How does the TypeScript Set data structure work?
In TypeScript, just like in JavaScript, the Set data structure allows the developer to store unique values inside a list. This data structure was first introduced in ES6, just like the map data structure.
Here is a basic example of how to initialize a Set data structure in TypeScript.
typescriptconst roles = new Set<string>([ 'admin', 'manager' ]);
It is important to know that a value in a Set collection can occur only ONCE... It must be unique.
In this article, I will go over, in detail, about how this data structure works, as well as provide answers to some of the most common questions about this data structure in TypeScript.
Let’s get to it 😎.
Definition
The Set data structure lets the developer store unique values inside a collection. You can store primitives values as well as object references inside a Set collection.
A value in a Set can only occur once.
Set is an ECMAScript 6 data structure, so if you are using an older browser you will need to use a polyfill.
How to add an element to a Set?
You can add elements to a Set using the provided add function.
typescriptconst set1 = new Set();
set1.add(5); // Set [ 5 ]
set1.add(4); // Set [ 5, 4 ]
set1.add(4); // Set [ 5, 4 ]
set1.add(3); // Set [ 5, 4, 3 ]
set1.add('Tim'); // Set [ 5, 4, 3, 'Tim' ]
set1.add({ name: 'Tim' }); // Set [ 5, 4, 3, 'Tim', { name: 'Tim' } ]
Alternatively, you can create all the Set elements at once using an array.
typescriptconst array = [ 4, 5, 6, 4, 5, 'Tim' ];
const set1 = new Set(array);
console.log(set1.keys()); // Outputs: [ 4, 5, 6, 'Tim' ]
As you can see, the Set data structure has conveniently removed all duplicates values.
How to check if a Set contains a key?
To check if a Set collection contains a specific key, use the provided has function.
javascriptconst array = [ 4, 5, 6, 4, 5, 'Tim' ];
const set1 = new Set(array);
set1.has(4); // true
set1.has(9); // false
set1.has('Tim'); // true
The Set data structure methods
Set gives the developer access to a lot of methods that will help manage the collection better.
Method | Description |
---|---|
set.add(value) | Adds an element to a set. |
set.delete(value) | Deletes an element from a set. |
set.has(value) | Returns if the set contains a specific value. |
set.clear() | Removes every element from the set. |
set.values() | Returns an iterator with the values of the set. |
set.keys() | Returns an iterator with the values of the set. Returns the same result as the values() function. |
set.entries() | Returns an iterator object with value/value pairs. |
set.forEach() | Invokes a callback on each value/value pair. I’ve written an extensive article about how does the forEach function work. |
Also, Set gives the developer access to one property.
Property | Description |
---|---|
set.size | Returns the number of elements that the set contains. |
How to iterate over a Set?
To iterate over each item of a Set you can use the for of loop.
typescriptconst set1 = new Set([ 4, 5, 6, 4, 5, 'Tim' ]);
for (const item of set1) {
console.log(item);
}
Alternatively you can use the built-in JavaScript forEach function.
typescriptconst set1 = new Set([ 4, 5, 6, 4, 5, 'Tim' ]);
set1.forEach(item => {
console.log(item);
});
As you can see, it's very easy to iterate over a Set.
How to convert a Set into an array?
To convert a Set into an array you can use the Array.from function.
typescriptconst set1 = new Set([ 4, 5, 6, 4, 5, 'Tim' ]);
const arr = Array.from(set1);
console.log(arr); // Outputs: [4, 5, 6, 'Tim']
Alternatively, you can simply use the spreading syntax.
typescriptconst set1 = new Set([ 4, 5, 6, 4, 5, 'Tim' ]);
const arr = [ ...set1 ];
console.log(arr); // Outputs: [4, 5, 6, 'Tim']
You can also, use the forEach function or a for loop to iterate over each element of the Set and add them to an array.
How to remove duplicates from an array?
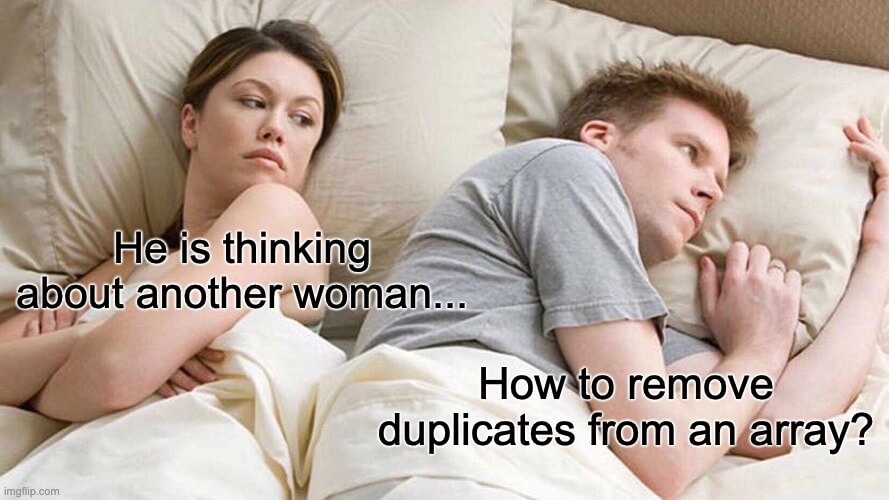
You can remove duplicates from an array using a Set.
Here is how you can do it.
typescriptconst set1 = [ 1, 2, 2, 5, 1, 3 ];
const unique = [ ...new Set(set1) ];
console.log(unique); // Outputs [ 1, 2, 3, 5 ]
How to get a union of two sets?
A union will create a new set containing elements from both collections.
Here is how you can implement a union with two sets.
typescriptconst set1 = new Set([ 1, 2, 3 ]);
const set2 = new Set([ 5, 3, 2 ]);
const union = new Set([ ...set1, ...set2 ]);
console.log(union.keys()); // Outputs [ 1, 2, 3, 5 ]
How to get an intersection of two sets?
An intersection will create a new set containing elements that both sets contain.
This is how you can implement an intersection operation with TypeScript.
typescriptconst set1 = new Set([ 1, 2, 3 ]);
const set2 = new Set([ 5, 3, 2 ]);
const intersection = new Set([ ...set1 ].filter(x => set2.has(x)));
console.log(intersection.keys()); // Outputs [ 2, 3 ]
How to get the difference of two sets?
A difference will create a new set containing elements from set 1 that don't exist in set 2.
This is how you can implement a difference operation with TypeScript sets.
typescriptconst set1 = new Set([ 1, 2, 3 ]);
const set2 = new Set([ 5, 3, 2 ]);
const difference = new Set([ ...set1 ].filter(x => !set2.has(x)));
console.log(difference.keys()); // Outputs [ 1 ]
Set vs Map - How are they different?
Since both data structures were added in ES6, some confuse them and don't understand the difference between them.
Both data structures are completely different data structures.
A Map is a collection of key/value pairs. I've written an extensive article about the TypeScript Map data structure here.
A Set is a collection of unique values.
Final thoughts
The Set data structure, exists in many programming languages, and whether you are a new graduate that hasn't landed their first job or an experienced developer it is very important to master it well and to know when to use it. In my case, I mostly use this data structure when I need to remove duplicates from an array.
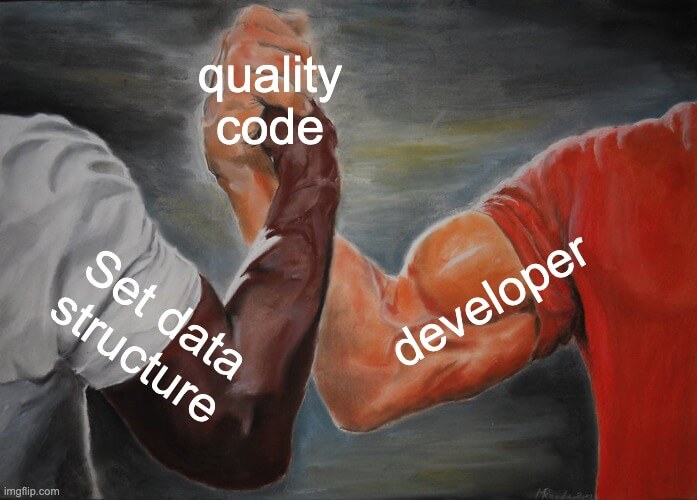
I hope that after reading through this article, you understand this data structure better, and you will start using it.
Thank you for reading and please share this article with your fellow colleagues.