How To Create A Queue In TypeScript?
When preparing for a coding interview, you may have encountered the queue data structure and wanted to implement it in a TypeScript project. Luckily, this is easy to do.
The queue data structure is a list of elements that works on a first-in, first-out approach.
This article explains the queue data structure and how to code it in TypeScript.
Let's get to it 😎.
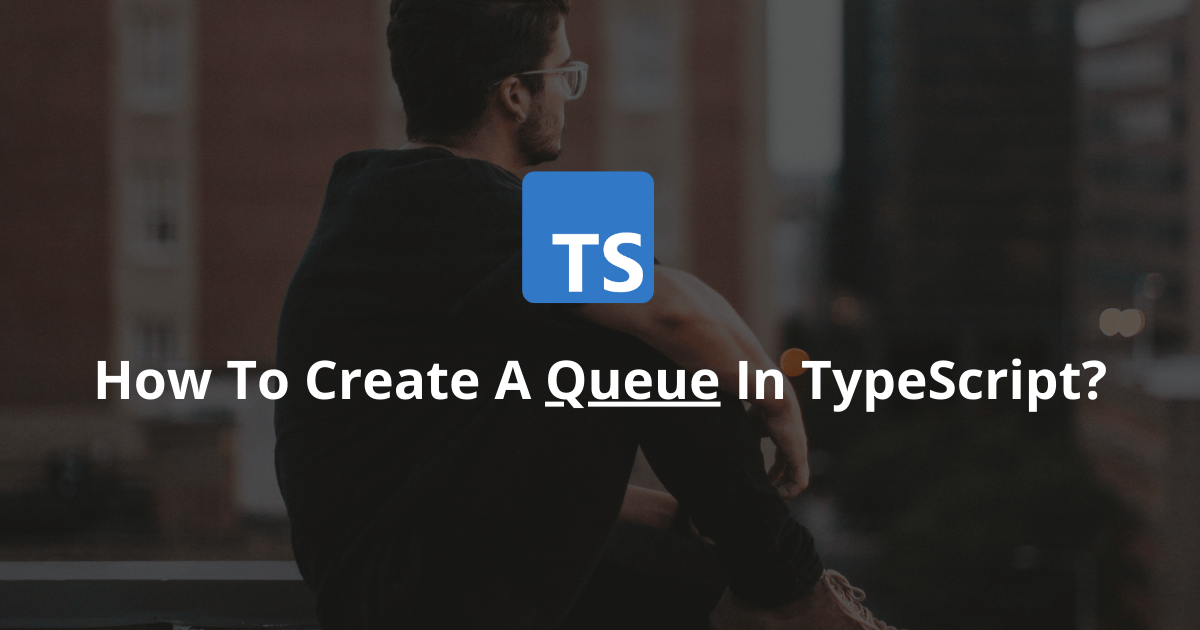
What is a queue?
The queue data structure is a list of ordered elements.
It works based on the first-in, first-out approach (FIFO order).
It has three main operations:
- The enqueue operation inserts a new element to the end of the queue.
- The dequeue operation returns AND removes the first element from the start of the queue.
- The peek operation returns the first element from the start of the queue without modifying it.
The queue's name is derived from a queue at a convenience store. The customer that came first will be served first by the cashier, and the customer that came last will be served last.
How to code a queue in TypeScript?
Unfortunately, TypeScript doesn't have a built-in data structure like a set or a map for the queue.
But we can code it ourselves!
Here is the complete code, which I'll explain later:
typescriptclass Queue<T> {
public constructor(
private elements: Record<number, T> = {},
private head: number = 0,
private tail: number = 0
) { }
public enqueue(element: T): void {
this.elements[this.tail] = element;
this.tail++;
}
public dequeue(): T {
const item = this.elements[this.head];
delete this.elements[this.head];
this.head++;
return item;
}
public peek(): T {
return this.elements[this.head];
}
public get length(): number {
return this.tail - this.head;
}
public get isEmpty(): boolean {
return this.length === 0;
}
}
Read more: Record utility type in TypeScript
Here is what happens in the code:
- We initialize an empty object to hold the queue elements inside the constructor.
- We initialize two variables to track the head and tail positions inside the constructor.
- We code the enqueue method that adds the new element to the elements object.
- We code the dequeue function that removes and returns the first element of the queue.
- We code the peek function that returns the queue's first element.
- We add two get properties, one to get the length of the queue and another one to return if the queue is empty.
As you may have noticed, this queue is generic.
That means you can add any item type you want.
Here is an example of using it:
typescriptconst queue = new Queue<number>();
queue.enqueue(1);
queue.enqueue(3);
queue.enqueue(5);
// Outputs: 3
console.log(queue.length);
// Outputs: 1
console.log(queue.peek());
// Outputs: 1
console.log(queue.dequeue());
In this example, we add three numbers to the queue.
Then we check the length of the queue, output the first element of the queue, and remove and return it.
Final thoughts
As you can see, coding a queue in TypeScript is easy.
Even though you rarely use it when coding applications, knowing and understanding this data structure is crucial.
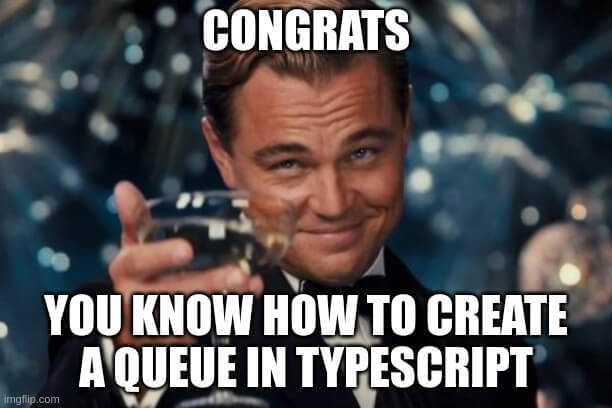
Here are some other TypeScript tutorials for you to enjoy: