How Does The Reduce Function Work In TypeScript?
The reduce function lets the developer iterate over an array and execute a reducer on each array element. This function returns a single result. Here is a simple example of the reduce function in TypeScript.
typescriptconst arr = [ 1, 5, 7, 3 ];
const sum = arr.reduce((a: number, b: number) => {
return a + b;
}, 0);
// Outputs: 16
console.log(sum);
In this article, I will explain the reduce function in detail, how to use it in TypeScript, and give different examples of typical use cases.
Let's get to it 😎.
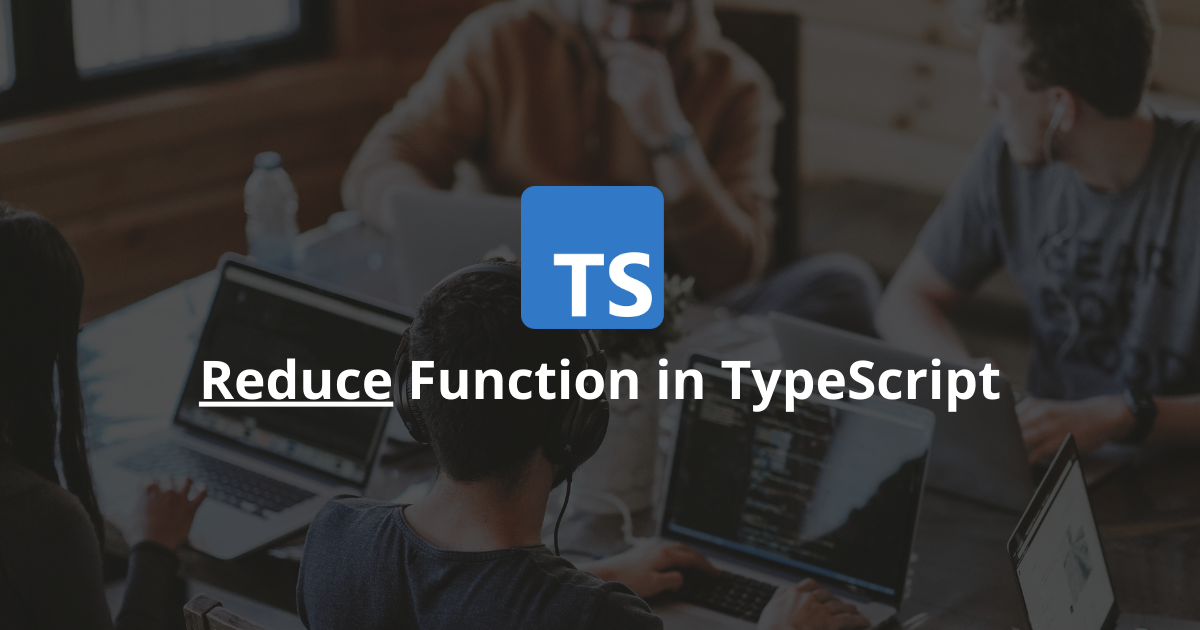
The definition
The reduce function is a built-in array function added in ES5.
The reduce function executes a reducer on each array's element and returns the accumulated result in a single result.
Here is the reduce function's syntax.
typescriptarray.reduce(function(total, value, index, array), initialValue)
Parameters
Parameter | Necessity | Description |
---|---|---|
function | Required | The reducer to execute on each array item. |
total | Required | The previously returned value (or initial value). |
value | Required | The current element value. |
index | Optional | The current element index. |
array | Optional | The whole array. |
initialValue | Optional | The initial value of the total. |
Return value
The reduce function returns the accumulated result from the last call of the function.
Note: In TypeScript, you can specify the return type of this function by using a generic argument.
Browser support
The reduce function works on all major browsers, including IE9, IE10, and IE11 🥳.
How to type the reduce function in TypeScript?
Sometimes, the TypeScript compiler cannot infer the reduce function return type.
This is when you need to specify a return type.
The reduce function accepts an optional generic argument to specify the return type.
Here is an example of this:
typescriptconst arr = [
{ firstName: 'Tim' },
{ lastName: 'Mousk' }
];
type Person = {
firstName: string;
lastName: string;
}
const person = arr.reduce<Person>((acc, current) => {
return { ...acc, ...current };
}, {} as Person);
// Outputs: { firstName: "Tim", lastName: "Mousk" }
console.log(person);
In this case, we create a person object by reducing each array item and specifying a return type.
In this example, we are using the spread operator to merge objects.
How to find the sum with reduce?
To find the sum of array elements, use the reduce function like so:
typescriptconst arr = [ 5, 2, 5, 12 ];
const sum = arr.reduce((a: number, b: number) => {
return a + b;
}, 0);
// Outputs: 24
console.log(sum);
How to calculate the average with reduce?
To calculate the average with the reduce function, you need to get the accumulated result sum and divide it by the array's length.
Here is an example of how to do it:
typescriptconst arr = [ 10, 4, 7, 3 ];
const sum = arr.reduce((a: number, b: number) => {
return a + b;
}, 0);
const average = sum / arr.length;
// Outputs: 6
console.log(average);
How to reduce an array of objects?
The reduce function works very well on an array of objects.
Here is an example of a reducer on an array of objects:
typescriptconst arr = [
{ value: 32 },
{ value: 12 },
{ value: 4 }
];
const sum = arr.reduce((a, b) => {
return a + b.value;
}, 0);
// Outputs: 48
console.log(sum);
How to use reduce with a condition?
To execute a reducer conditionally, you need to use the filter function with the reduce function.
Here is an example of a reduce with a condition:
typescriptconst persons = [
{
role: 'admin',
value: 22
},
{
role: 'manager',
value: 30
},
{
role: 'admin',
value: 31
}
];
const valueSum = persons
.filter(({ role }) => role === 'admin')
.reduce<number>((sum: number, person) => {
return sum + person.value
}, 0);
// Outputs: 53
console.log(valueSum);
In this example, we get the sum of all the admin's values in the array.
Final thoughts
In conclusion, the reduce function is a great way to simplify your TypeScript code and eliminate unnecessary calls to the forEach function.
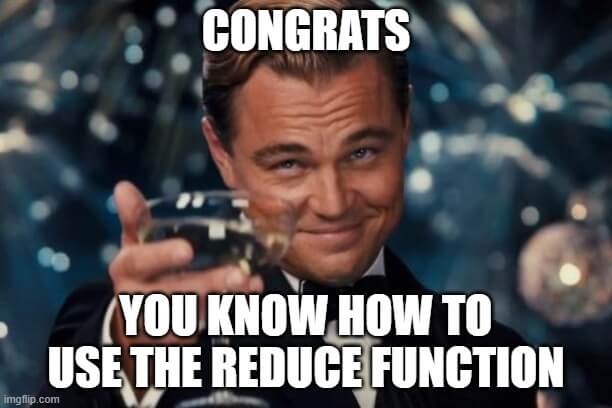
I hope you've enjoyed reading this article.
Please share it with your fellow coders.