Fixing The "Cannot Find Module" Error In TypeScript
Sometimes, when developing a TypeScript project and importing a new package, you get a "cannot find module" error. Luckily, this error is easy to fix.
There are many reasons why the "cannot find module" error can happen in TypeScript:
- The package is not installed.
- Something is wrong with the node_modules folder.
- The package import contains a spelling mistake.
- Something is wrong with your tsconfig.json file.
This article will analyze those four potential causes and show how to fix this error for each one of them.
Let's get to it 😎.
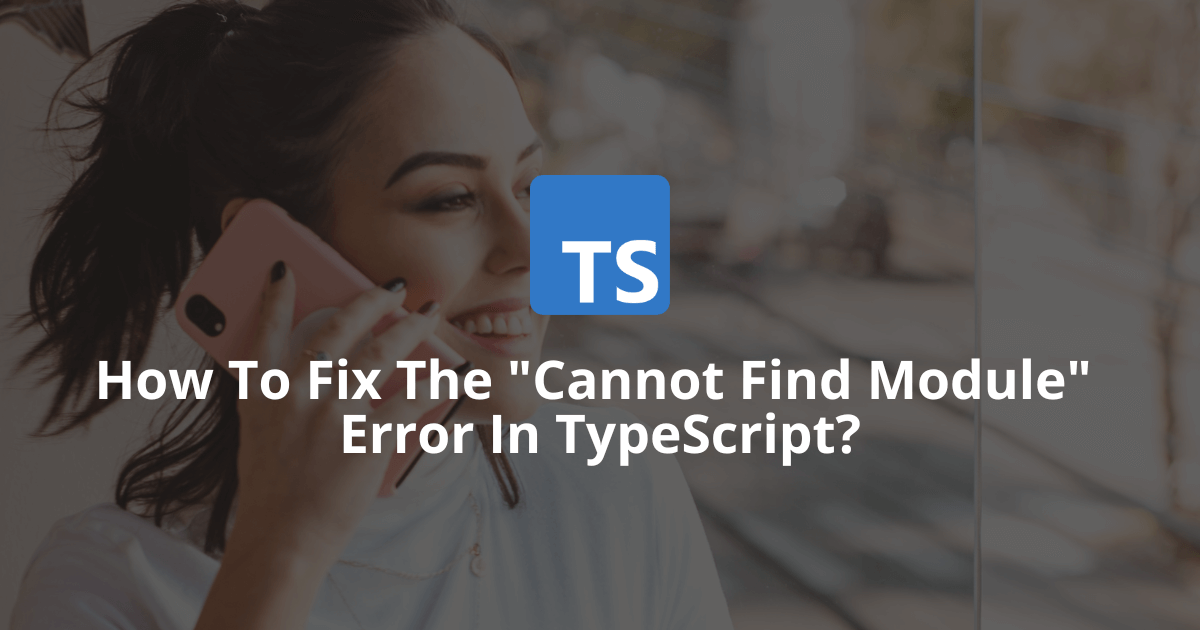
Here is how this error can look in your console:
bashCould not find a declaration file for module 'package/x'.
To fix this error, try those solutions one by one.
One of them will solve your error.
Solution #1 - Install the package
The first thing to fix the "cannot find module" error in TypeScript is to ensure that the package is installed on your system.
Run this command to install the package:
bashnpm install package-name
Also, some packages have a separate package with TypeScript types.
You need to install it as well, like so:
bashnpm install --save-dev @types/package-name
Solution #2 - Re-install your dependencies
Another potential fix for the "cannot find module" error is re-installing your dependencies. Indeed, something may be wrong with the project's node_modules folder.
Here's how to do it:
1. Remove the node_modules folder and the package-lock.json file, like so:
bashrm -rf node_modules package-lock.json
2. Install the dependencies like so:
bashnpm install
Solution #3 - Verify the import's name
This error can occur when you try building your project on a different OS than originally built. Indeed, various case sensitivity errors can occur.
You must verify the import in question and match it to the file path.
If your file path is: path/File.ts
Your import should be the same: path/File.ts
It should NOT be: path/file.ts
Note: Set forceConsistentCasingInFileNames to true, inside the tsconfig.json file, for this not to happen.
Solution #4 - Fix the tsconfig.json file
Maybe this error occurs because something is wrong with the tsconfig.json file.
You can try to set the moduleResolution to node, like so:
json{
"compilerOptions": {
"moduleResolution": "node",
// Rest
}
}
If it doesn't help, verify that your TypeScript file path is inside the include array AND is not inside the exclude array, like so:
json{
"include": ["src/**/*.ts", "tests/**/*.ts"],
"exclude": ["node_modules", ".vscode"],
// Rest
}
Or try adding a baseUrl, like so:
json{
"compilerOptions": {
"baseUrl": ".",
// Rest
}
}
Note: Sometimes, you must also add a valid paths entry for this solution to work.
Final Thoughts
As you can see, solving the "cannot find module" error in TypeScript is simple.
If it is a new dependency, it is usually a problem with the tsconfig.json file.
Otherwise, re-installing the dependencies will solve this error most of the time.
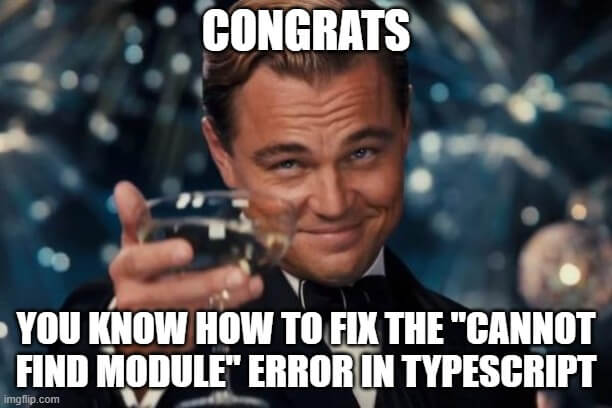
Here are some other TypeScript tutorials for you to enjoy: