How To Build A Dictionary In TypeScript?
The dictionary, also called map or associative array, is one of the fundamental data structures in computer science. But what is it, and how to build one in TypeScript?
In TypeScript, you can build a dictionary using:
- An indexed interface
- The Record utility type
- The ES6 built-in Map object
This article explains the different methods of building a dictionary with clear code examples.
Let's get to it 😎.
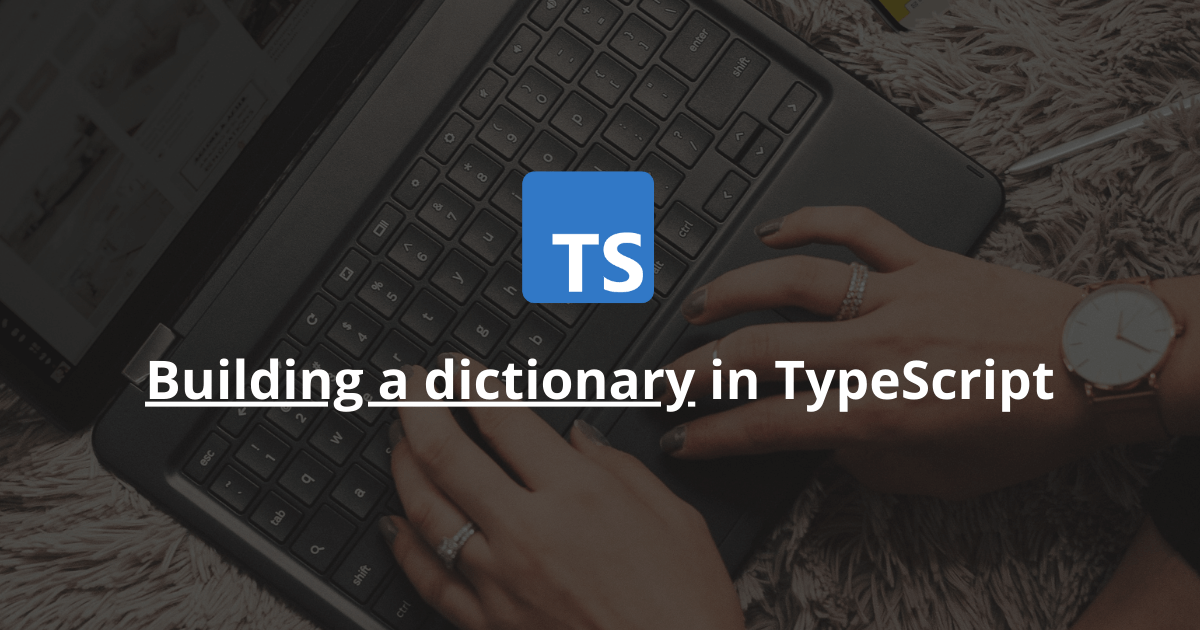
What is a dictionary?
A dictionary is an unordered collection of key/value pairs.
This data structure must follow those simple rules:
- Each key must be unique.
- Each key must have an associated value.
Generally, a dictionary's key is of type string, but it also can be of type number.
On the other hand, each key's value can be of any type you want.
Way #1 - Using an indexed interface
The easiest way to build a dictionary is to create an annotated indexed interface AND use it on an empty object.
Here is an example:
typescriptinterface IDictionary {
[key: string]: string;
}
const dictionary: IDictionary = {};
dictionary['key1'] = 'value1';
dictionary['key2'] = 'value2';
// To verify if a key exists.
// Outputs: true
console.log('key1' in dictionary);
// To get a key's value.
// Outputs: 'value1'
console.log(dictionary['key1']);
As you can see, in this example, we create a simple dictionary AND add key/value pairs.
You can also create a generic indexed interface if you want to reuse the dictionary interface with different value types.
Here is an example:
typescriptinterface IDictionary<T> {
[key: string]: T;
}
const dictionary: IDictionary<string> = {};
dictionary['key1'] = 'value1';
const dictionary2: IDictionary<number> = {};
dictionary2['key1'] = 1;
In this example, the first dictionary can only contain string values, and the second can only have number values.
Way #2 - Using the Record utility type
Another option to build a dictionary in TypeScript involves using the Record utility type.
Here is an example:
typescriptconst dictionary: Record<string, string> = {};
dictionary['key1'] = 'value1';
dictionary['key2'] = 'value2';
This method is similar to the indexed interface but easier since you don't have to create a new interface.
Way #3 - Using the ES6 built-in Map object
Finally, you can create a dictionary using the ES6 built-in Map object.
Here is an example:
typescriptconst dictionary = new Map<string, string>();
dictionary.set('key1', 'value1');
dictionary.set('key2', 'value2');
// To verify if a key exists.
// Outputs: true
console.log(dictionary.has('key1'));
// To get a key's value.
// Outputs: 'value1'
console.log(dictionary.get('key1'));
Compared to other methods, the main advantage of using the Map object is its API methods to manage key/value pairs.
Final thoughts
As you can see, building a dictionary is simple in TypeScript.
In my day-to-day programming life, I prefer to create dictionaries using the ES6 built-in Map object. This method is the simplest to understand for new programmers and easy to refactor.
A polyfill exists if you want to use the ES6 Map object in older browsers.
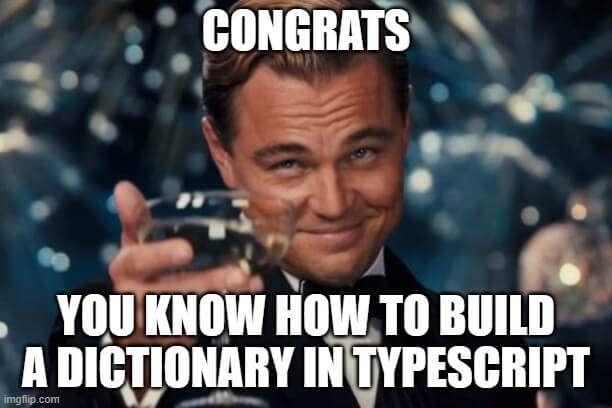
Here are some other TypeScript tutorials for you to enjoy: