How To Transform An Enum Into An Array In TypeScript?
Enums are useful when you have a fixed set of constants. Sometimes, a developer needs to transform a TypeScript enum into an array. Luckily, this is easy to do.
To transform an enum into an array in TypeScript, you can use:
- The Object.values function.
- The Object.keys function.
This article shows how to transform a numeric or string enum into an array with code examples.
Let's get to it 😎.
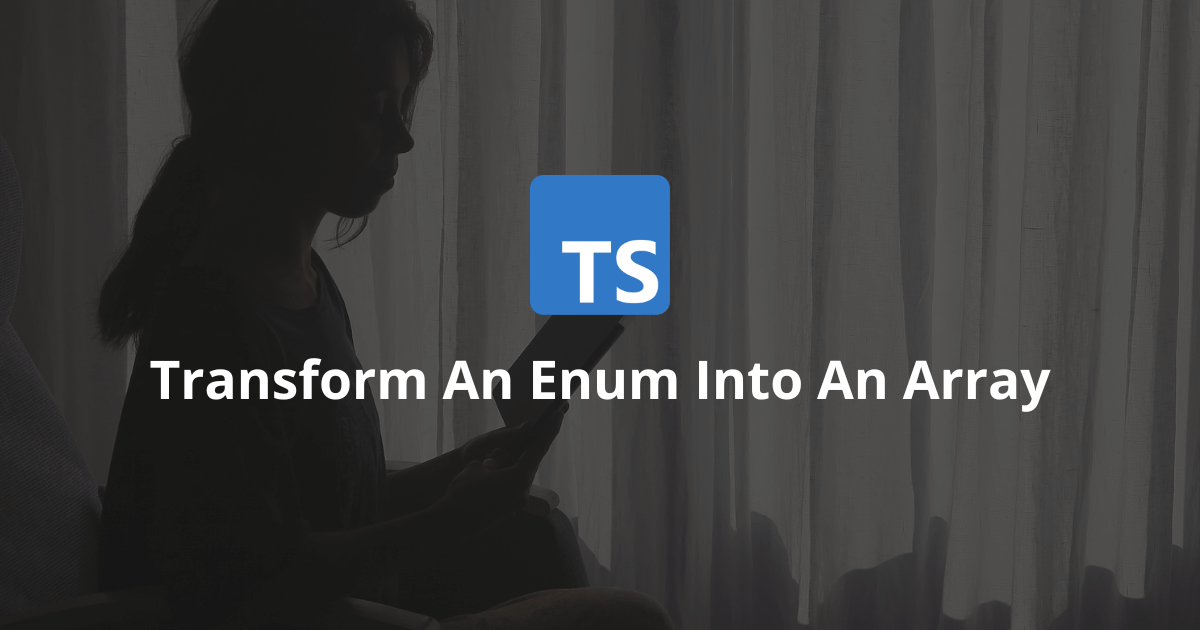
Enums in TypeScript, unlike interfaces, exist at runtime. They are converted into real objects by the TypeScript compiler.
How to transform a numeric enum into an array?
To transform a numeric enum into an array, you need to use the Object.values function with the filter function.
Let's have a small example. We have this enum:
typescriptenum Direction {
Up,
Down,
Left,
Right
}
We need to use the Object.values function to transform this enum into an array.
However, when we try to use the Object.values function on this enum, we get this result:
typescript// Outputs: ["Up", "Down", "Left", "Right", 0, 1, 2, 3]
console.log(Object.values(Direction));
As you can see, this is not what we want. But why is it happening?
For numeric enums, the Object.values function returns the values AND the keys of the enum (because that's how the TypeScript compiler compiles numeric enums into objects).
Note: With numeric enums, their value starts at zero and auto-increment (unless we provide default values).
To transform the KEYS of this enum into an array, we need to filter out the numeric values, like so:
typescript// Outputs: ["Up", "Down", "Left", "Right"]
console.log(
Object.values(Direction).filter((v) => isNaN(Number(v)))
);
To transform the VALUES of this enum into an array, we need to filter out the string values, like so:
typescript// Outputs: [0, 1, 2, 3]
console.log(
Object.values(Direction).filter((v) => !isNaN(Number(v)))
);
And here is the complete solution:
typescriptenum Direction {
Up,
Down,
Left,
Right
}
// Outputs: ["Up", "Down", "Left", "Right"]
console.log(
Object.values(Direction).filter((v) => isNaN(Number(v)))
);
// Outputs: [0, 1, 2, 3]
console.log(
Object.values(Direction).filter((v) => !isNaN(Number(v)))
);
How to transform a string enum into an array?
To transform a string enum into an array, you can use the Object.values or Object.keys functions.
Let's have a small example. We have this enum:
typescriptenum Country {
US = 'United States',
CA = 'Canada',
FR = 'France'
}
To transform the KEYS of this enum into an array, you can do:
typescript// Outputs: ["US", "CA", "FR"]
console.log(Object.keys(Country));
To transform the VALUES of this enum into an array, you can do:
typescript// Outputs: ["United States", "Canada", "France"]
console.log(Object.values(Country));
And here is the complete solution:
typescriptenum Country {
US = 'United States',
CA = 'Canada',
FR = 'France'
}
// Outputs: ["US", "CA", "FR"]
console.log(Object.keys(Country));
// Outputs: ["United States", "Canada", "France"]
console.log(Object.values(Country));
Final thoughts
As you can see, transforming an enum into an array is simple in TypeScript.
For numeric enums, combine the Object.values function with the filter function.
Use the Object.values or the Object.keys functions for string enums.
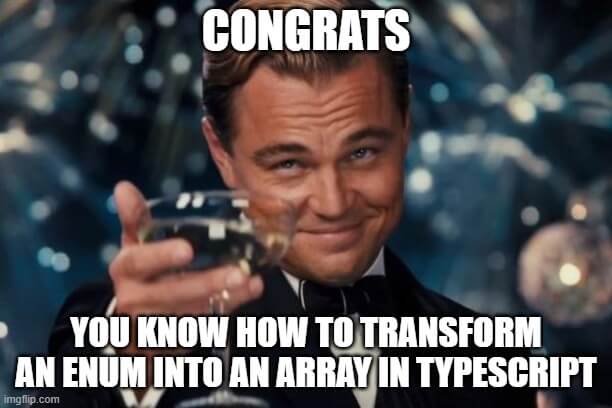
Here are some other TypeScript tutorials for you to enjoy: