How does the TypeScript forEach function work?
The forEach function lets the developer iterate over an array and executes a callback function on each element of the array. Here is a basic example of the forEach function in TypeScript.
typescriptconst animals = [ 'dog', 'cat', 'cow' ];
animals.forEach((element) => {
console.log(element);
});
// outputs: 'dog', 'cat', 'cow'
The forEach function is very similar to the for loop, but with a few differences, that I will go over in this article. Also, I will provide answers to some of the most common questions about this function.
But first, let's review the definition and the syntax of this function. Let's get to it 😎.
Definition
The forEach function is an array built-in function that was added with ES5 (just like the reduce function).
The forEach function iterates over elements from an array and calls a callback function on each of the array's elements.
Here is the forEach function's syntax.
typescriptarray.forEach(function(value, index, arr), thisValue)
Parameters
Parameter | Necessity | Description |
---|---|---|
function | Required | The callback function that will be executed on each item of the array. |
value | Required | The value of the current item. This is a callback argument. |
index | Optional | The index of the current item. This is a callback argument. |
arr | Optional | The array element that contains the current item. This is a callback argument. |
thisValue | Optional | An optional value that will override the this value. |
Return value
The forEach function always returns undefined.
Browser support
This function works in all major browsers (even IE9).
How to iterate over an array with the forEach function?
Iterating over an array using the forEach function is very easy.
Here is an example of how to do it.
typescriptconst users = [ 'Tim', 'Mark', 'Bob' ];
users.forEach((value, index) => {
console.log(`${value} at index ${index}`);
});
// Tim at index 0
// Mark at index 1
// Bob at index 2
In this example, we output the user name as well as the index of each item by iterating over the users array with the forEach function.
Note: Here we are using string interpolation to concat the strings.
Differences between the for loop vs forEach
Both the for loop and the forEach function are meant to archive a similar goal, that's why it may be confusing of which to use and when.
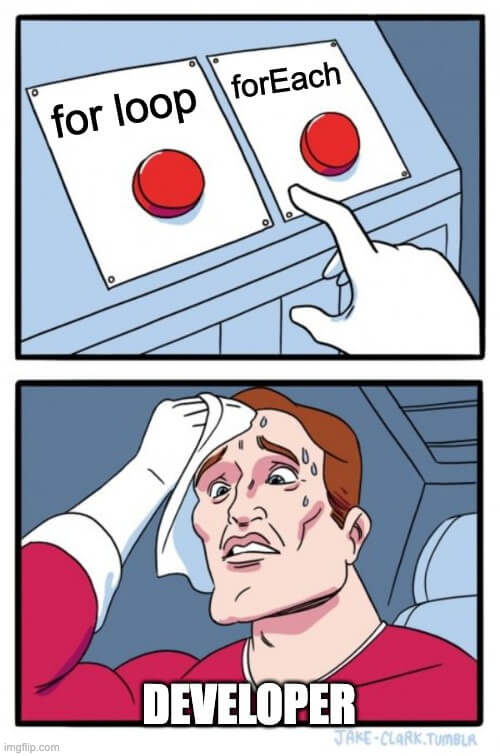
Here are some of the key differences between them...
The for loop
- Better performance overall.
- You can use break/continue inside a for loop. (early loop termination)
- You can control how the main condition behaves.
The forEach function
- Requires no variable setup.
- More maintainable & easier to read.
You should only use the forEach function when you need to iterate over EACH item of an ARRAY.
In all other cases, use a for loop.
How to break or exit inside a forEach function?
Unfortunately, there is no way to stop or break a forEach function execution.
This is why you should only use this function when you want to iterate over EVERY item of an array.
There are some workarounds to archive this behavior, like using a try catch statement, but there are counter-intuitive, and you shouldn't use them.
To return/break while iterating over an array, you need to use a for loop.
How to iterate over a map with the forEach function?
To iterate over a map in TypeScript you need to use the built-in Map forEach function.
Here is an example of how to do it.
typescriptconst map = new Map([
[ 'a', 1 ],
[ 'b', 2 ],
[ 'c', 3 ]
]);
map.forEach((value, key) => {
console.log(`key: ${key} | value: ${value}`);
});
// key: a | value: 1
// key: b | value: 2
// key: c | value: 3
This example iterates over a Map and prints the key and the value of each item.
Final thoughts
As you can see, the forEach function is a great way to simplify your code, but you can only use it on arrays and when you don't have to break early from the iteration.
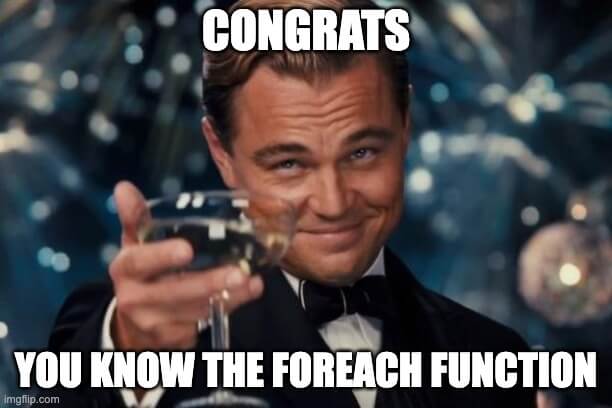
I hope you've enjoyed reading this article, please share it with your fellow developers.