How to fix "object is possibly null" in TypeScript?
TypeScript adds optional type checking capabilities to JavaScript. Indeed, code written in TypeScript is checked for error before it is executed, saving development and debugging time. One of the potential errors that a developer might encounter is the TypeScript "object is possibly null" error.
One of the methods to fix the "object is possibly null" error in TypeScript is to use the optional chaining operator, like so:
typescriptinterface IBook {
author?: {
name?: string;
} | null;
}
const book: IBook = {};
// Notice the question mark operator
console.log(book.author?.name);
// Outputs: 'undefined'
By the end of this article, you will know why the "object is possibly 'null' or 'undefined'" error happens and different ways to fix it.
Let's get to it 😎.
Why does this error happen?
This error, also called TS2533, happens if an object or a property might be null or undefined.
It happens at compile time due to the TypeScript strictNullChecks flag set to true.
Here is an example of this error:
typescriptinterface IBook {
author?: {
name?: string;
} | null;
}
const book: IBook = {};
// TS2533: Object is possibly 'null' or 'undefined'
console.log(book.author.name);
Luckily, there are multiple ways of fixing this error.
Read more: How do interfaces work in TypeScript?
How to fix this error with the optional chaining operator?
The optional chaining operator (?.), introduced in TypeScript 3.7, lets the developers safely access deeply nested properties by checking for null and undefined values.
Here is an example of the optional chaining operator in action:
typescriptlet arr: number[] | undefined | null;
// Outputs: undefined
console.log(arr?.length);
If a property that we want to access does not exist, the result of the expression will be undefined.
How to fix this error with the non-null assertion operator?
The non-null assertion operator (!.), also called the exclamation mark operator, indicates to the compiler that we are sure that the value we want to access is not null or undefined.
Here is an example of the non-null assertion operator in action:
typescriptconst myName = 'Tim' as string | undefined;
// Outputs: 3
console.log(myName!.length);
How to fix this error with the nullish coalescing operator?
If the left operand is null or undefined, the nullish coalescing operator (??) returns the right one.
Here is the nullish coalescing operator in action:
typescriptlet myName: string | undefined | null
console.log(myName ?? 'Tim');
In this example, we provide a default value for the myName variable.
How to fix this error with an if statement?
For old-school folks, you can use a simple if statement.
typescriptconst myName = 'Tim' as string | undefined;
if (typeof myName === 'string') {
// Outputs: 3
console.log(myName.length);
}
Read more: The typeof operator in TypeScript
Final thoughts
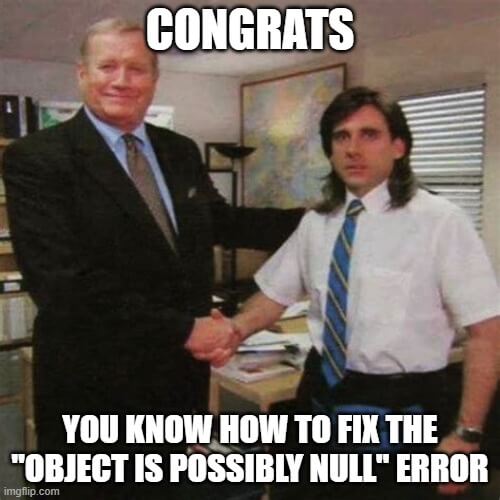
As you can see, you have multiple ways of fixing the "object is possibly null" error.
For my part, to fix this error, I usually use the optional chaining operator.
You can also disable TypeScript "strict null checks" in the tsconfig.json file. By doing so, the compiler will not output this error anymore. However, I don't recommend doing so because that may introduce new bugs into your code.
Finally, here are some other TypeScript tutorials that I've written: