How To Convert A String To Boolean In TypeScript?
When developing a TypeScript project, developers often need to convert a string to a boolean. But how do you do it?
This article shows multiple methods to convert a string to a boolean in TypeScript with code examples.
Let's get to it 😎.
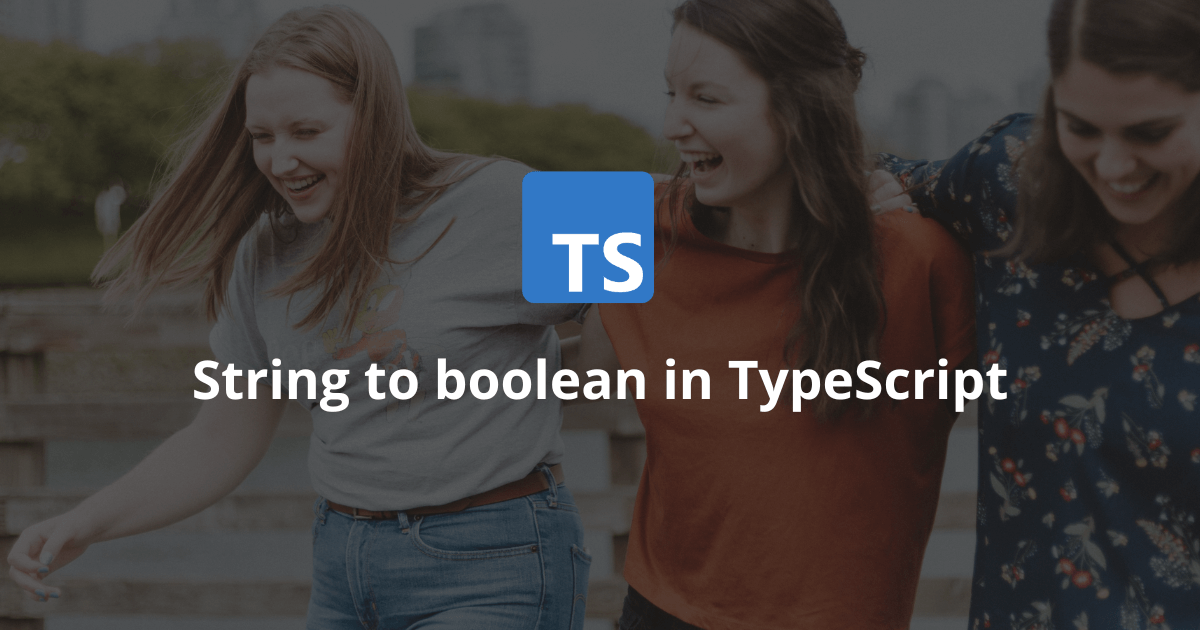
Solution #1 - Use the strict equality operator
The simplest method to convert a string to a number in TypeScript involves using the strict equality operator (also called the identity operator) to check if a string equals true.
Here is an example:
typescriptconst strValue = 'true';
const boolValue = strValue === 'true';
// Outputs: true
console.log(boolValue);
This solution is case-sensitive, meaning it will not work if the string value begins with an uppercase letter. To make the solution case-intensive, you can transform the string value to lowercase.
Here is the case-insensitive solution:
typescriptconst strValue = 'True';
const boolValue = strValue.toLowerCase() === 'true';
// Outputs: true
console.log(boolValue);
Solution #2 - Use a regex
Another solution to convert a string to a number in TypeScript involves using a regex.
Here is an example:
typescriptconst strValue = 'true';
const boolValue = /^\s*(true|1|on)\s*$/i.test(strValue);
// Outputs: true
console.log(boolValue);
This solution is case-insensitive, AND it matches true, 1 and on.
Solution #3 - Use the Boolean object
Using the JavaScript built-in Boolean object, you can also convert a string to a boolean.
Here is an example:
typescriptconst v1 = Boolean('true'); // Returns true
const v2 = Boolean('false'); // Returns true
const v3 = Boolean(' '); // Returns true
const v4 = Boolean(null); // Returns false
As you can see, this method determines if a value is truthy, as opposed to if a string equals true, so this solution may only be suited for some use cases.
Solution #4 - Use the JSON.parse function
Finally, you can convert a string to a boolean using the built-in JSON.parse function.
Here is an example:
typescript// Returns true
const v1 = JSON.parse('true'.toLowerCase());
// Returns false
const v2 = JSON.parse('false'.toLowerCase());
Final thoughts
As you can see, casting a string to a boolean is simple in TypeScript.
You have many different methods to choose from.
Which one you choose is a matter of preference.
As for myself, I prefer the strict equality operator method (usually).
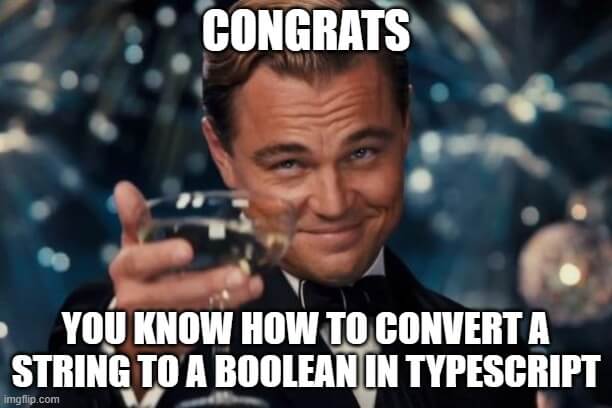
Here are some other TypeScript tutorials for you to enjoy: