What Is An Intersection Type In Typescript?
TypeScript provides developers with different ways to combine and compose existing types to create new ones. One such TypeScript feature is the intersection type.
The intersection type allows a developer to combine multiple types into one.
This article will explain everything about intersection types in TypeScript and also answer some of the most common questions.
Let's get to it 😎.
The definition
In TypeScript, an intersection type combines multiple types (two or more) into one.
The new intersection type contains all the properties from all the combined types.
The developer needs to use the & symbol to combine all the types.
Let's see an example to understand the intersection type better:
typescriptinterface IArticle {
content: string;
}
interface ICategory {
category: string;
}
type ArticleWithCategory = IArticle & ICategory;
const article: ArticleWithCategory = {
content: 'my content',
category: 'web'
};
In this example, we create a new type called ArticleWithCategory from an intersection of two types.
The new type ArticleWithCategory has all the properties from IArticle and ICategory.
If we try to create a new variable of type ArticleWithCategory without filling all the properties, the TypeScript compiler will output an error.
typescript// TypeScript Compiler error:
// Type '{ content: string; }' is not assignable to type 'ArticleWithCategory'.
// Property 'category' is missing in type '{ content: string; }' but required in type 'ICategory'.
const article: ArticleWithCategory = {
content: 'my content',
};
Note: The order of the intersection between types doesn't matter. It will result in the same intersection type.
What is the difference between an intersection type vs extends?
Both the intersection type and extends are used to combine TypeScript object types.
That's why many developers confuse them.
- To combine interfaces, we use extends or an intersection type.
- To combine types, we use intersection types.
Read: The differences between an interface and a type.
The most significant difference between an intersection type and extends is their behavior when a property is present in both types, but the type of that property is not the same.
1. When extending interfaces, having the same property of different types outputs an error.
typescriptinterface IArticle {
category: string;
}
// TypeScript compiler Error
// Interface 'IArticleWithCategory' incorrectly extends interface 'IArticle'.
// Types of property 'category' are incompatible.
// Type 'string[]' is not assignable to type 'string'.(2430)
interface IArticleWithCategory extends IArticle {
category: string[];
}
2. When intersecting types, having the same property of different types works.
typescripttype IArticle = {
category: string;
}
type IArticleWithCategory = IArticle & {
category: string[];
}
Note: I wrote an extensive guide on how to extend a type in TypeScript on this blog.
Final thoughts
In conclusion, an intersection type is a combination of two or more types that contains all the properties from all of the combined types.
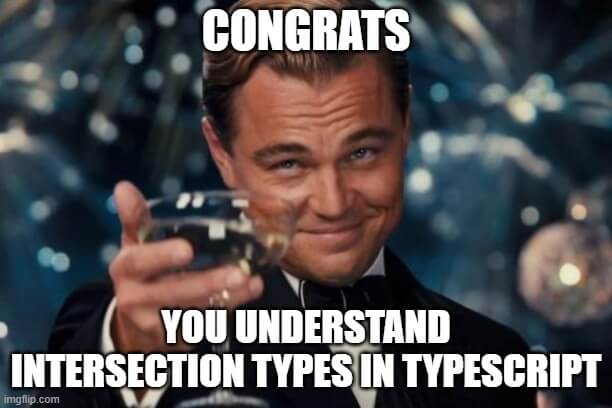
Finally, to learn more about TypeScript and support my blog, here is another article for you to enjoy: