How To Export A Function In TypeScript?
When working on a TypeScript repository, developers want to keep the files short, making it easier to navigate the code and debug. This leads to the TypeScript repository having many different files. For those TypeScript files to work together, they need to use each other's functions. Luckily, it is easy to export a function in TypeScript.
To export a function in TypeScript, you must use the export keyword like so:
typescriptexport const getName = (): string => {
return 'Tim';
}
This article will analyze all the different methods of exporting a function in TypeScript and answer some common questions.
Let's get to it 😎.
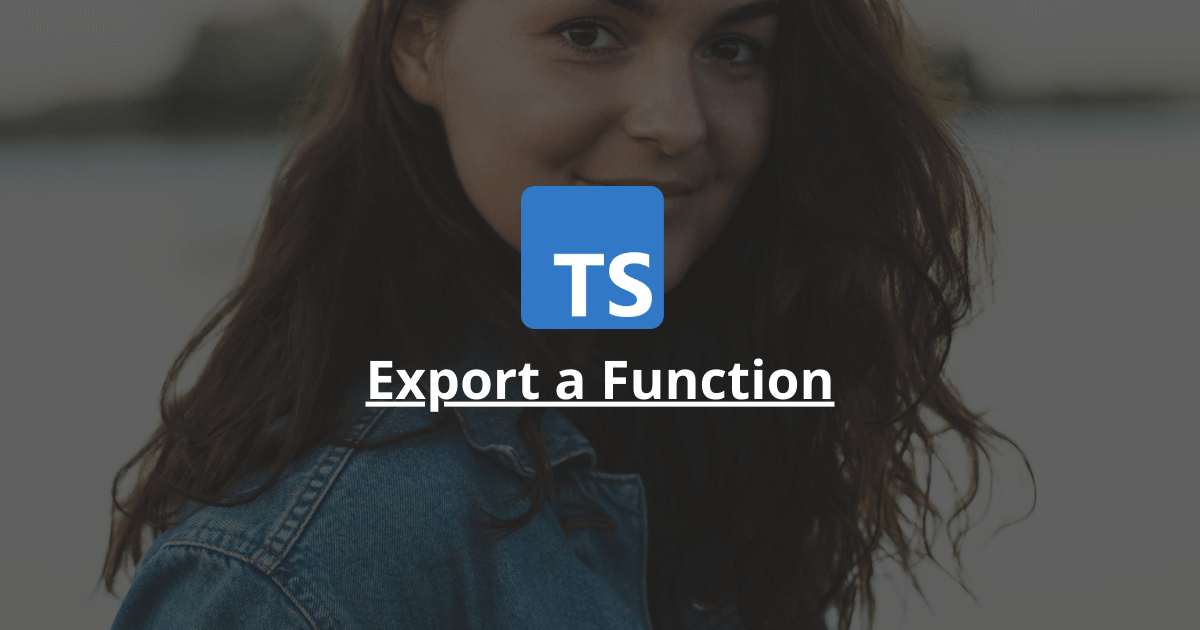
Here are the different ways that exist to export a TypeScript function:
- a declaration export (named)
- a list export (named)
- a default export
Each method is made for a different occasion and has advantages and disadvantages.
What is the difference between a named export and a default export?
In TypeScript, you can export a function as a named or a default export.
Here are the most significant differences between them:
- You can have multiple named exports in a file but only one default export.
- When importing a named export, you must use its original name.
- When importing a default export, you can use any name you want.
How to export a function as a declaration?
Exporting as a declaration is the easiest way to export a function.
Here is an example of a declaration export:
typescriptexport const getDate = (): number => Date.now();
And here is how to import the function into another file:
typescriptimport { getDate } from './file'
As you can see, you import the function using its original name.
How to export a function as a list?
Another option to export a function is to export it as a list.
You can export multiple entities using a list export.
Here is an example of a list export:
typescriptconst getDate = (): number => Date.now();
export {
getDate
}
Importing a list export item is the same as importing a declaration item:
typescriptimport { getDate } from './file'
Note: To import multiple items in the same import, separate the items by commas.
How to export a function as default?
Finally, you can export a function as a default export.
A TypeScript file can only contain one default export.
Here is an example of a default export:
typescriptconst getDate = (): number => Date.now();
export default getDate;
When you import this function, you can specify the original function name:
typescriptimport getDate from './file'
Or any name you want:
typescriptimport func1 from './file'
Final thoughts
As you can see, exporting a function in TypeScript is simple, and you have different methods of doing it.
In my day-to-day coding life, I only use declaration exports.
Default exports are not ideal because you can only have one in a file, and refactoring of default exports is not handled well by IDEs like VSCode.
On the other hand, list exports can be hard to follow if the file is big.
That's why I prefer to export the necessary functions when I need to, using declaration exports.
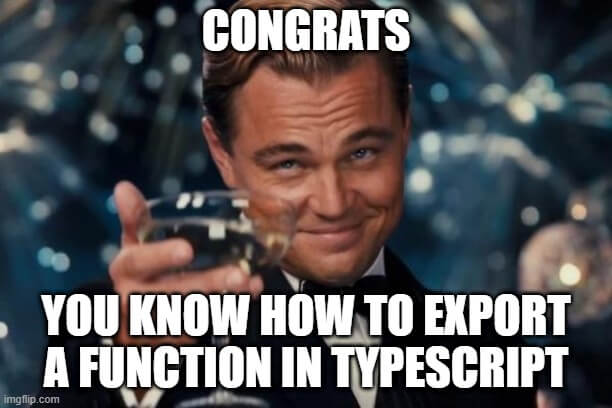
Here are some other TypeScript tutorials for you to enjoy: