How To Create A Readonly Property In TypeScript?
To create a read-only property in TypeScript, you need to use the readonly keyword.
Here is an example of a read-only property inside an interface:
typescriptinterface IPerson {
readonly name: string;
role: string;
}
This article explains everything about the readonly keyword and shows many code examples.
Let's get to it 😎.
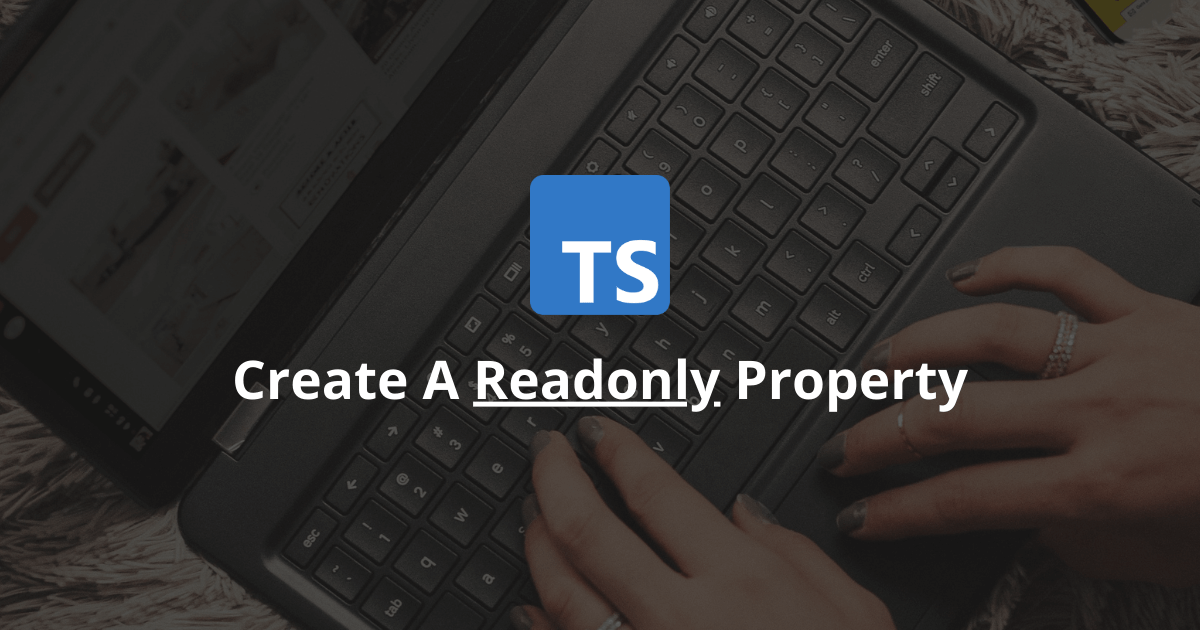
The definition
A developer can use the readonly keyword to mark a property as read-only to prevent re-assignment.
In TypeScript, you can use the readonly keyword with the following objects:
You cannot change the value of a read-only property once a value is assigned to it.
You can assign a value to a read-only property only in those two places:
- In the property declaration.
- In the class constructor (only for classes).
Note: If you want to create a read-only array, cast your array as a const.
How to mark a property as read-only in a class?
In TypeScript, the readonly keyword works with class properties.
Here is an example:
typescriptclass Person {
public readonly name: string;
public constructor (name: string) {
this.name = name;
}
}
const person = new Person('Tim');
// Outputs: 'Tim'
console.log(person.name);
If we try to assign a different value to the name property, we will get an error:
typescript// Error: Cannot assign to 'name' because it is a read-only property.
person.name = 'Bob';
You can also declare class constants with the readonly keyword. A class constant's value is assigned in the property declaration AND cannot be changed.
typescriptclass Cat {
public readonly PROPERTY = 1;
}
const cat = new Cat();
// Outputs: 1
console.log(cat.PROPERTY);
How to mark a property as read-only in an interface?
To mark a property as read-only in an interface, you can use the readonly keyword.
Here is an example:
typescriptinterface IPerson {
readonly name: string;
role: string;
}
const person: IPerson = {
name: 'Tim',
role: 'admin'
};
If we try to re-assign both properties, we will get an error for the name property:
typescript// This works.
person.role = 'user';
// Error: 'Cannot assign to 'name' because it is a read-only property.(2540)'
person.name = 'Bob';
Note: You can use the Readonly utility type to make all the properties read-only.
How to mark a property as read-only in a type?
The readonly keyword also works with types.
Here is the same example, but this time with a type instead of an interface:
typescripttype Person = {
readonly name: string;
role: string;
}
const person: Person = {
name: 'Tim',
role: 'admin'
};
// This works.
person.role = 'user';
// Error: 'Cannot assign to 'name' because it is a read-only property.(2540)'
person.name = 'Bob';
readonly vs. const
Both the readonly and const keywords prevent re-assignment.
The difference between them is:
- The readonly keyword is for properties.
- The const keyword is for variables.
Final thoughts
As you can see, it is easy to mark a property as read-only in TypeScript.
Simply use the readonly keyword before the property name.
Remember that you can only assign a value to a read-only property inside the property declaration or the class constructor.
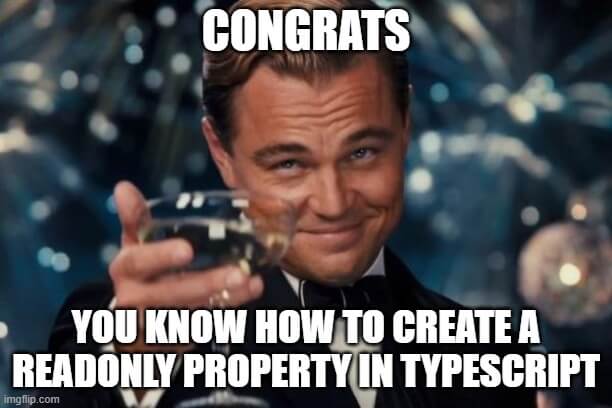
Here are some other TypeScript tutorials for you to enjoy: