What Is The Exclamation Mark Operator In TypeScript?
When looking through other TypeScript libraries' source code, maybe you have encountered a strange exclamation mark operator placed after a member. This operator is a TypeScript-only feature and does not exist in JavaScript. It is called the non-null assertion operator.
The non-null assertion operator (or exclamation mark operator) asserts that an optional value is both:
- non-null
- non-undefined
This article will go through everything about the exclamation mark operator in TypeScript and answer some common questions.
Let's get to it 😎.
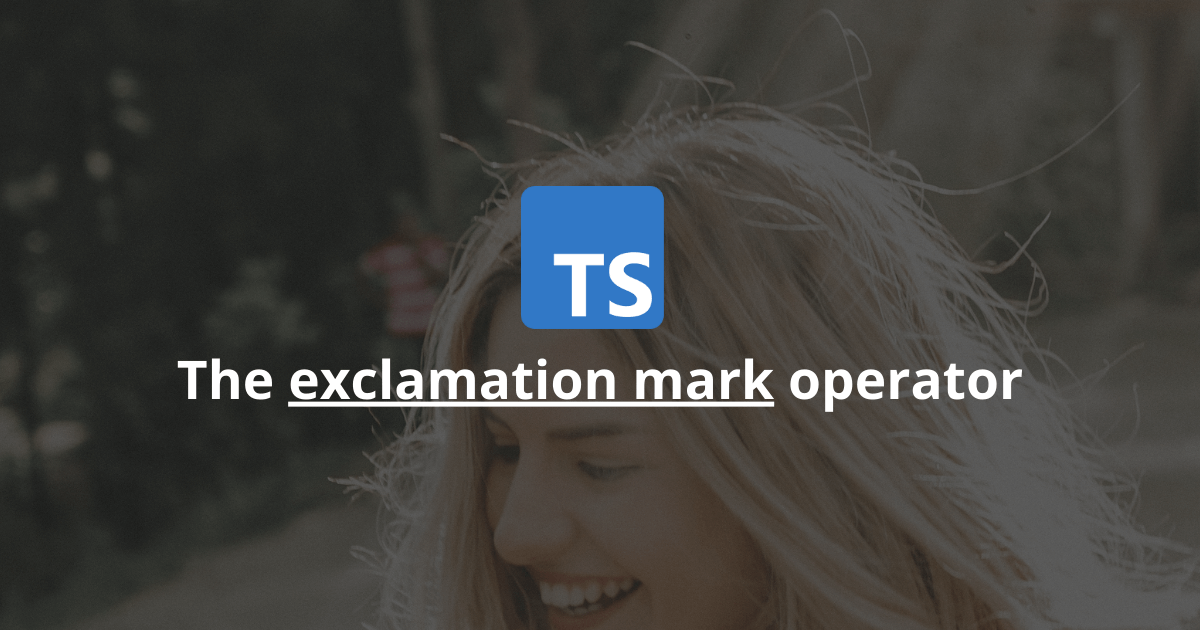
The definition
The exclamation mark operator or non-null assertion operator asserts that an optional value cannot be null or undefined.
Some popular use cases for the non-null assertion operator include:
- When you don't want to handle any null or undefined and want to treat a variable as a solid type.
- To fix the "The left-hand side of an assignment expression may not be an optional property access." error.
🚨 Remember: The non-null assertion operator does not change the runtime of your code. If a value you asserted as non-null turns out null, it will break your code.
Here is an example of the exclamation mark operator in action:
typescriptconst logUppercased = (str?: string): void => {
console.log(str!.toUpperCase());
}
In this example, the string parameter of the logUppercased function is an optional parameter. Using the exclamation mark operator, we tell the TypeScript compiler to treat it as a solid value.
Only use this operator when you are 100% sure of what you are doing.
A safer alternative to the non-null assertion operator
Since the non-null assertion operator doesn't affect the runtime, your code can break.
That is where the optional chaining operator comes into play.
The optional chaining operator allows access to a nested property of an object AND automatically handles if the parent's value is null or undefined.
Here is the same example, but this time using an optional chaining operator:
typescriptconst logUppercased = (str?: string): void => {
console.log(str?.toUpperCase());
}
This code is safer because the optional chaining operator automatically handles it if the string is not defined.
If the string is not defined, the toUpperCase function will not execute.
When the exclamation mark operator might be appropriate
The exclamation mark operator might be appropriate when you need to fix the "The left-hand side of an assignment expression may not be an optional property access" error.
This error happens when you want to assign a value to a child property of an optional parent.
In this situation, the optional chaining operator won't work.
Here is an example of how to fix this error using the non-null assertion operator:
typescriptinterface IArticle {
content: string;
}
const modifyContent = (article?: IArticle): void => {
article!.content = 'new content';
}
Read more: How do interfaces work in TypeScript?
In this example, we tell the TypeScript compiler that the article is always defined.
Again, only use this when you are 100% sure of what you are doing.
Final thoughts
As you can see, the exclamation mark operator tells the TypeScript compiler to treat an optional value as a solid type.
Since the exclamation mark operator does not affect the runtime, you must be sure of what you are doing. If an optional value marked by a non-null assertion operator as a solid type turns out to be null or undefined, it will break your code.
That's why in most cases, I recommend using optional chaining, which is a safer alternative.
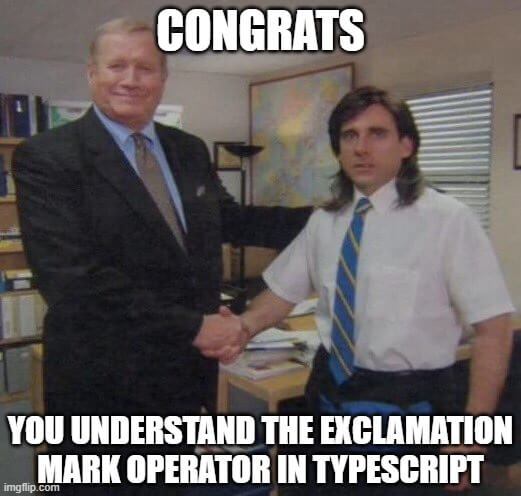
Here are some other TypeScript tutorials for you to enjoy: