How To Define A Singleton in TypeScript?
If you come from a computer science object-oriented programming background, you may want to utilize some of the design patterns you've always used but in TypeScript. One of the most well-known design patterns is the singleton.
A singleton is a pattern that ensures that only one instance of a class can exist. Luckily, Typescript provides a simple way to define one.
This article will go through the definition of a singleton, how to create one in TypeScript, and answer some common questions.
Let's get to it 😎.
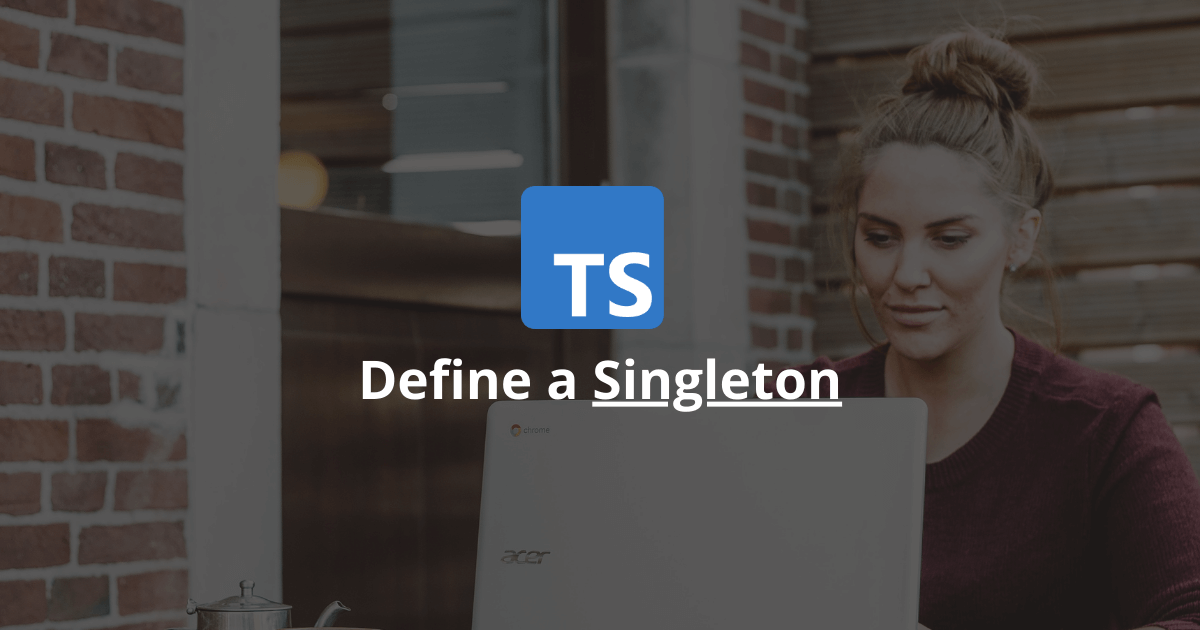
The definition
A singleton is a pattern that restricts a class from having more than one instance of itself.
You can think of a singleton as the OOP version of a global variable. When global variables store simple objects, singletons store more complex resources.
There are a few reasons to implement the singleton pattern in your code:
- When you need to manage a shared resource (think of a database connection).
- When a resource is going to be accessed by multiple parts of the code base.
- When you always need one instance of the resource.
- When you want to implement logging.
How to create a singleton?
To define a singleton in TypeScript, we need to:
- Restrict the usage of the new keyword on the class (using the new keyword on the class creates a new class instance.
- Provide a new way to instantiate the class.
And, TypeScript gives all the tools to do it!
Here is the code to implement a simple singleton:
typescriptclass Singleton {
private static instance: Singleton;
private constructor() { }
public static getInstance(): Singleton {
if (!Singleton.instance) {
Singleton.instance = new Singleton();
}
return Singleton.instance;
}
public doAction() {
console.log("action");
}
}
The static instance property holds the instance of the class.
To disallow the usage of the new keyword to instantiate the class, we mark the constructor as private.
Then, we define the getInstance method that instantiates the class if no class instance exists.
Here is how to use this class:
typescriptconst singleton1 = Singleton.getInstance();
const singleton2 = Singleton.getInstance();
// Outputs: true
console.log(singleton1 === singleton2);
// Outputs: 'action'
singleton1.doAction();
As you can see, both singletons refer to the same class instance.
Is the singleton an anti-pattern in TypeScript?
Some developers consider the singleton as an anti-pattern in TypeScript.
Here are some issues with the singleton pattern they cite:
- You cannot inherit from a singleton class.
- You have no control over the creation of the instance.
- You cannot get a "clean" class instance for testing purposes.
- You cannot do dependency injection.
That's why, before using this pattern, consider if one of those reasons is an issue for your specific case.
If not, use it when you need it.
Final thoughts
As you can see, defining a singleton in TypeScript is easy.
However, before defining one, you should consider the issues of using this pattern.
If it doesn't bother you, use it.
And if some of the issues are deal-breakers for you, use another pattern.
Luckily, TypeScript provides many other different ones.
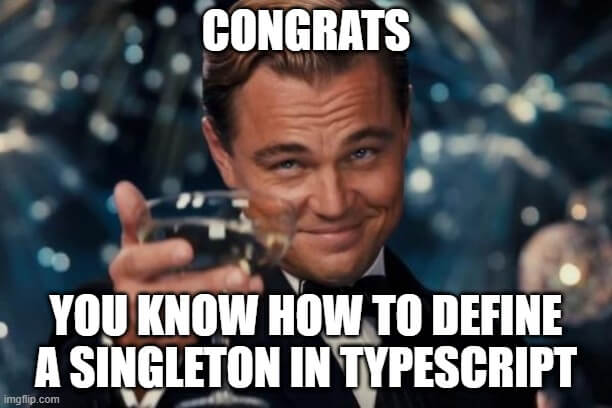
Here are some other TypeScript tutorials for you to enjoy: