How Do Optional Parameters Work In TypeScript?
As you may know, TypeScript is a strongly typed language. By default, when you declare a function with parameters, you need to pass values for each parameter when you call it. Sometimes, however, developers may want to make a function parameter non-required. Luckily, TypeScript offers optional parameters to fix this issue.
In TypeScript, to create a function with an optional parameter, you need to use a question mark after the parameter declaration, like so:
typescriptconst getFullName = (firstName: string, lastName?: string): string => {
if (!lastName) {
return firstName;
}
return `${firstName} ${lastName}`;
}
This article will go through everything about optional parameters in TypeScript and answer common questions.
Let's get to it 😎.
Optional parameters in TypeScript
In TypeScript, when you call a function with parameters, the compiler checks that you pass the correct number of arguments and the right argument types.
TypeScript considers all the parameters required unless specified otherwise.
To define an optional parameter, you must use a question mark (?) after a parameter name.
Here is an example of a function with an optional parameter:
typescriptconst getError = (message: string, error?: number): string => {
if (!error) {
return message;
}
return `${error}: ${message}`;
}
Note: Here we are using string interpolation to concat the strings.
In this example, the error parameter is optional.
You can also define multiple optional parameters, like so:
typescriptconst getAddress = (code: string, address?: string, city?: string): string => {
return `${code} | ${address || ''} | ${city || ''}`;
};
Note: Use default parameters if you want to provide a default value for a parameter.
Order of optional parameters
When you have optional parameters in a function, it is essential to follow the correct order.
Optional parameters should always be placed AFTER the required parameters.
Otherwise, the TypeScript compiler will complain.
How to check if a value was passed to an optional parameter?
The parameter is undefined when a developer doesn't pass a value to an optional parameter.
To check if an optional parameter has no value, simply check if the parameter's type is undefined, like so:
typescriptconst getAddress = (code: string, address?: string): string => {
if (typeof address === 'undefined') {
return code;
}
return `${code} | ${address}`;
};
In this example, we check that the address parameter has no value with an if statement.
Read more: The typeof operator in TypeScript
How to define an optional parameter in an interface?
In TypeScript, you can also define interface optional properties.
Here is an example of how to do it:
typescriptinterface IAddress {
city: string;
address: string;
street?: string;
}
In this example, the city and address fields are required, and the street property is optional.
Read more: How do interfaces work in TypeScript?
How to define an optional parameter in a constructor?
You can also define an optional parameter in a class constructor.
Here is an example of an optional parameter in a constructor:
typescriptclass Animal {
public constructor(
public name: string,
public age?: number
) {};
}
In this example, the age constructor parameter is optional.
Final thoughts
As you can see, setting up optional parameters in TypeScript is easy.
You can set an optional parameter in functions, interfaces, types, and constructors.
With functions, place all the optional parameters after the required ones.
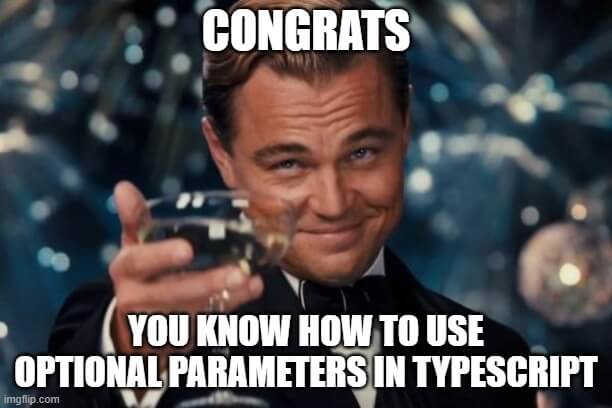
Here are other TypeScript tutorials for you to enjoy: