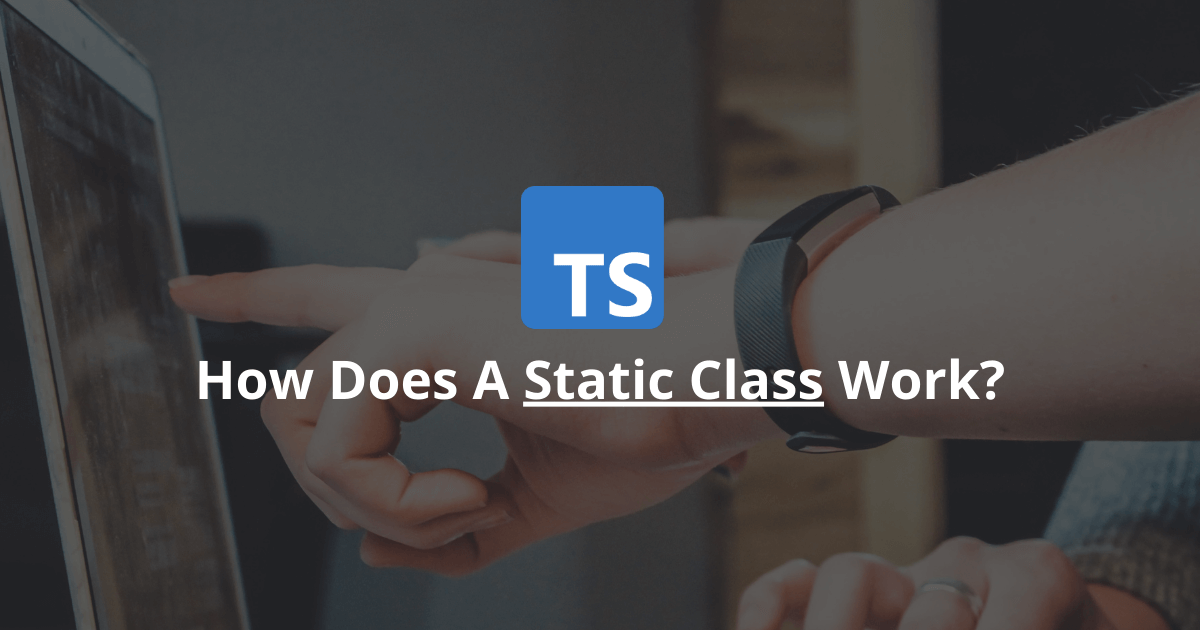
The definition
In TypeScript, a static class is a sealed class that cannot be instantiated.
A static class can only contain static members:
- Static properties
- Static methods
- Static constructor
Since a static class cannot have an instance variable, the developer uses the class name directly to call the methods and access the properties.
A static class is useful when:
- The class only operates with input parameters.
- The class doesn't need to set or get internal instance fields.
For example, a static class is a great choice when a developer needs to create a utility class (think of the JavaScript Math class).
Here is an example implementation of a custom static class:
typescriptabstract class Utils {
public static pi: number = 3.14;
public static calculate (radius: number): number {
return this.pi * radius;
}
}
In this example, you must use the Utils class name directly to call the calculate method or to access the pi property.
Unfortunately, TypeScript doesn't yet support the static keyword at a class level.
That's why, to make the class non-instantiable, a developer can use the abstract class keyword.
This way, other developers won't be able to instantiate this class.
Note: When you use the this keyword inside a static member, it refers to the class (and not a class instance).
How to define a static property?
To define a static property in TypeScript, you need to use the static keyword before the property name declaration, like so:
typescriptclass Utils {
public static pi: number = 3.14;
}
Note: The access modifier before the static keyword is optional.
To access this property, use the class name like so:
typescript// Outputs: 3.14
console.log(Utils.pi);
Note: Static properties are shared among the instances of the class.
How to define a static method?
To declare a static method, you must also use the static keyword before the method name declaration, like so:
typescriptclass Utils {
public static calculate (radius: number): number {
return radius * 2;
}
}
Static methods are also shared across class instances.
How to define a static constructor?
Unfortunately, TypeScript does not currently support static constructors.
However, you can use static blocks, a new ES2022 feature that helps initialize static classes.
Here is an example of how to initialize a static class:
typescriptclass Utils {
static {
console.log('static class init');
}
}
Another option to initialize a class is to create a static initialization method that you call before using the class.
How to define a static property on an interface?
Unfortunately, there is no method to define a static property on an interface in TypeScript.
Final thoughts
As you can see, TypeScript offers good support for static classes.
When declaring a static class, you must use the static keyword before declaring a method or a property.
Also, using the initialization blocks, you can initialize a static class.
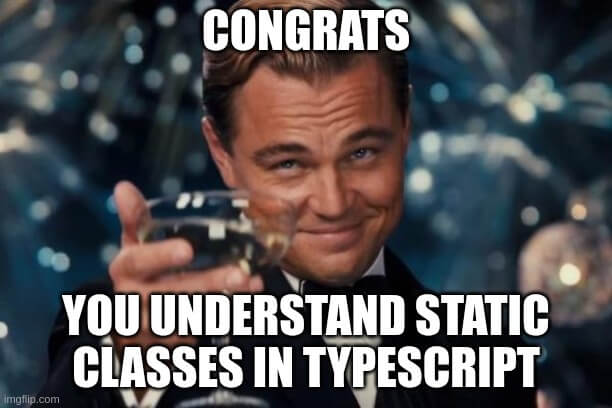
Here are other TypeScript tutorials for you to enjoy: