How Does The While Loop Work In TypeScript?
TypeScript offers multiple loop types to iterate through a piece of code. Some are well known and used a lot, like the for loop. Others are less used, like the while loop.
The while loop runs some code while a specific condition is true.
Here is an example where the while loop executes some code five times:
typescriptlet i = 0;
while (i < 5) {
console.log("Current index - " + i);
i++;
}
This article shows how the while loop works in TypeScript with code examples.
Let's get to it 😎.
The definition
The while loop is a TypeScript control statement that executes a block statement until a specific condition is true. When the specific condition becomes false, the while loop exits.
Here is the syntax of the while loop:
typescriptwhile (condition) {
// code block
}
Here are the steps when a while loop executes:
- The condition is evaluated.
- If it is false, the while loop exists.
- If the condition is true, the block statement is executed.
- Go back to step one.
Let's do a small example to understand the while loop better:
typescriptlet index = 1;
while (index <= 5) {
console.log("Your index - " + index);
index++;
}
// Outputs: Your index - 1
// Outputs: Your index - 2
// Outputs: Your index - 3
// Outputs: Your index - 4
// Outputs: Your index - 5
In this code example, the while loop outputs the current index and runs till the index variable is less or equal to five.
Note: The browser crashes if the condition never becomes false.
How to break from a while loop?
When you want to break from a while loop, you need to use the break keyword.
Here is an example:
typescriptlet index = 1;
while (index <= 5) {
if (index === 3) {
break;
}
console.log("Your index - " + index);
index++;
}
// Outputs: Your index - 1
// Outputs: Your index - 2
In this example, we break from the while loop when the index variable is equal to three.
How to skip one iteration in a while loop?
When you want to skip one iteration in a while loop, you need to use the continue keyword.
Here is an example:
typescriptlet index = 0;
while (index < 5) {
index++;
if (index === 3) {
continue;
}
console.log("Your index - " + index);
}
// Outputs: Your index - 1
// Outputs: Your index - 2
// Outputs: Your index - 4
// Outputs: Your index - 5
In this example, we skip one iteration of the while loop when the index variable is equal to three.
That's why index number three is not printed.
Final thoughts
As you can see, the while loop is excellent when you need to execute some code several times, BUT you don't know exactly how many times.
Although the while loop is less known than the for loop, it is still essential to understand it well.
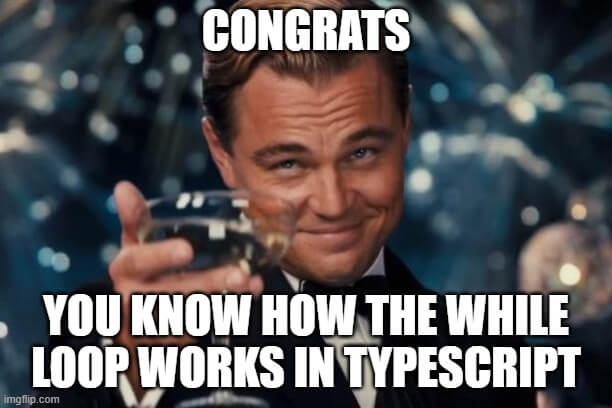
Here are some other TypeScript tutorials for you to enjoy: