How To Define An Array Of Objects In TypeScript?
Often, a TypeScript developer needs to define an array of objects. Luckily, this is easy to achieve.
In TypeScript, to define an array of objects, you can:
- Use an existing type or interface.
- Use an inline type.
- Use the built-in Array type.
- Use the typeof operator.
This article will go through those methods and show how to implement them with code examples.
Let's get to it 😎.
To define an array of objects in TypeScript, a developer must specify the object's type AND pass it to the array. That way, the array will only be able to hold objects of that specific type.
Solution #1 - Use an existing type or interface
The easiest way to define an array of objects is to define a type or interface that matches the desired structure of one object of the array. Then, you can set the type of the array that holds the objects to array of the created type (or interface).
Let's see how it works with an example.
typescriptinterface IPerson {
name: string;
age: number;
}
const people: IPerson[] = [
{
age: 27,
name: 'Tim'
},
{
age: 28,
name: 'Bob'
}
]
As you can see, we create the IPerson interface, which we use to define the array's type.
Solution #2 - Use an inline type
If you don't plan to re-use the object's type that the array holds, you can define an inline type.
Let's see how it works with an example.
typescriptconst people: { name: string; age: number; }[] = [
{
age: 27,
name: 'Tim'
},
{
age: 28,
name: 'Bob'
}
]
However, this method can become hard to manage if the object's type holds a lot of different properties.
Solution #3 - Use the built-in Array type
Another way to define an array of objects in TypeScript is by using the special built-in Array<T> type.
The Array<T> type accepts a generic argument that indicates the structure of the object that the array holds.
Let's see how it works with an example.
typescriptconst people: Array<{ name: string; age: number; }> = [
{
age: 27,
name: 'Tim'
},
{
age: 28,
name: 'Bob'
}
]
Some developers prefer to use the Array<T> type instead of [] because it is more verbose.
Solution #4 - Use the typeof operator
A different way to define an array of objects involves using the TypeScript typeof operator.
Let's see how it works with an example.
typescriptconst item1 = {
age: 27,
name: 'Tim'
};
const item2 = {
age: 28,
name: 'Bob'
};
const people: Array<typeof item2> = [
item1,
item2
];
Using the typeof operator, we can determine the object's structure and pass it to the Array<T> type.
However, you must have one object constant ready before the array's initialization.
Final thoughts
As you can see, it is easy to create an array of objects in TypeScript.
We have four different ways of achieving it.
Which one you choose is a question of preference.
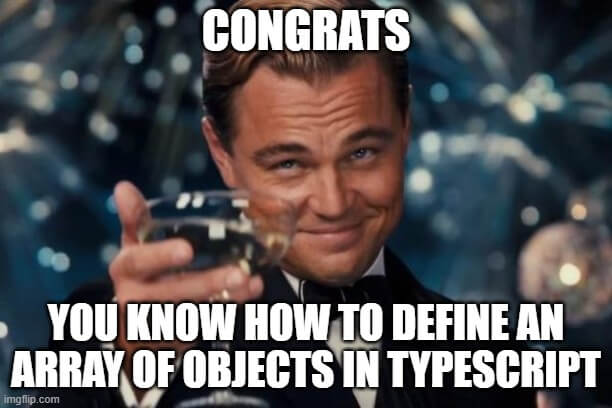
Here are some other TypeScript tutorials for you to enjoy: