How To Merge Objects In TypeScript?
When creating a TypeScript project, developers often need to merge objects. But how do you do it?
To merge objects in TypeScript, a developer can:
- Use the spread operator
- Use Object.assign
- Use the Lodash merge function
This article explains merging objects in TypeScript and shows multiple solutions with code examples.
Let's get to it 😎.
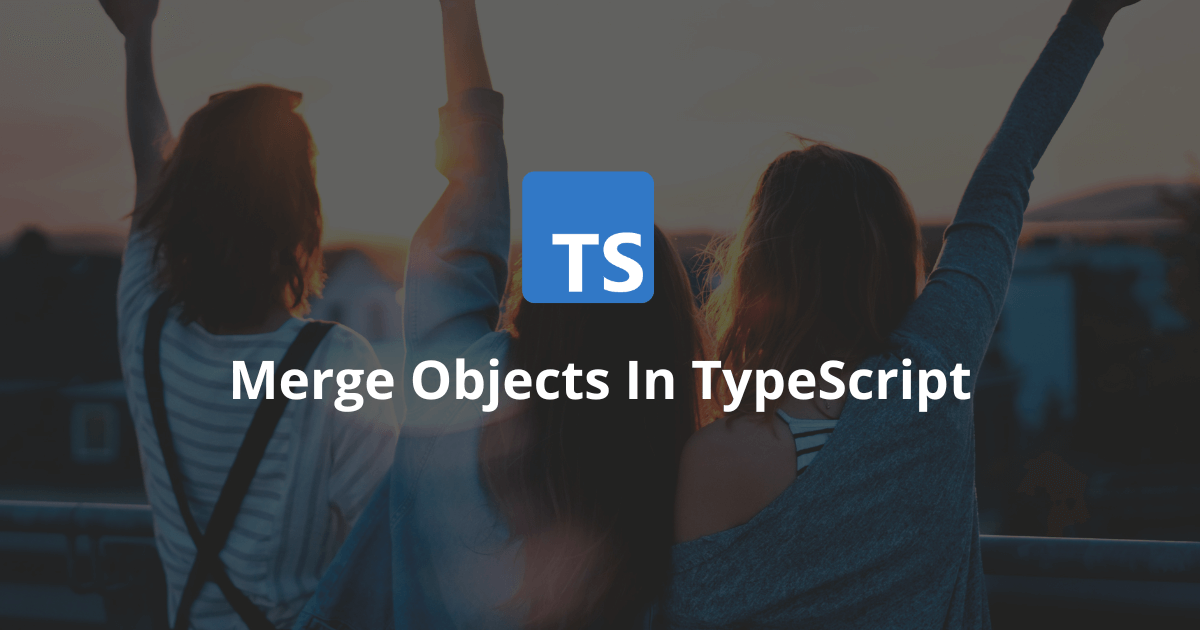
Solution #1 - Use the spread operator
The easiest solution to merge objects in TypeScript involves using the spread operator.
Here is an example:
typescriptconst obj1 = {
name: 'Tim'
};
const obj2 = {
role: 'Admin'
};
const merged = {
...obj1,
...obj2
};
// Outputs: { "name": "Tim", "role": "Admin" }
console.log(merged);
As you can see, the merged object now contains properties from obj1 and obj2.
Note:Â This solution works well when merging primitive type values because this method does a shallow copy. If you want to merge complex values (objects, arrays), use another solution (see solution #3).
Solution #2 - Use Object.assign
Another option for merging objects is to use the Object.assign built-in function. This function works similarly to the spread operator and also does a shallow copy.
Here is an example:
typescriptconst obj1 = {
name: 'Tim'
};
const obj2 = {
role: 'Admin'
};
const merged = Object.assign({}, obj1, obj2);
// Outputs: { "name": "Tim", "role": "Admin" }
console.log(merged);
Solution #3 - Use the Lodash merge function
The Lodash merge function is great when you want to merge complex values. Indeed, this function does a deep copy, so the newly created object will NOT share any references with the original objects.
Before using this function, you must install Lodash using npm or yarn.
Here is an example of merging two objects using Lodash:
typescriptimport { merge } from 'lodash';
const obj1 = { startYear: 2000 };
const obj2 = { endYear: 2022 };
const merged = merge(obj1, obj2);
// Outputs: '{ endYear: 2022, startYear: 2000 }'
console.log(merged);
Note: Changes to the newly created object WILL NOT affect the original objects.
Final thoughts
As you can see, you have multiple solutions to merge objects in TypeScript.
On the one hand, when you need to merge primitive values, you can use the spread operator or Object.assign.
On the other hand, when you need to merge complex values, you can use the Lodash merge function.
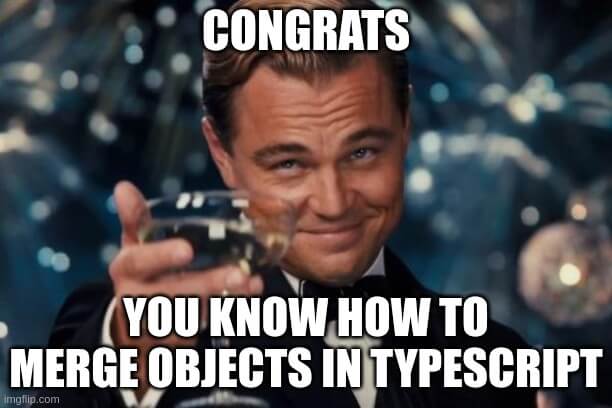
Here are some other TypeScript tutorials for you to enjoy: