How To Set A Default Parameter In TypeScript?
Sometimes developers need to define a default parameter for a TypeScript function. Luckily, TypeScript, just like its counterpart JavaScript, offers a simple way to define default parameters.
In TypeScript, you can define a default parameter like so:
typescriptconst getUser = (firstName: string, role = 'user'): string => {
return `${firstName} | ${role}`;
}
This article will go through everything about default parameters in TypeScript and answer the most common questions.
Let's get to it 😎.
The definition
JavaScript supports default parameters since the ES2015 version.
TypeScript also fully supports default parameters.
Default parameters are optional parameters that fall back to a default value if it has no value or is undefined.
The difference between calling a function and omitting an optional parameter vs a default parameter is:
- If an optional parameter has no value, the parameter's value is undefined.
- If a default parameter has no value, the parameter's value is the default value.
Default parameters are set using the assignment operator (=).
Here is an example of a function with a default parameter:
typescriptconst outputUser = (firstName: string, lastName = 'Mousk'): void => {
console.log(`${firstName} ${lastName}`);
}
// Outputs: 'Tim Mousk'
outputUser('Tim');
// Outputs: 'Bob Clark'
outputUser('Bob', 'Clark');
In this example, the lastName parameter is an optional parameter with a default value.
When we call the outputUser function, we only need to pass an argument for the first parameter.
If the lastName parameter has no value or is undefined, it will fall back to its default value.
Note: Here we are using string interpolation to concat the strings.
Default parameters rules
Here are a few default parameters rules in TypeScript:
- In TypeScript, the type of a default parameter is inferred. You don't have to specify its type explicitly.
- Default parameters need to come AFTER the required parameters. They need to come last.
- Default parameters are optional.
- A default parameter takes the default value if you omit the argument or pass undefined to it.
- A developer can set multiple default parameters inside a function.
How to pass a function as the default value?
You can pass a function result as the default value to a default parameter.
Here is an example of how to do it:
typescriptconst outputUser = (name: string, year = new Date().getFullYear()): void => {
console.log(`${name} ${year}`);
}
// Outputs: 'Tim 2022'
outputUser('Tim');
In this example, the year parameter is an optional parameter that fallbacks to the current year if it has no value.
How to pass default values through destructuring?
TypeScript also supports default values when destructuring an object inside a function definition.
Here is an example of how to do it:
typescriptinterface IUser {
name: string;
year?: number;
}
const outputUser = ({ name, year = new Date().getFullYear() }: IUser): void => {
console.log(`${name} ${year}`);
}
// Outputs: 'Tim 2022'
outputUser({ name: 'Tim' });
Read more: How do interfaces work in TypeScript?
In this example, we first destruct the user object.
Then, we define a default value for the year property.
Final thoughts
As you can see, setting a default parameter in TypeScript is easy.
To define one yourself, use the assignment operator and provide a default value to it.
Be sure always to place the default parameters AFTER the required ones.
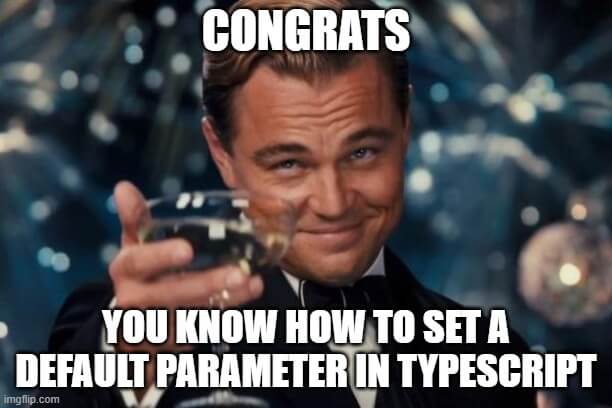
Here are some other TypeScript tutorials for you to enjoy: