How To Get The Last Element Of An Array In TypeScript?
In TypeScript, arrays are one of the most useful data structures. They can store a collection of values of any data type. Sometimes, a developer might need to get the last element of an array. Luckily, it is easy to do.
To get the last element of an array in TypeScript, you can:
- Use the Array's length property.
- Use the slice function.
- Use the at function.
- Use the pop function.
This article explains those methods and shows code examples for each.
Let's get to it 😎.
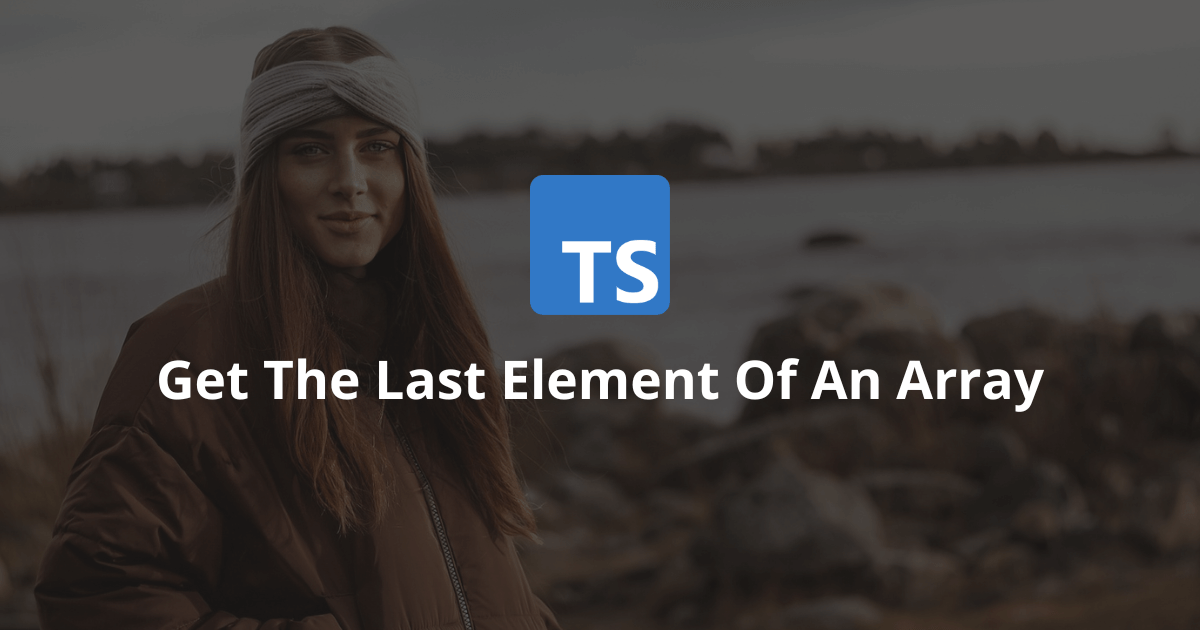
In TypeScript, an array's index always begins at 0.
It means that the last element's index is: array.length -1.
Method #1 - Use the Array's length property
The easiest way to get the last element of an array is to use the built-in length property.
Here is how to use it:
typescriptconst arr = [ 'Tim', 'Bob', 'Math' ];
// Outputs: 'Math'
console.log(arr[arr.length - 1]);
In this case, the array's length is three.
The last element's index is two.
That's why we need to subtract one from the array's length to get the last element.
Method #2 - Use the slice function
Another option to get the last element of an array is to use the slice function.
The slice function returns selected array elements in a new array.
Here is how to use it:
typescriptconst arr = [ 'Tim', 'Bob', 'Math' ];
// Outputs: 'Math'
console.log(arr.slice(-1)[0]);
Note: When using slice, a negative integer selects x elements from the end of the array.
Method #3 - Use the at function
Another option to get the last element of the array is to use the at function.
This method is similar to the bracket notation.
Here is how to use it:
typescriptconst arr = [ 'Tim', 'Bob', 'Math' ];
// Outputs: 'Math'
console.log(arr.at(-1));
Note: This method only works when the lib property in the typescript config is set to es2022.
Method #4 - Use the pop function
The pop method is helpful when you need to get and remove the last element of a TypeScript array.
Here is an example:
typescriptconst arr = [ 'Tim', 'Bob', 'Math' ];
// Outputs: 'Math'
console.log(arr.pop());
Note: This solution affects the array's values and length.
Final thoughts
As you can see, you have many different options to get the last element of an array in TypeScript.
You can pick any one of those methods based on your preferences.
As for me, I mostly stick to good old fashion bracket notation.
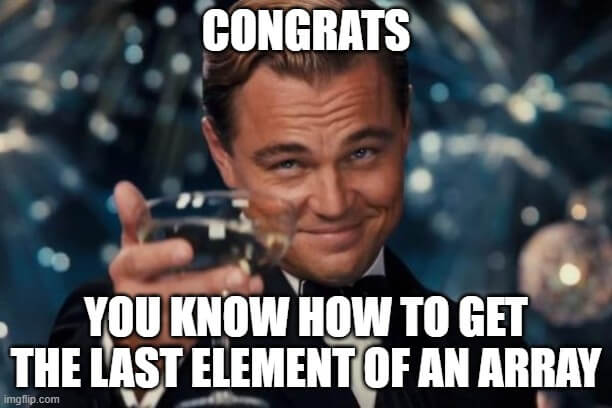
Here are some other TypeScript tutorials for you to enjoy: