How To Use The Double Question Mark Operator In TypeScript?
If you've been writing TypeScript code for long enough, you've undoubtedly seen occurrences of the double question mark operator.
The double question mark operator or nullish coalescing operator helps assign a default value to a null or undefined TypeScript variable.
This article explains the double question mark operator and shows various code examples.
Let's get to it 😎.
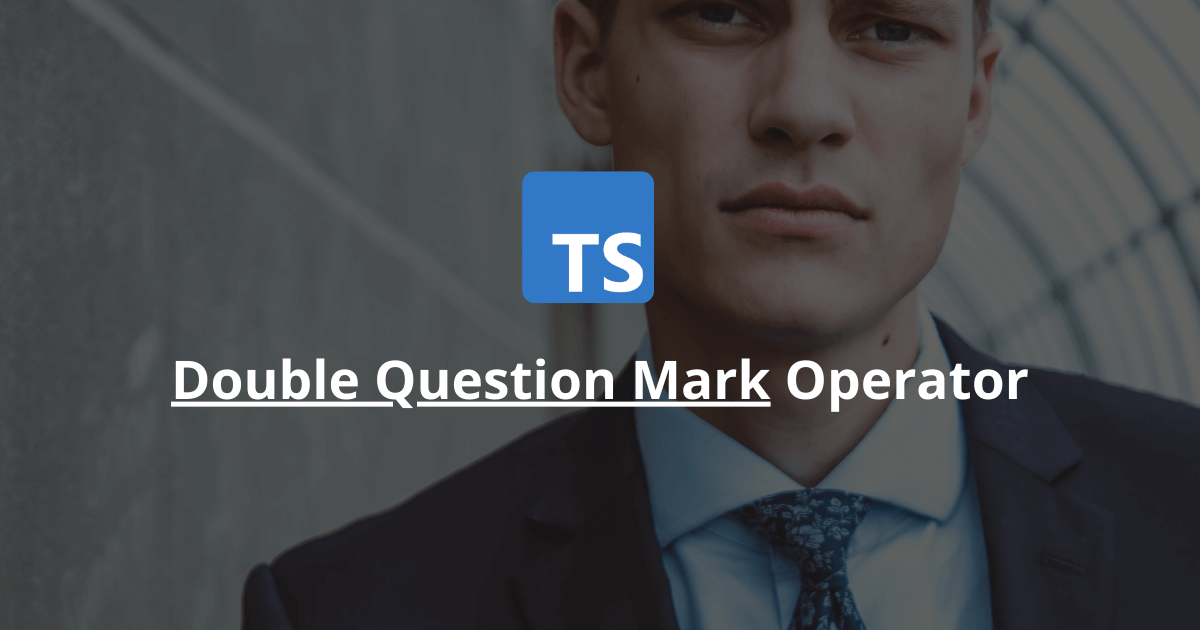
The definition
The nullish coalescing or double question mark operator provides a default value for a null or undefined variable.
This feature exists in JavaScript and TypeScript (added in version 3.7).
Here is an example:
typescriptconst myVar = null;
const ex = myVar ?? 'default';
// Outputs: 'default'
console.log(ex);
When the left-hand operand is undefined or null, the double question mark operator returns the right-hand operand (default value). Otherwise, it returns the left-hand operand.
This previous example can also be written as:
typescriptconst myVar = null;
let ex: any = myVar;
if (ex != null && ex != undefined) {
ex = myVar;
} else {
ex = 'default';
}
// Outputs: 'default'
console.log(ex);
As you can see, using the nullish coalescing operator is much simpler.
Although this can hurt the readability of your code, you can combine multiple nullish coalescing operators.
Difference between double question mark VS double bar
Since both operators provide default fallbacks, many developers confuse them.
The difference between the double question mark and the double bar operators is:
- The double question mark operator fallbacks when the value of the left-hand operand is null or undefined.
- The double bar operator fallbacks when the value of the left-hand operand is falsy (null, undefined, 0, '', NaN, false).
Here is how the double question mark operator behaves:
typescriptconsole.log(null ?? "default"); // "default"
console.log(undefined ?? "default"); // "default"
console.log(false ?? "default"); // false
console.log("" ?? "default"); // ""
console.log(0 ?? "default"); // 0
console.log(42 ?? "default"); // 42
As you can see, the nullish coalescing operator falls back to the default value ONLY when the variable is null or undefined.
Here is how the double bar operator behaves:
typescriptconsole.log(null || "default"); // "default"
console.log(undefined || "default"); // "default"
console.log(false || "default"); // "default"
console.log("" || "default"); // "default"
console.log(0 || "default"); // "default"
console.log(42 || "default"); // 42
As you can see, the double pipe operator falls back to the default value when the variable is falsy.
Final thoughts
As you can see, the nullish coalescing or double question mark operator becomes handy when a developer needs to provide a default value for a null or undefined variable.
To ensure a variable is falsy (in simple terms defined), you must use the double pipe operator.
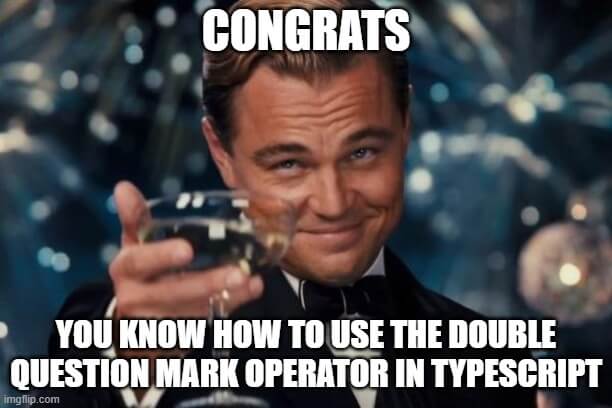
Here are some other TypeScript tutorials for you to enjoy: