Objects in TypeScript - The Definitive Guide
In TypeScript, just like in JavaScript, an object contains a set of keys and values. Objects are used to group and pass data to functions. In TypeScript, we represent those objects, with object types, usually interfaces or types.
Here is an example of an object with its corresponding TypeScript object type definition.
typescriptinterface IArticle {
date: number;
content: string;
}
const article: IArticle = {
content: 'new content',
date: Date.now(),
};
In this article, I will go over, in detail, objects, object types, answer common TypeScript questions, as well as provide real-life TypeScript examples for better understanding.
Let's get to it 🥸.
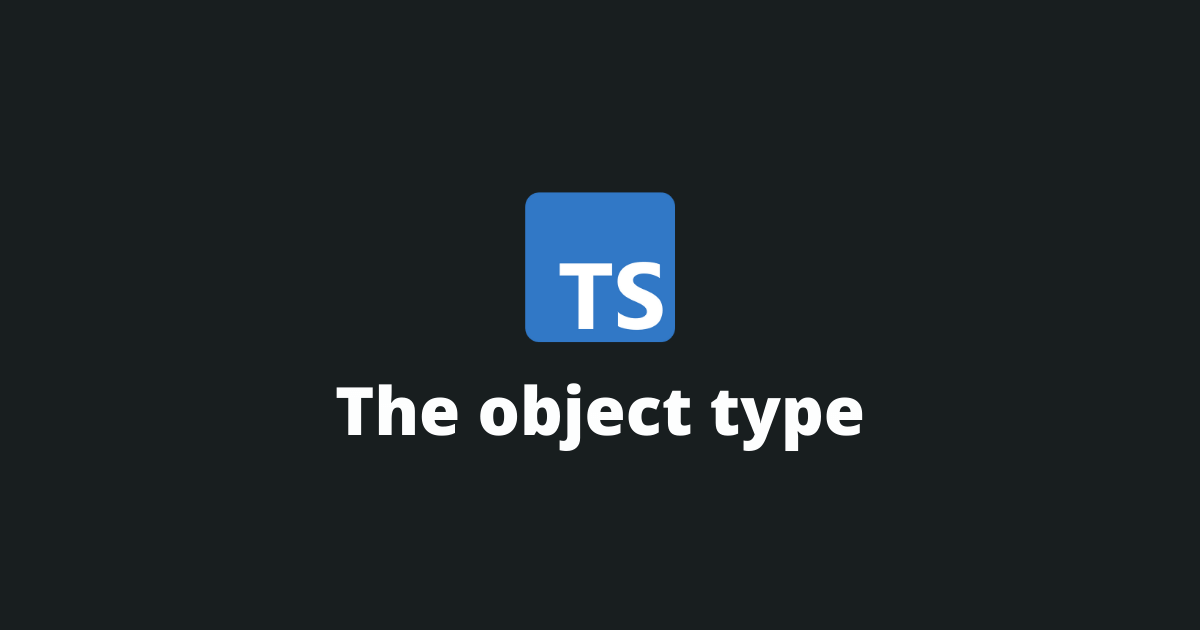
The definition
In JavaScript, an object is a complex data type. It contains a set of key/value pairs.
The value of each property of an object can be either:
Using the object literal notation, we can declare an object like so:
typescriptconst book = {
publicationDate: Date.now(),
title: 'Odysee'
};
We can then use the book object in our code, for example, to pass it as a parameter to a function.
JavaScript has 7 primitive types (number, bigint, string, boolean, null, undefined, and symbol).
In TypeScript, objects are represented with object types. An object type can be either anonymous or named (with an interface or a type).
Here is an example of an anonymous object type:
typescriptconst getDate = (book: { date: number, title: string }): number => {
return book.date;
};
Here is the same example, but with a named object type, in this case, an interface:
typescriptinterface IBook {
date: number,
title: string
}
const getDate = (book: IBook): number => {
return book.date;
};
And the same example one last time, but with a type:
typescripttype Book = {
date: number,
title: string
}
const getDate = (book: Book): number => {
return book.date;
};
As you can see, when you need to re-use an object type multiple times, it is better to create a named object type.
If you don't know which one to choose between a type vs an interface, I have written a guide on it.
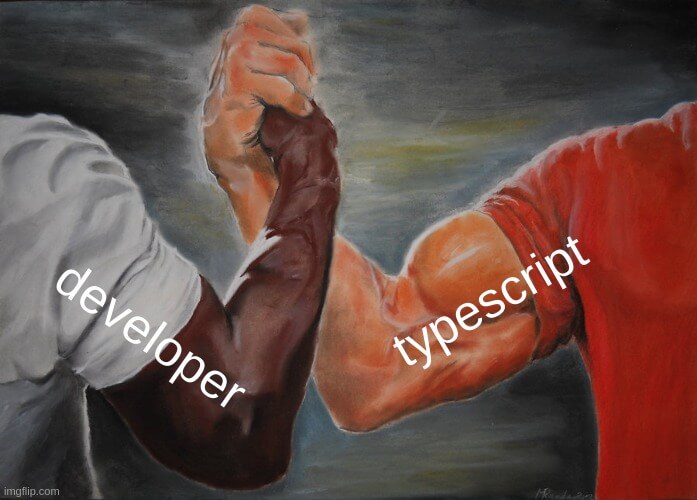
In TypeScript, a developer can specify 3 things about an object type property:
- The type of the property.
- Whether the property is optional.
- Whether the property is read-only.
Optional properties
You can make a property optional by using the question mark (?).
Here is an example of this:
typescriptinterface IBook {
date?: number;
title: string;
}
In this example, the date field is optional.
Read-only properties
You can mark a property as read-only by using the special readonly TypeScript keyword.
Here is an example of this:
typescriptinterface IBook {
date: number;
readonly title: string;
}
const book: IBook = {
date: Date.now(),
title: 'Odysee'
};
// This will not work.
book.title = 'Tim';
Read more: The readonly keyword in TypeScript
In this example, we cannot reassign the title property, because it is read-only.
If you want to make all the properties of an interface read-only, use the ReadOnly utility type.
How to define an object with dynamic keys?
If you want to define an object with dynamic keys, you will need to use the index signature. It is very useful when you want to create a TypeScript dictionary.
Here is an example of an object using the index signature:
typescriptinterface IMap {
[key: string]: string;
}
const map: IMap = {
a: '1',
b: '2'
};
As you can see, we can add any property that we want inside the map object.
How to define a generic object type?
Generics allow developers to pass a type as an argument to a function or type. By using generics we are making components much more reusable.
Here is an example of a generic in TypeScript:
typescriptinterface IPerson<T> {
favoriteSystem: T;
}
interface IXbox {
title: string;
}
const person: IPerson<IXbox> = {
favoriteSystem: {
title: 'xbox'
}
};
In this example, we have created a generic interface that accepts different favorite systems.
What is the '{}' type in TypeScript?
In TypeScript, you can specify an empty object by using the empty type '{}' notation. An empty type describes an object that contains no properties.
Here is an example of an empty type:
typescriptconst obj: {} = {};
If you try to access an empty object's property you will get a TypeScript compiler error.
Object vs object
Since TypeScript is an extension of JavaScript, it also contains the uppercase Object type. It is very important to understand the difference between them because they are two completely different things.
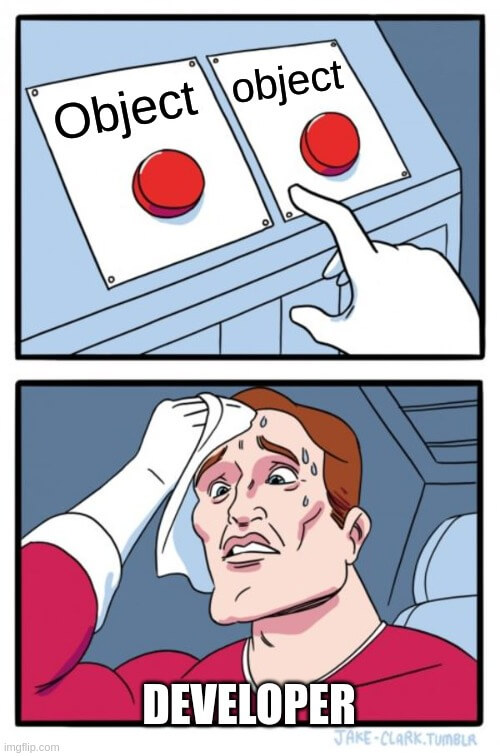
The uppercase Object is a class that stores key/value pairs and contains multiple helpers function like freeze, create, and many more.
The lowercase object is a type that represents key/value pairs in TypeScript.
How to check if an object is empty?
To check if an object is empty you need to use the Object.keys function.
typescriptconst isEmpty = (obj: any): boolean => {
return Object.keys(obj).length === 0;
};
In this helper method, we check that the object contains 0 keys.
Final thoughts
As you can see, objects are the foundation of JavaScript programming, just like the control flow statements (for loop, switch, etc...). So much, that developers use them practically every time they write a new line of code.
Thus it is very important to understand them well, before learning more complex things.
On the other hand, object types are representations of those objects in TypeScript.
If you have any questions about objects or object types, don't hesitate to ask them in the comments, I always respond.
Thank you for reading this article, please share it with your fellow TypeScript developers.