How To Extend A Type In TypeScript?
In TypeScript, developers often need to combine or extend a type to create a new one. Luckily, this is simple to do and is very easy to accomplish.
This article will explore different ways of extending a type or an interface in TypeScript and also answer common questions.
Let's get to it 😎.
To extend a type in TypeScript, a developer has multiple options:
- Use the intersection type.
- Use the extends keyword.
How to extend a type using the intersection type?
In TypeScript, the intersection type helps combine existing object types (types and interfaces).
The intersection type is defined by the & operator.
Here are a few examples of how to extend a type using the intersection operator:
1. Extend a type with a type.
typescripttype ArticleContent = {
content: string;
}
type Article = ArticleContent & {
category: string;
}
2. Extend a type with an interface.
typescriptinterface ArticleContent {
content: string;
}
type Article = ArticleContent & {
category: string;
}
I wrote an extensive article on the difference between type and interface in TypeScript.
How to extend a type using the extends keyword?
Another way to extend a type in TypeScript is to use the special extends keyword.
However, you should note that the extends keyword only works with interfaces.
Here are a few examples of how to use the extends keyword.
1. Extend an interface with an interface.
typescriptinterface ArticleContent {
content: string;
}
interface ArticleCategory extends ArticleContent{
category: string;
}
2. Extend an interface with a type.
typescripttype ArticleContent = {
content: string;
}
interface ArticleCategory extends ArticleContent{
category: string;
}
Another option to merge interfaces is to use declaration merging.
How to extend multiple interfaces?
You can also extend multiple interfaces by using the extends keyword and listing all the interfaces separated by commas.
Here is an example of this:
typescriptinterface ArticleContent {
content: string;
}
interface ArticleAuthor {
author: string;
}
interface ArticleCategory extends ArticleContent, ArticleAuthor {
category: string;
}
How to extend a class with an interface?
Many developers are unaware, but TypeScript allows an interface to extend a class.
Here is an example of how to do it:
typescriptclass Article {
private content: boolean;
}
interface MyArticle extends Article {
getContent(): void
}
Final thoughts
In conclusion, extending a type or an interface in TypeScript is very simple.
You have two ways of doing it: using the intersection operator or the extends keyword.
Finally, to learn more about how to modify properties of existing types, I recommend reading my article on TypeScript utility types.
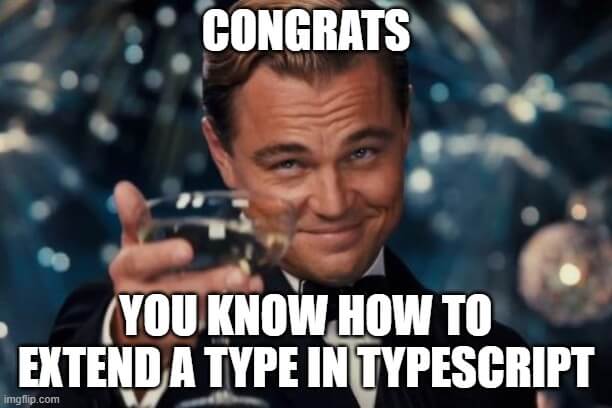