How To Perform String Interpolation In TypeScript?
When using TypeScript, developers often need to replace placeholders with values inside a string. This operation is called string interpolation.
To perform string interpolation in TypeScript, create a template literal like so:
typescriptconst age = 20;
console.log(`Your age is ${age}`);
This article explains template literals and string interpolation in TypeScript and shows code examples.
Let's get to it 😎.
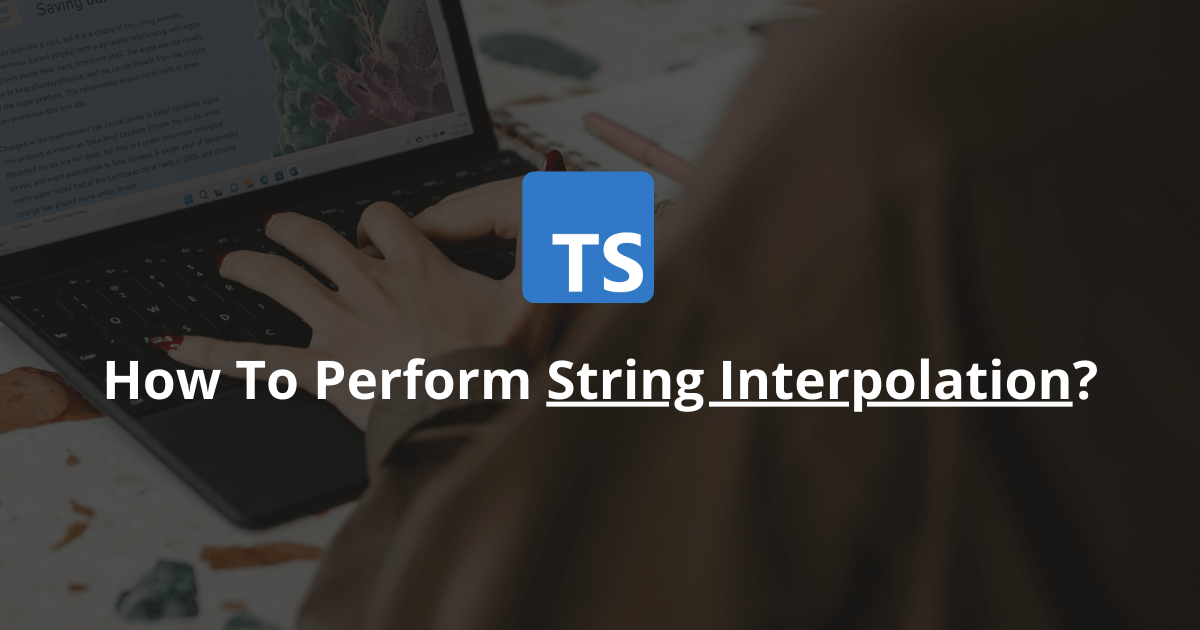
What is a template literal?
Template literals, also called template strings, were added in ES6.
They allow developers to:
- Create a multi-line string
- Perform string interpolation
- Create a tagged template
A template literal is delimited with backtick ` characters.
Here is an example of a template literal:
typescriptconst lit = `My name is Tim`;
Performing string interpolation
An interpolated string allows the combining of multiple expressions into one string. In short, it enables replacing placeholders in a string with values. This operation used to be hard to do in legacy JavaScript.
Before template literals, developers needed to use the + operator to concatenate strings.
Here is an example of this:
typescript// Outputs: 'My name is Tim'
console.log('My name is ' + 'Tim');
This can be hard to maintain, especially when using multiple variables. Also, you needed to add spaces manually.
An easier way involves using template literals, which allow developers to use variables directly inside them. To add a variable inside a template literal, you need to use the special dollar sign ${} notation.
Here is the same example but using a template literal:
typescriptconst myName = 'Tim';
// Outputs: 'My name is Tim'
console.log(`My name is ${myName}`);
As you can see, using template literals to concatenate strings is much more readable.
You can also perform arithmetic operations inside template expressions.
Here is an example:
typescriptconst a = 5;
const b = 20;
const c = 30;
// Outputs: 'The result is: 3000'
console.log(`The result is: ${a * b * c}`);
You can also use functions inside template expressions.
Here is an example:
typescriptconst getName = (first: string, last: string): string => {
return `${first} ${last}`;
}
// Outputs: 'My name is: Tim Mousk'
console.log(`My name is: ${getName('Tim', 'Mousk')}`);
Finally, you can use the double question mark operator or the double pipe operator to set a default fallback.
Here is an example:
typescriptconst getName = (first: string): string => {
return `My name is: ${first ?? 'not_defined'}.`
}
// Outputs: 'My name is: Tim.'
console.log(getName('Tim'));
// Outputs: 'My name is: not_defined.'
console.log(getName('not_defined'));
How to perform a multi-line string interpolation?
Template literals also allow developers to perform multi-line string interpolation.
Here is an example:
tyescriptconst myName = 'Tim';
const age = 27;
console.log(`
My name is: ${myName}
My age is: ${age}`);
How to escape the dollar sign with a template literal?
Sometimes, you need to use the backslash \ character to escape special characters inside a template literal.
Here is an example:
typescript// Outputs: '${'
console.log(`$\{`);
Final thoughts
As you can see, using template literals helps developers quickly replace placeholder values in a string.
They also allow developers to create multi-line strings, which used to be difficult to maintain using the + operator.
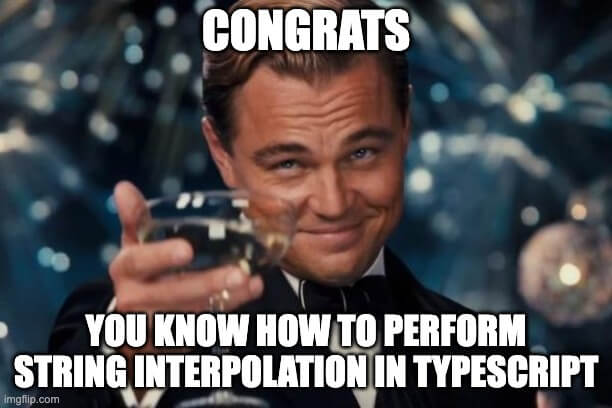
Here are some other TypeScript tutorials for you to enjoy: