How To Extend An Interface In TypeScript?
When working on a TypeScript project, a developer sometimes needs to modify an existing interface by adding one or more members. Luckily, this is easy to do.
To extend an interface in TypeScript, you must use the extends keyword.
This article explains using the extends keyword in TypeScript and shows many code examples.
Let's get to it 😎.
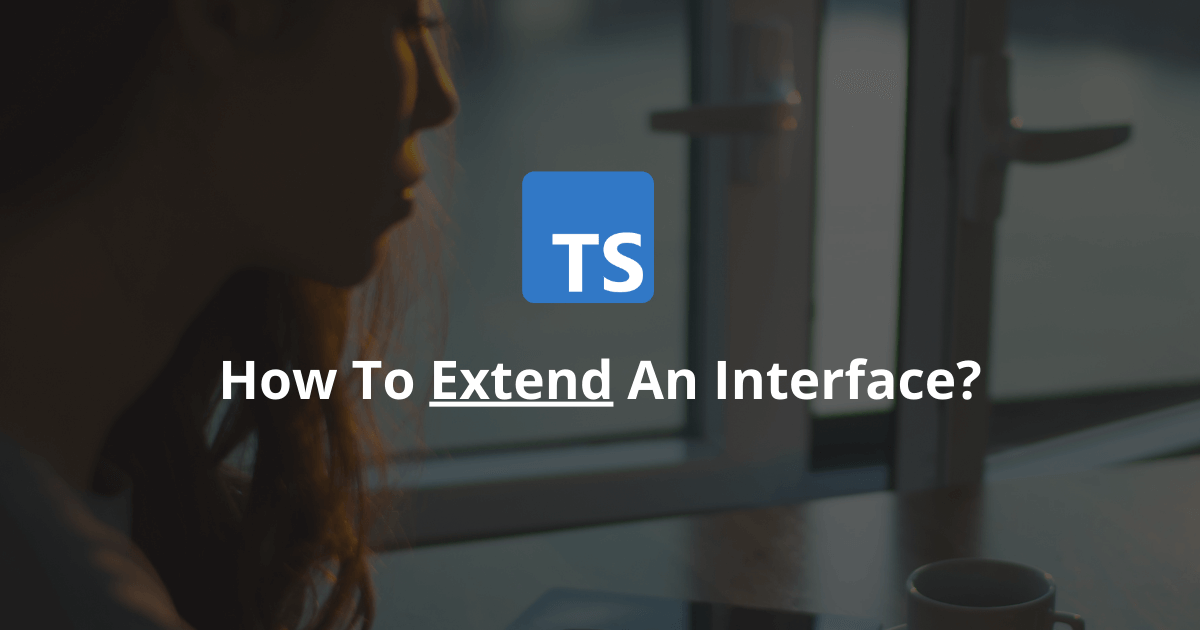
How to make an interface extend another interface?
To extend an interface, a developer must use the extends keyword.
Let's see how it works with an example.
We have an interface containing two methods, AND we want to add another function WITHOUT modifying the original one.
typescriptinterface IAnimal {
eat: (food: string) => void;
sleep: () => void;
}
interface IFish extends IAnimal {
swim: () => void;
}
const obj: IFish = {
eat: (food: string): void => {
console.log('Eating ' + food);
},
sleep: (): void => {
console.log('Sleeping');
},
swim: (): void => {
console.log('Swimming');
}
}
We first define the IAnimal interface, which contains two methods.
Then we define the IFish interface, which extends the IAnimal interface and adds a new method.
The IFish interface contains the eat, sleep, and swim functions.
Note: If you are interested in extending a type, I wrote an extensive guide.
How to extend multiple interfaces?
In TypeScript, an interface can extend multiple interfaces.
Use the extends keyword and pass a list of comma-separated interfaces.
Let's see how it works with an example.
typescriptinterface IAnimal {
eat: (food: string) => void;
sleep: () => void;
}
interface IFish {
swim: () => void;
}
interface IJellyFish extends IAnimal, IFish {
glow: () => void;
}
const obj: IJellyFish = {
eat: (food: string): void => {
console.log('Eating ' + food);
},
glow: (): void => {
console.log('Glowing');
},
sleep: (): void => {
console.log('Sleeping');
},
swim: (): void => {
console.log('Swimming');
}
};
In this example, the IJellyFish interface extends the IAnimal and the IFish interfaces.
How to make an interface extend a class?
TypeScript gives developers the ability to make an interface extend a class.
The interface inherits the methods and properties from the class it extends.
Protected and private members are also inherited, making the interface only implementable by a class containing those protected and private members.
Let's see how it works with an example.
typescriptclass Animal {
public name: string = '';
}
interface IFish extends Animal {
swim: () => void;
}
const fish: IFish = {
name: 'Fish',
swim: (): void => {
console.log('swim');
}
};
In this example, the IFish interface inherits the name property from the Animal class.
How to override a property's type when extending an interface?
To override a property's type when extending an interface, you can use the omit utility type.
Let's see how to do it with an example.
typescriptinterface IOriginal {
name: string
}
interface IFinal extends Omit<IOriginal, 'name'> {
name: number
}
const obj: IFinal = {
name: 222
};
In this example, we override the name property's type from string to number.
Final thoughts
As you can see, extending an interface is simple in TypeScript.
Simply use the extends keyword, and voila!
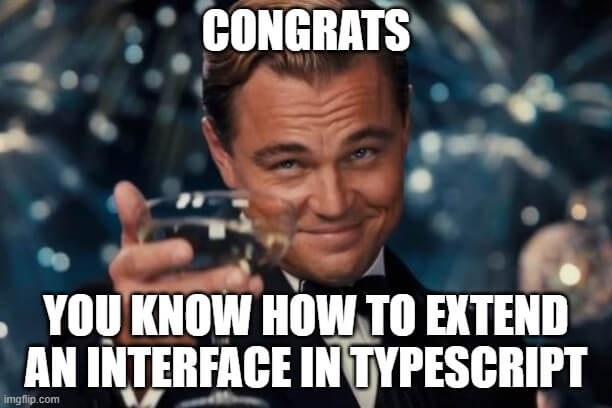
Here are some other TypeScript tutorials for you to enjoy: