How Does An Array Work In TypeScript?
An array is one of the most frequently used foundational data structures in programming. But what is it exactly, and how does it work in TypeScript?
In TypeScript, an array is a structure that stores values in sequential order.
Here is a basic example of an array of strings in TypeScript:
typescriptconst names = ['Tim', 'Bob', 'Jack'];
This article explains the array data type and shows many code examples.
Let's get to it 😎.
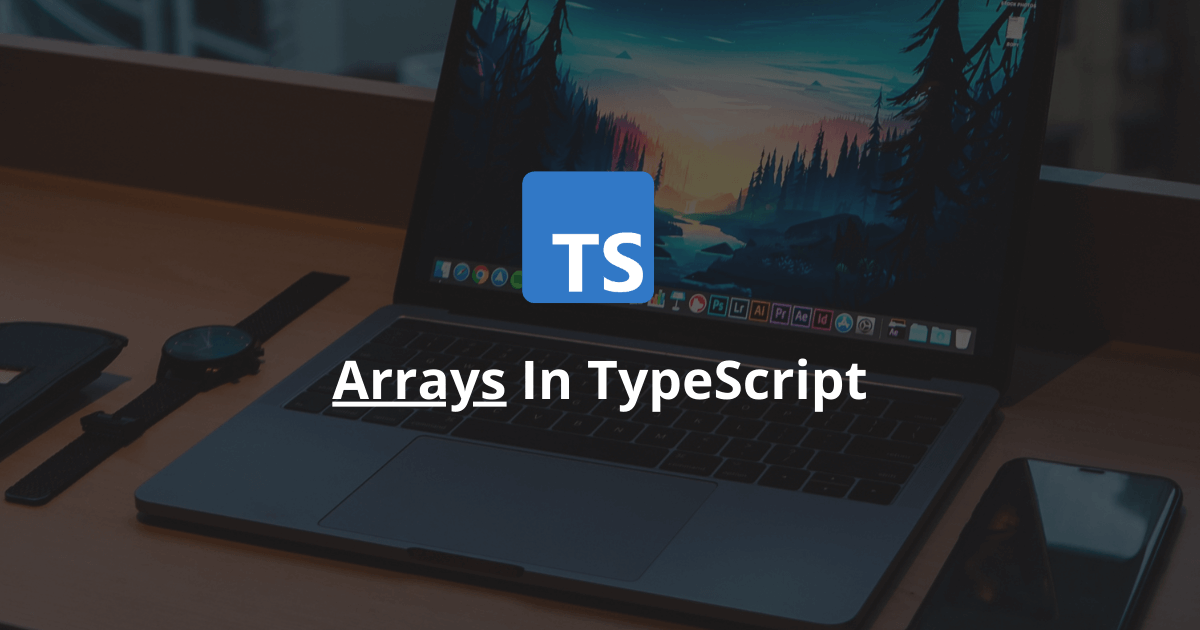
The definition
An array is an ordered list of values. Those values can be of any data type.
You can use an array when you have a list of values and want to work with them as a collection.
Here is a small example of an array of numbers:
typescriptconst ages = [22, 32, 19];
When we don't add a type to the array, TypeScript infers it. But we can also add it to make the code more verbose:
typescriptconst ages: number[] = [22, 32, 19];
The TypeScript compiler will check the data type of the array's elements and prevent the developer from adding an element of an unallowed type.
typescriptconst ages = [22, 32, 19 ];
// This doesn't work.
ages.push('Tim');
Here we get an error because the array is of type number[], but we are trying to add a string element.
Note: You can add multiple types of elements to an array by setting the array type to a union.
Note: An array can also be readonly by using the readonly keyword or by using as const.
Initialization
In TypeScript, you have two different methods of initializing an array.
The first one uses square brackets. Here is an example:
typescriptconst animals: string[] = ['cow', 'dog', 'cat'];
The second one uses the generic Array type. Here is an example:
typescriptconst animals: Array<string> = ['cow', 'dog', 'cat'];
Which of those methods you choose is a question of preference because they both produce the same result.
You can also initialize an empty array and add values later.
Here is an example:
typescriptconst animals: Array<string> = [];
animals.push('cow');
animals.push('dog');
animals.push('cat');
Note: You can also initialize an array without writing its type. In that case, TypeScript infers it.
Accessing elements
You can access an array's element using the index notation.
Here is an example:
typescriptconst animals: string[] = ['cow', 'dog', 'cat'];
// Outputs: 'cow'
console.log(animals[0]);
// Outputs: 'dog'
console.log(animals[1]);
// Outputs: 'cat'
console.log(animals[2]);
Note: The index of an array always starts at zero.
You can also destructure the array to get an element at a specific position.
Here is an example:
typescriptconst animals: string[] = ['cow', 'dog', 'cat'];
const [cow, dog, cat] = animals;
// Outputs: 'cow'
console.log(cow);
// Outputs: 'dog'
console.log(dog);
// Outputs: 'cat'
console.log(cat);
Note: Destructuring is useful when you don't have a lot of elements inside the array. For example, destructuring is often used with the useState React hook.
The methods
The array prototype provides many built-in methods.
Here are a few of them:
One of the most useful functions is the push method to add a new element to an array. To find the index of an element, use the indexOf function. Sorting the array is done using the sort function.
typescriptconst animals: string[] = ['cow', 'dog', 'cat'];
// ["cow", "dog", "cat", "elephant"]
animals.push('elephant');
// ["cat", "cow", "dog", "elephant"]
animals.sort();
// Outputs: 2
console.log(animals.indexOf('dog'));
Dictionary
Here is a table with all the built-in array methods:
Name | Description |
---|---|
concat | Merges two or more arrays. |
copyWithin | Copies part of an array to a location in the same array. |
every | Tests if all the elements of an array pass a condition. |
filter | Creates a copy of the array with only the filtered items. |
flat | Creates a new array with all the sub-arrays flattened. |
flatMap | Applies a callback to every item of an array and flattens the result. |
forEach | Runs a callback on every item of the array. |
indexOf | Returns the index of an element or -1 if the element doesn't exist. |
lastIndexOf | Returns the last index of an element or -1 if the element doesn't exist. |
map | Runs a callback on every item and creates a new array populated by each callback's result. |
reduce | Iterates over an array and executes a reducer on each array element. |
reverse | Reverses an array. |
slice | Returns a shallow copy of a portion of an array. |
some | Tests if one of the elements of an array passes a condition. |
sort | Sorts an array. |
splice | Removes or replaces existing elements of an array. |
entries | Returns a new iterator of the array. |
fill | Fills the array with a static value. |
find | Returns the first element that matches a condition. |
findIndex | Returns the index of the first element that matches a condition. |
findLast | Returns the last element that matches a condition. |
findLastIndex | Returns the index of the last element that matches a condition. |
includes | Determines if an array includes an item. |
join | Creates a string from the array's elements. A separator separates the elements. |
keys | Returns an iterator containing the indexes of an array. |
toLocaleString | Returns a string that represents the array. |
values | Returns an iterator containing the values of an array. |
How to traverse an array?
To traverse an array, you can use the for loop.
Here is an example:
typescriptconst animals: string[] = ['cow', 'dog', 'cat'];
for (const i in animals) {
console.log(animals[i]);
}
// Outputs: 'cow'
// Outputs: 'dog'
// Outputs: 'cat'
Another option to traverse an array involves using the built-in forEach function.
Here is an example:
typescriptconst animals: string[] = ['cow', 'dog', 'cat'];
animals.forEach(animal => {
console.log(animal);
});
// Outputs: 'cow'
// Outputs: 'dog'
// Outputs: 'cat'
Final thoughts
As you can see, arrays are easy to learn but very powerful in TypeScript.
I always recommend setting the type of the array explicitly. This practice helps avoid bugs and refactoring problems.
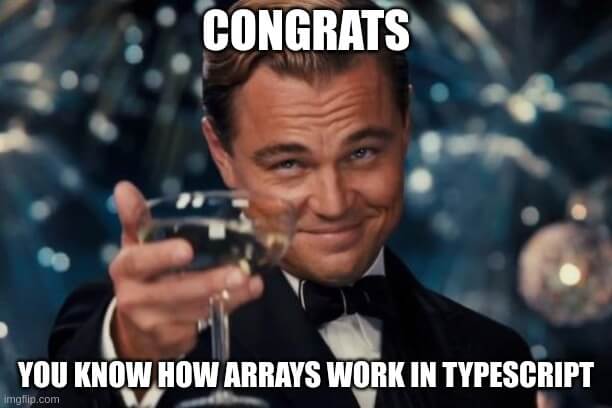
Here are some other TypeScript tutorials for you to enjoy: