How To Create A Global Variable In TypeScript?
Sometimes, a TypeScript developer may want to add a global variable to the code base. Luckily, this is easy to do.
To add a new global variable to TypeScript, you can:
- Augment the global object (when running in a Node.js context).
- Augment the window object (when running in a browser context).
This article explains both methods and shows how to code them with examples.
Let's get to it 😎.
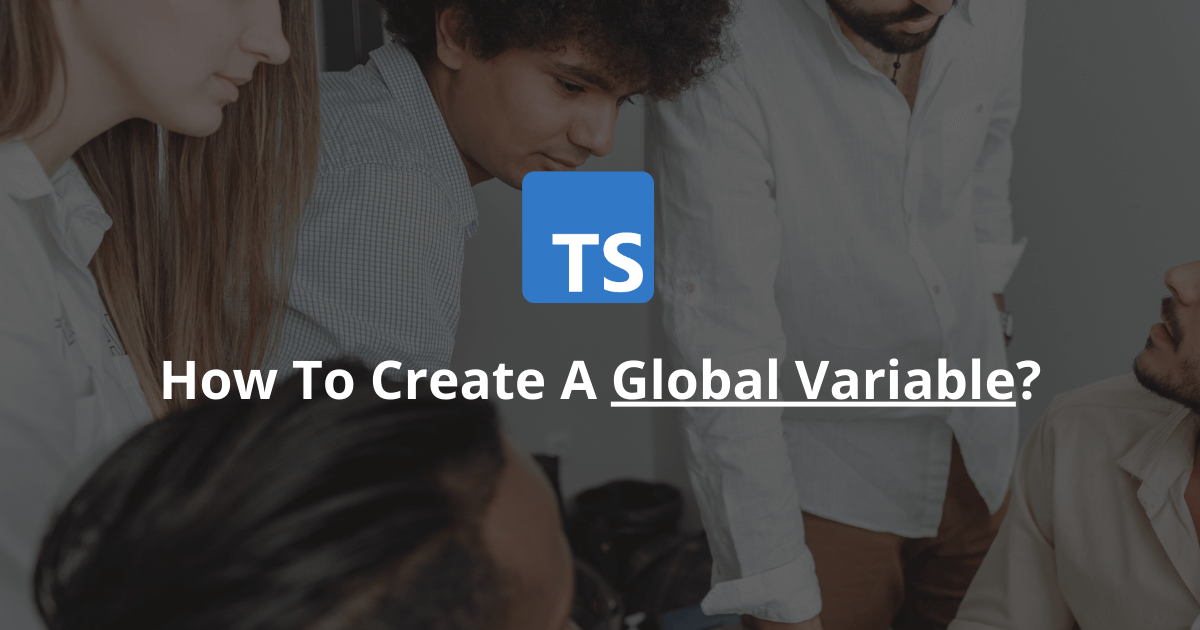
Method #1 - Augment the global object (Node.js)
If you are running your TypeScript code in a Node.js context, the easiest way to declare a global variable involves using the global object.
Here is how to do it:
1. Add the new property to the global object.
typescriptglobal.__GLOBAL_VAR__ = {
name: 'Global'
};
If you get the error: Cannot find name 'global', add the node type to the compilerOptions.types on your tsconfig.json file.
However, you will still get an error because the new property doesn't exist inside TypeScript's definition of the global object. That's why we need to augment it.
2. Create a new file called definitions.d.ts that will hold all the definitions.
3. Augment the global object with the new property definition inside the new file.
typescriptdeclare global {
var __GLOBAL_VAR__: {
name: string;
};
}
export {};
You can now use this variable anywhere in your TypeScript code.
Method #2 - Augment the window object (Browser)
If you are running your TypeScript code inside a browser, the easiest way to declare a global object involves using the JavaScript window object.
Here is how to do it:
1. Add the new property to the window object.
typescriptwindow.__GLOBAL_VAR__ = {
name: 'Global'
};
However, you will get this error: Property '__GLOBAL_VAR__' does not exist on type 'Window & typeof globalThis'.
This error happens because the property doesn't exist inside the window interface.
Let's augment the window interface to fix this error.
2. Create a new definition file called definitions.d.ts.
3. Add the property to the window object definition inside the new file.
typescriptdeclare global {
interface Window {
__GLOBAL_VAR__: {
name: string;
};
}
}
export {};
You can now use the __GLOBAL_VAR__ property anywhere on your HTML page.
Final thoughts
As you can see, adding a global variable to your TypeScript code is easy.
Depending on the running context, you can augment the window or the global object with the new property.
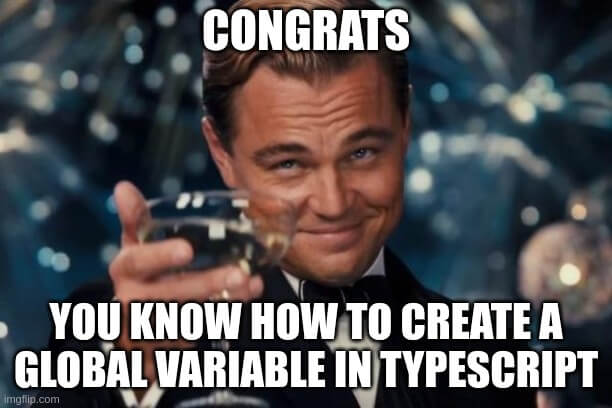
Here are some other TypeScript tutorials for you to enjoy: