How To Declare Class Constants In TypeScript?
Sometimes, developers need to declare class constants accessible by all the class's methods. Luckily, this is very easy to accomplish in TypeScript.
To declare class constants in TypeScript, you can use the readonly keyword, like so:
typescriptclass Cat {
readonly PROPERTY = 1;
}
This article will go through multiple ways of declaring class constants in TypeScript and answer some of the most common questions.
Let's get to it 😎.
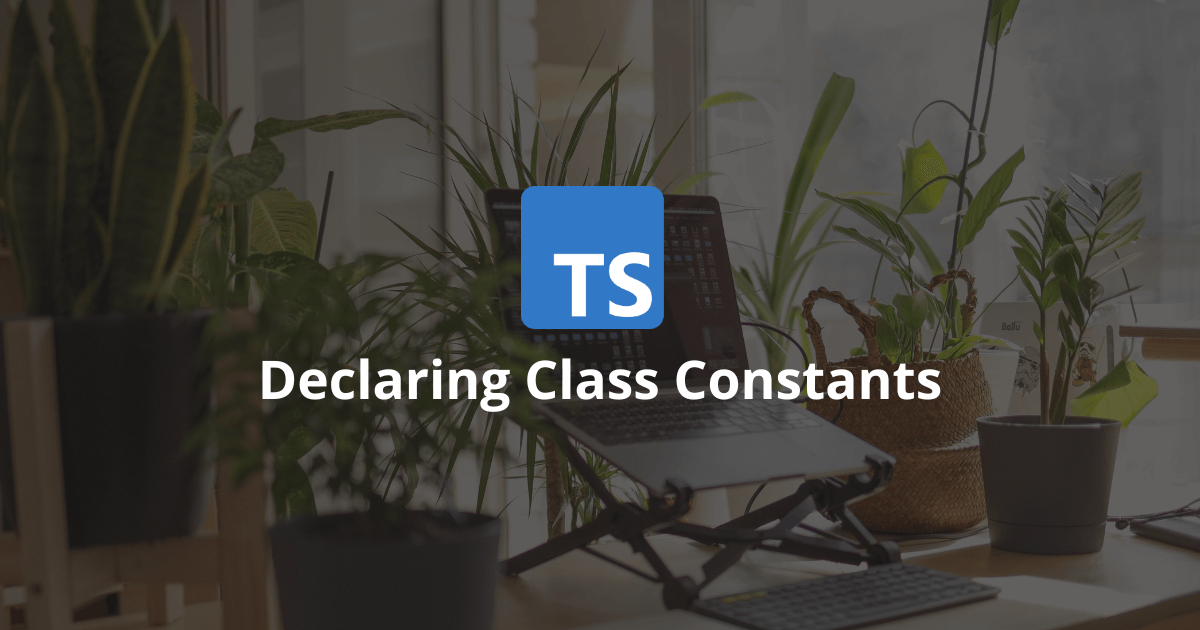
The readonly keyword
The readonly keyword marks a property as immutable.
This means that once set, the property's value cannot change.
You can assign a value to a read-only property:
- in the property declaration
- in the class constructor
You can use the readonly keyword in a class or an interface.
Read more: The readonly keyword in TypeScript
Declare a class constant with the readonly keyword
In TypeScript, you can declare a class constant using the readonly keyword.
Here is an example of this:
typescriptclass Animal {
readonly TYPE = 1;
}
// Outputs: 1
console.log(new Animal().TYPE);
In this example, the Animal class contains a class constant called TYPE.
To get the class constant outside the class, you must first initialize the class.
By default, this class constant is public.
If you want to make it only accessible inside the class, use the private keyword.
Declare a class constant with a static read-only property
Another way to declare a class constant is to combine the readonly and static keywords.
Here is an example of this:
typescriptclass Animal {
static readonly TYPE = 1;
}
// Outputs: 1
console.log(Animal.TYPE);
As you can see, you don't have to initialize the class to get the constant.
Also, only one single instance of the class constant exists.
Declare a class constant with a const
Also, you can declare a constant outside the class and use it inside the class.
Here is an example of this:
typescriptconst TYPE = 1;
class Animal {
public getType() {
return TYPE;
}
}
// Outputs: 1
console.log(new Animal().getType());
In this example, we declare the TYPE constant outside the class and use it in the getType function.
Final thoughts
As you can see, declaring class constants in TypeScript is simple.
Just use the readonly keyword before declaring the class property.
If you want the constant to only be accessible inside the class, declare it privately.
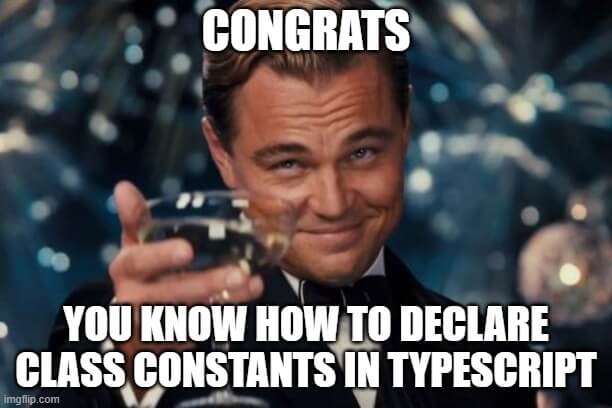
Here are some other TypeScript tutorials for you to enjoy: