How To Add Comments In TypeScript?
In programming, comments are a great way to add explanations to a piece of code, making it more readable and maintainable. But how do they work in TypeScript, and what is their syntax?
In TypeScript, you can create two types of comments:
- Single-line comments (start with //).
- Multi-line comments (start with /* and end with */).
This article explains both methods and describes the convention for TypeScript comments.
Let's get to it 😎.
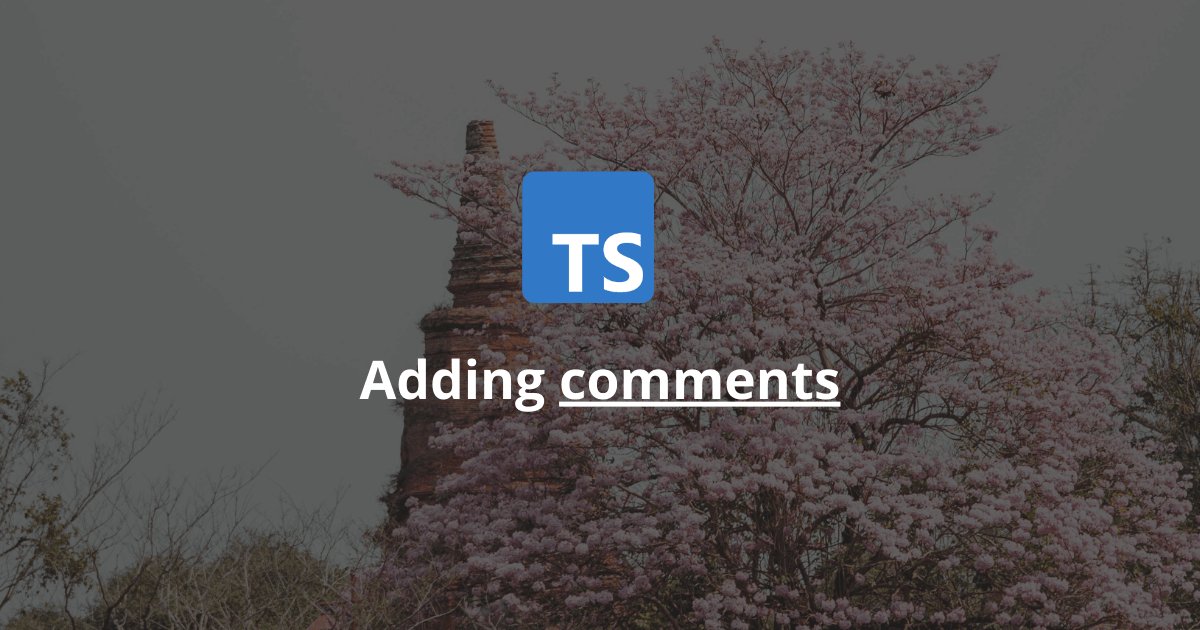
Why use comments in your code?
Comments provide a multitude of benefits to your code.
First, a comment can add explanations to a piece of code, saving investigation time in the long run.
Also, a comment can help you understand why the developer chose to do something in a particular way.
Other benefits of using comments in your code include the following:
- Allow developers to navigate through long pieces of code more efficiently.
- Help with the maintenance of your project.
- Allow developers to keep track of what needs to be done.
- Allow developers to comment out code to prevent its execution.
- And much more!
How to create a single-line comment?
To create a single-line comment in TypeScript, you must use the // characters before the comment.
Here is an example:
typescript// We define the x constant as 5.
const x = 5;
Or, we can add the same comment at the end of a line of code like that:
typescriptconst x = 5; // We define the x constant as 5.
Read more: How To Declare A Constant In TypeScript?
How to create a multi-line comment?
To create a multi-line comment in TypeScript, you must use the /* characters before the start of the comment and end it with the */ characters.
Here is an example:
typescript/*
This is a multi-line comment.
We define the x constant as 5.
*/
const x = 5;
What is the convention for documenting code?
In TypeScript, there are two documentation and comments specifications a developer can use:
- TSDoc
- JSDoc
Both specifications are similar, but nowadays, the recommended approach is for TypeScript developers to use TSDoc.
TSDoc is a specification that proposes to standardize the way developers write comments. It describes different tags that allow documenting pieces of code in a standard way.
Here is an example syntax to document a function using the TSDoc specification:
typescript/**
* Returns the sum of two numbers.
* @param x - The first input number
* @param y - The second input number
* @returns The sum of `x` and `y`
*/
const getSum = (x: number, y: number): number => {
return x + y;
}
As you can see, in TSDoc, a tag always starts with the @ character. In this case, this comment describes the parameters and the return type of the getSum function.
Final thoughts
As you can see, writing comments is easy in TypeScript.
They can provide context for other developers and save a lot of time.
Use them well!
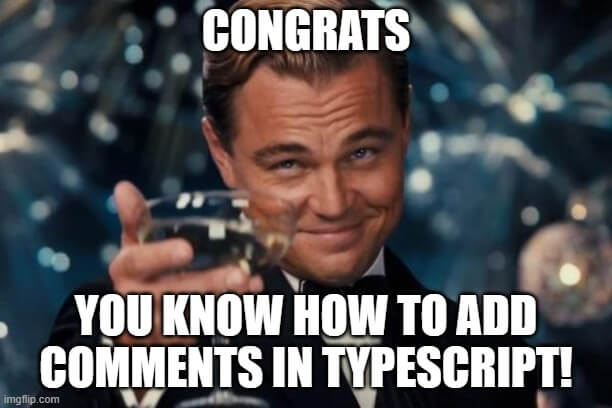
Here are some other TypeScript tutorials for you to enjoy: