How To Make A Class Implement An Interface In TypeScript?
When using object-oriented programming concepts in TypeScript, often a developer needs to make a class implement an interface. Luckily, this is easy to do.
To make a class implement an interface in TypeScript, you need to use the special implements keyword.
This article explains everything to know about implementing interfaces in TypeScript.
Let's get to it 😎.
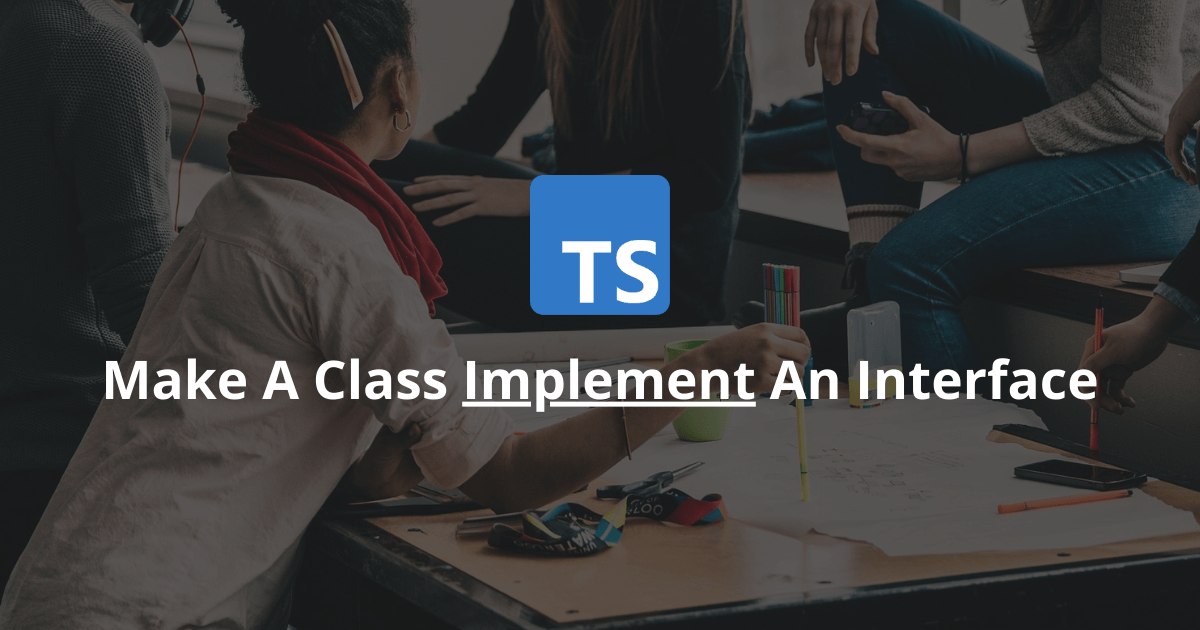
The definition
The implements keyword is used to make a class implement an interface in TypeScript.
Why should you make a class implement an interface, you may ask?
An interface is a contract that an object must follow.
By making a class implement an interface, you ensure that the class contains ALL the properties and methods defined inside the interface.
You can make a class implement an interface for several reasons:
- When you have multiple classes that have the same behavior.
- When you want to make a class more testable.
- When you want to use specific design patterns that require it (for example, dependency injection).
How to make a class implement one interface?
To make a class implement one interface, use the implements keyword in the class definition.
Here is an example:
typescriptinterface IAnimal {
name: string;
eat: () => void;
}
class Dog implements IAnimal {
public constructor(
public name: string
) { }
public eat () {
console.log('The dog is eating.');
}
}
const dog = new Dog('Fluffy');
// "The dog is eating."
dog.eat();
In this example, we first define the IAnimal interface.
Then, we make the Dog class implement the IAnimal interface.
Note: In this example, we use a constructor assignment to define a property inside the constructor.
How to make a class implement multiple interfaces?
In TypeScript, you can make a class implement multiple interfaces by passing a list of interfaces separated by commas to the class.
Here is an example:
typescriptinterface IAnimal {
eat: () => void;
}
interface IDog {
bark: () => void;
}
class Dog implements IAnimal, IDog {
public bark () {
console.log('The dog is barking.');
}
public eat () {
console.log('The dog is eating.');
}
}
const dog = new Dog();
// "The dog is eating."
dog.eat();
// "The dog is barking."
dog.bark();
The Dog class implements the IAnimal AND the IDog interfaces in this example.
Final thoughts
As you can see, making a TypeScript class implement an interface is easy.
Simply use the implements keyword, and voila.
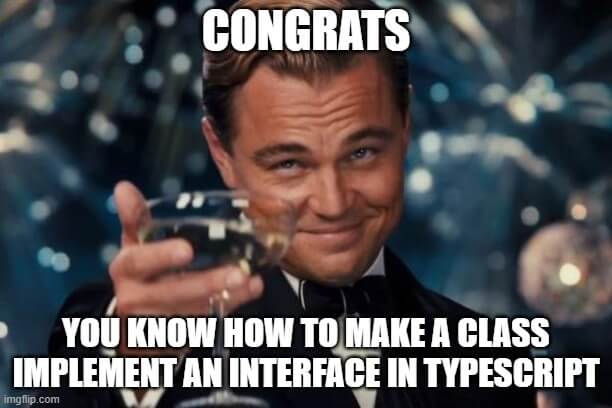
Here are some other TypeScript tutorials for you to enjoy: