How To Check For Undefined In TypeScript?
In TypeScript, checking if a variable or argument is defined is one of the most common tasks. Luckily, it is simple to accomplish.
The easiest way to check for undefined in TypeScript is to use a condition check, like so:
typescriptconst myName: string | undefined;
if (myName === undefined) {
console.log('name is undefined');
}
This article will explain checking for undefined in TypeScript and answer some of the most common questions.
Let's get to it 😎.
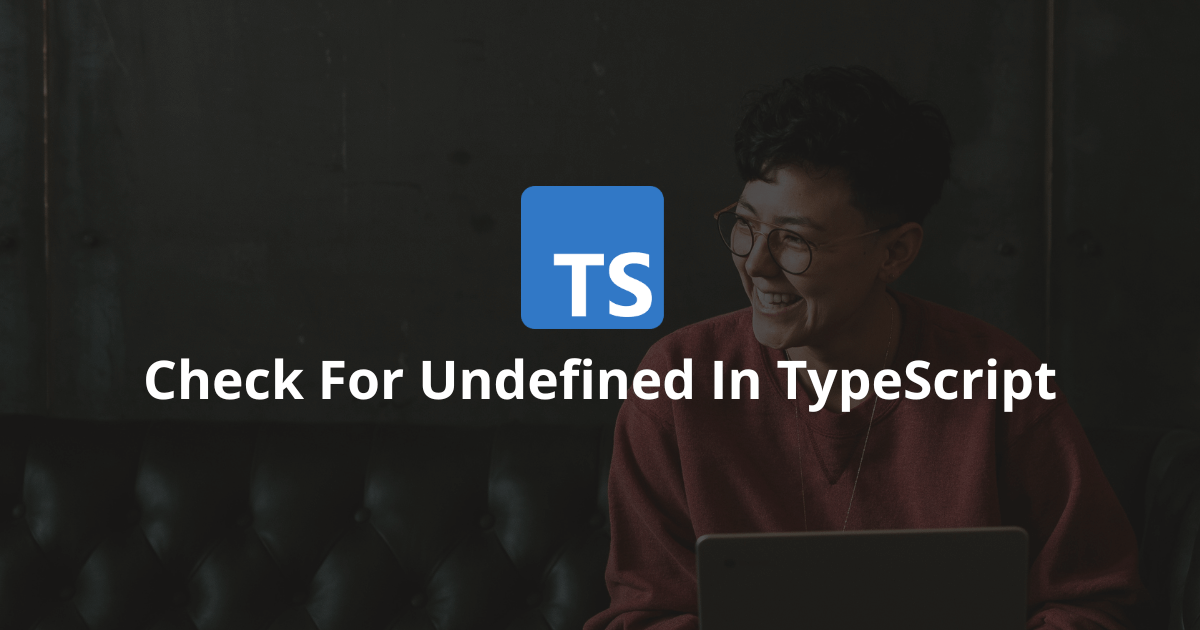
In JavaScript, undefined is a primitive data type that can indicate that:
- A variable is not initialized. (for example, when a developer tries to access a non-existing property of an object).
- A variable has no assigned value to it.
How to check for undefined using an if statement?
To check for undefined, in TypeScript, just like in JavaScript, the developer can use a condition check, like so:
typescriptconst myEmail: string | undefined;
if (myEmail === undefined) {
console.log('email is undefined');
} else {
console.log('email is defined');
}
In this example, we check if myEmail is equal to undefined and output the correct log.
How to check for undefined using falsy values?
Another option to check for undefined in TypeScript is to check for falsy values.
In JavaScript, falsy values are a list of values that return false in a boolean context.
Here is how to check if a constant is falsy in TypeScript:
typescriptconst myEmail: string | undefined;
if (!myEmail) {
console.log('email is undefined');
}
This code not only checks for undefined but also for other falsy values.
Other JavaScript falsy values include: false, 0, -0, 0n, '', null, NaN.
How to check for undefined before calling a function?
In TypeScript, you can use the optional chaining operator to check if an object is undefined before calling a function.
Here is an example of how to do it:
typescriptconst myEmail: string | undefined;
myEmail?.indexOf('abc');
In this example, if the myEmail constant is undefined, the indexOf function is never called (so the code doesn't break).
How to provide a default fallback for undefined?
TypeScript provides the nullish coalescing operator to help set a fallback for an undefined value.
Here is an example of how to do it:
typescriptlet myEmail = email ?? 'info@admin.com';
In this example, if the email variable is undefined, myEmail fallbacks to the default email.
Final thoughts
As you can see, checking for undefined in TypeScript is simple.
You just need to do a condition check, and voila.
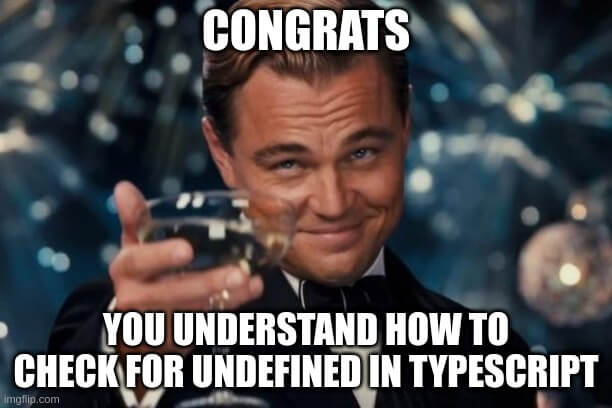
Here are other TypeScript tutorials for you to enjoy: