How To Convert A String To A Number In TypeScript?
When coding with TypeScript, developers sometimes need to convert a string to a number. Luckily, JavaScript provides many different ways to do it that you can also use in TypeScript.
The easiest way to cast a string to a number in TypeScript is to use the Number constructor like so:
typescriptconst myString = '444222';
const myNumber = Number(myString);
// Outputs: 444222
console.log(myNumber);
This article will go through different methods of converting a string to a number in TypeScript and show how to use each.
Let's get to it 😎.
Method #1 - Using the Number constructor
Perhaps, the easiest way to cast a string to a number is to use the JavaScript Number constructor.
This function works with integers and with floats.
typescript// Outputs: 9999
console.log(Number('9999'));
// Outputs: NaN
console.log(Number('asdqwewe2'));
Note: If the function cannot cast the string into a number, it will return a NaN (not a number).
Method #2 - Using the parseInt function
The JavaScript parseInt function only works with integers.
If you attempt to pass a string with floating-point values, they will be cut off.
typescript// Outputs: 55
console.log(parseInt('55'));
// Outputs: 23
console.log(parseInt('23.22'));
Method #3 - Using the parseFloat function
The parseFloat function behaves the same as the parseInt function, except it accepts floating point values.
typescript// Outputs: 55
console.log(parseFloat('55'));
// Outputs: 23.22
console.log(parseFloat('23.22'));
Method #4 - Using multiplication by one
One less known JavaScript trick is to multiple a string by one, which converts the string into a number.
Here is how to do in TypeScript:
typescript// Outputs: 2323.12
console.log("2323.12" as any * 1);
// Outputs: 444
console.log("444" as any * 1);
// Outputs: -55
console.log("-55" as any * 1);
As you can see, you must first cast the string to an any type.
Otherwise, the TypeScript compiler will complain.
Method #5 - Using the double tilde operator
Using the double tilde operator, you can convert a string to a number in TypeScript.
However, values after the decimal point will be cut off.
typescript// Outputs: 2323
console.log(~~"2323.12");
// Outputs: 444
console.log(~~"444");
Final thoughts
As you can see, you have plenty of methods to choose from when casting a string to a number.
When it comes to me, most of the time, I use the Number constructor.
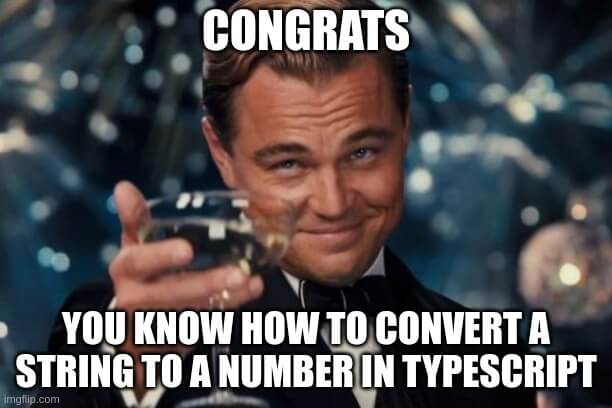
Here are some other TypeScript tutorials for you to enjoy: