How To Ignore The Next Line In TypeScript?
The TypeScript compiler is excellent at showing errors and warning when it detects something is wrong with the code. Sometimes, however, a developer may want to ignore an error on the next line AND still compile the code. Luckily, this is simple to achieve in TypeScript.
To ignore a compiler error on the next line in TypeScript, you can use the @ts-ignore rule, like so:
typescriptif (false) {
// @ts-ignore
console.log("unreachable code");
}
This article will go through everything on ignoring compiler errors and warnings in TypeScript and answer some common questions.
Let's get to it 😎.
In TypeScript, you can ignore compiler errors by adding a TypeScript directive comment above the line with the error you want to silence.
To silence TypeScript errors, multiple types of comments exist:
- @ts-ignore
- @ts-expect-error
- @ts-nocheck
How to ignore an error with @ts-ignore?
The @ts-ignore comment allows suppressing an error on the next line.
Here is an example of how to use the @ts-ignore comment:
typescriptwhile(true) {
console.log('a');
}
// @ts-ignore
console.log('b')
Read: The while loop in TypeScript
In this example, the second console.log is unreachable.
With the @ts-ignore directive comment, we silence this error.
How to ignore an error with @ts-expect-error?
The @ts-expect-error comment was introduced in TypeScript version 3.9.
It has the same behavior as the @ts-ignore comment, but with one difference.
The @ts-expect-error comment suppresses the next line error just like the @ts-ignore comment.
However, if there is no error to suppress, the compiler shows a warning that the directive is unnecessary.
Here is the same code example as above, but this time it uses a @ts-expect-error:
typescriptwhile(true) {
console.log('a');
}
// @ts-expect-error
console.log('b')
In this example, we silence the "Unreachable code detected" error.
The behavior of the directive
In this example, the @ts-expect-error comment suppresses the error:
typescript// @ts-expect-error
console.log(20 * "Tim");
And here, the TypeScript compiler outputs an error:
typescript// @ts-expect-error
console.log(20*20);
Here, the code is valid, and the @ts-expect-error directive is unnecessary.
The TypeScript compiler outputs an "Unused '@ts-expect-error' directive." error.
@ts-ignore vs. @ts-expect-error
Since both comments suppress errors, it can be hard to choose between them.
Use @ts-expect-error when:
- You are writing test code.
- You expect a fix to be coming soon.
- You have a smaller project.
Use @ts-ignore when:
- You have a larger project.
- You are switching between TypeScript versions.
- You don't know which one to choose.
How to ignore all TypeScript compiler errors on a file?
To ignore all TypeScript compiler errors of a file, add a @ts-nocheck comment at the top of the file.
Here is an example of how to accomplish it:
typescript// @ts-nocheck
if (false) {
console.log("unreachable code");
}
This comment will silence all the errors in the file outputted by the TypeScript compiler.
Final thoughts
As you can see, ignoring TypeScript compiler errors is simple.
However, carefully consider and verify that you need it before doing so.
Usually, the TypeScript compiler shows errors for reasons, and you should only silence errors when you are 100% sure of what you are doing.
A code base should have a minimum number of TypeScript directive comments.
Since suppressing errors reduces TypeScript effectiveness, some teams implement a special Eslint rule (called ban-ts-comment) to disallow all TypeScript directive comments.
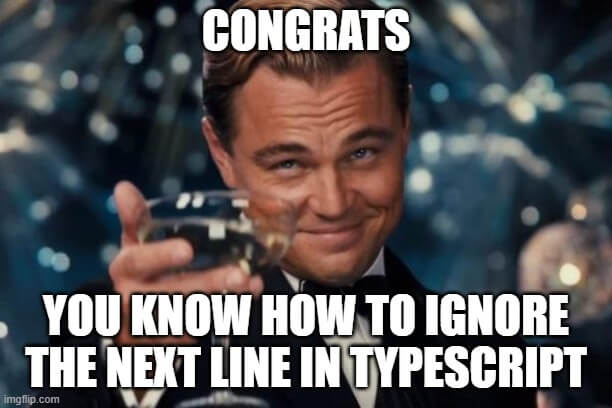
Here are some other TypeScript tutorials for you to enjoy: