How Does The Boolean Data Type Work In TypeScript?
TypeScript provides support for many primitive data types. One of them is the boolean type.
This article explains the boolean data type in TypeScript and shows many code examples.
Let's get to it 😎.
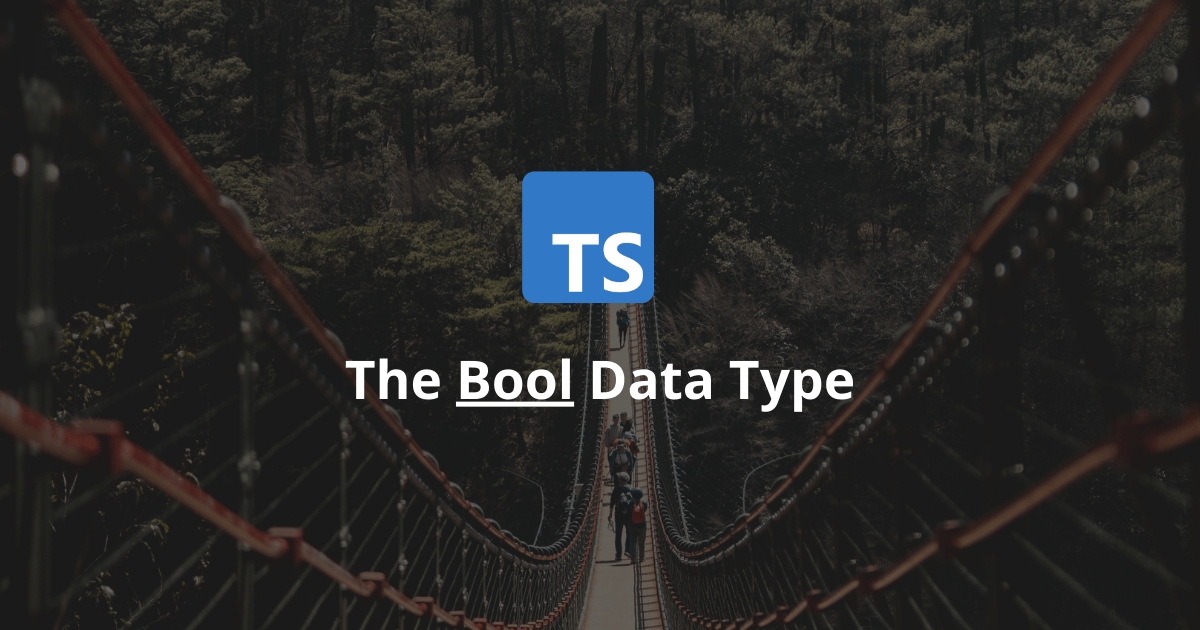
The definition
The boolean is one of the primitive data types of JavaScript and TypeScript.
Its value can be either true or false.
Here is an example:
typescriptconst obj1 = true;
const obj2 = false;
Boolean vs boolean
A lot of developers confuse Boolean and boolean.
boolean always refers to a primitive data type.
Boolean can refer to an object type or a global function. Using the Boolean function, you can cast a value to a boolean. The Boolean object creates a new Boolean object instance.
typescript// The Boolean function casts any value to a boolean.
const obj1 = Boolean(1);
// The Boolean object constructs a new Boolean object.
const obj2 = new Boolean(1);
// Outputs: true, 'boolean'
console.log(obj1, typeof obj1);
// Outputs: Boolean: true, 'object'
console.log(obj2, typeof obj2);
In TypeScript, you must use boolean when defining a constant or a function's parameter.
When casting a variable to a boolean, use the Boolean function.
Truthy & Falsy in TypeScript
TypeScript, just like JavaScript, has the concept of truthy and falsy in a Boolean context (for example, in an if statement).
Truthy values are considered true in a Boolean context. A value is truthy unless it's one of the falsy values.
Here are the falsy values in TypeScript:
- false
- 0
- -0
- 0n
- ""
- null
- undefined
- NaN
Here is an example:
typescript// All of those values are truthy.
Boolean(1);
Boolean("Tim");
Boolean(new Date());
Boolean([]);
Boolean({});
Boolean("2");
Boolean(-42);
// All of those values are falsy.
Boolean(false);
Boolean(0);
Boolean(-0);
Boolean(null);
Boolean(undefined);
Boolean(NaN);
How to convert a boolean to a string?
To convert a boolean to a string, you can use the String object.
Here is an example:
typescriptconst a = true;
const b = false;
// Outputs: 'true'
console.log(String(a));
// Outputs: 'false'
console.log(String(b));
How to convert a boolean to a number?
To convert a boolean to a number, you can use the Number object.
Here is an example:
typescriptconst a = true;
const b = false;
// Outputs: 1
console.log(Number(a));
// Outputs: 0
console.log(Number(b));
Final thoughts
As you can see, the boolean type is no rocket science.
It is one of the primitive data types in TypeScript, so it is essential to understand it well.
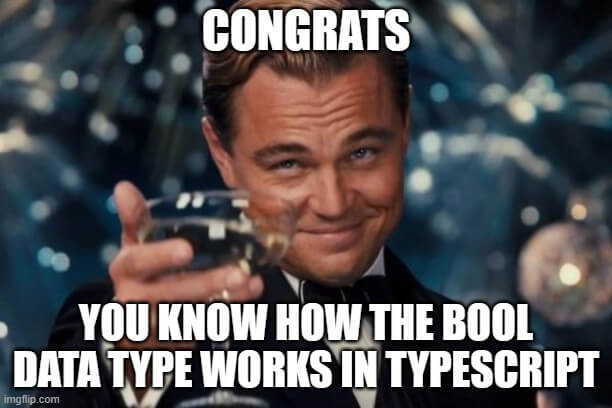
Here are some other TypeScript tutorials for you to enjoy: