How Does A Class Work In TypeScript?
A class is the fundamental building block of object-oriented programming languages like C# or Java. TypeScript also provides support for classes and gives developers access to such features as inheritance, encapsulation, and polymorphism.
In TypeScript, a class is an object that encapsulates data and functions to manipulate the data.
In this article, I explain how a TypeScript class work and show many code examples.
Let's get to it 😎.
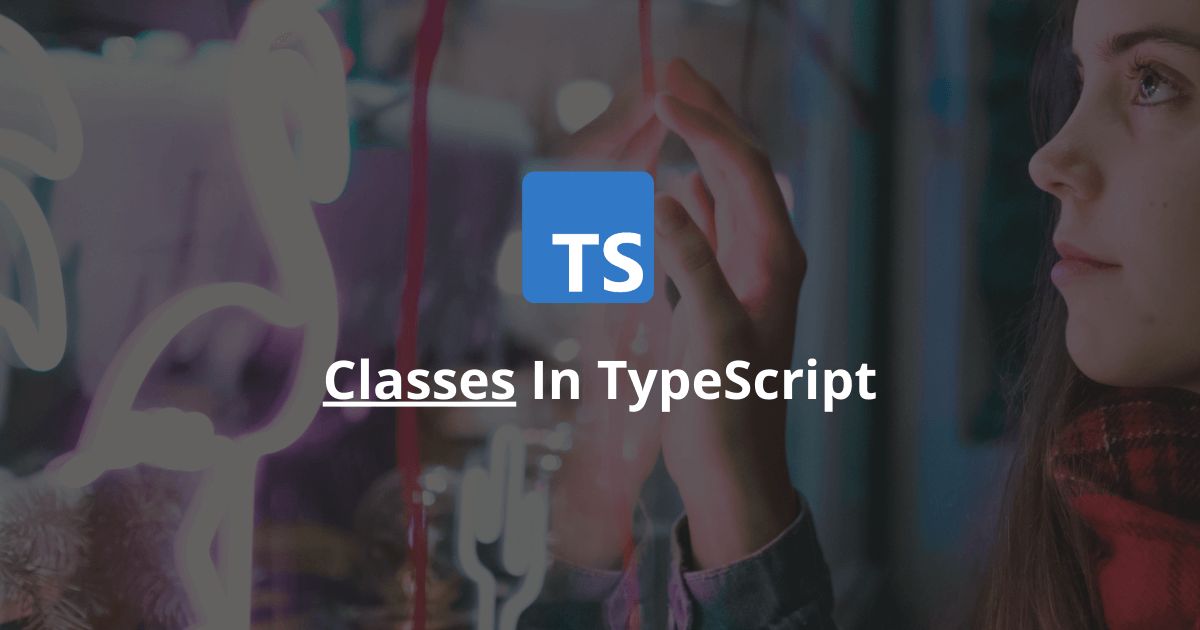
The definition
A class encapsulates data and functions that manipulate the data. It acts as a template for creating an object.
In TypeScript, you can define a class using the class keyword, like so:
typescriptclass Name {
}
A class can contain the following members:
- A constructor
- One or more methods
- One or more properties
Each class member can have an access modifier to limit its visibility.
A developer can extend a class, override a parent class method, or make a class implement an interface.
Also, a developer can define an abstract class or a static class.
Furthermore, you can use the singleton pattern inside a class.
The class constructor
The constructor is a special function, defined using the constructor keyword, called when instantiating a class. This method is used to initialize the properties and to set the class to its default state.
Here is an example:
typescriptclass Animal {
private name: string;
public constructor(name: string) {
this.name = name;
}
}
In TypeScript, a constructor can have multiple constructor overloads. Also, you can directly assign a value to a property inside the constructor using the constructor assignment feature.
Visibility
When defining a class member, a developer can provide an access modifier to limit its visibility.
TypeScript provides three different access modifiers:
- public - Allows access to the member to everyone. This access modifier is the default one.
- private - Only allows access to the member within the class.
- protected- Allows access to the member within the class or a derived child class.
P.S. For public members, explicitly setting the access modifier helps with the readability of your code.
Class inheritance
TypeScript allows a child class to inherit members from a parent class. This feature is called class inheritance.
To inherit parent members, use the extends keyword with the parent's name inside the child's class definition.
Here is an example:
typescriptclass Animal {
public constructor(
public name: string
) {}
public sleep(): void {
console.log(`${this.name} is sleeping.`);
}
protected addYear (): void {
console.log(`${this.name} is now older.`);
}
}
class Dog extends Animal {
public bark(): void {
console.log(`${this.name} is barking.`);
}
}
const dog = new Dog('Doggy');
// Outputs: "Doggy is sleeping."
dog.sleep();
// Outputs: "Doggy is barking."
dog.bark();
In this example, the Dog class inherits all the members from the Animal class.
The addYear function can only be called within the Dog or Animal classes but not from outside because it has the protected access modifier.
Defining a class property
TypeScript allows defining class properties easily. To define one, you need to use an access modifier to set the property's visibility, and set its name and type. You can also directly initialize its value or set it later.
Here is an example:
typescriptclass Person {
private name: string;
public constructor(name: string) {
this.name = name;
}
}
const person = new Person('Tim');
In this example, the name member is a class property.
Defining a class constant
Also, TypeScript allows developers to define class constants using the read-only keyword. A read-only property must be declared inside the property declaration or the constructor.
Here is an example:
typescriptclass Dog {
readonly PROPERTY = 2;
}
Read more: How To Declare Class Constants In TypeScript?
Constructor assignment
Like many other OOP languages, TypeScript lets us quickly define class properties by putting them inside the constructor definition. This technique is called constructor assignment. To use this technique, a developer needs to put an access modifier before a parameter's name.
Here is an example:
typescriptclass Person {
public constructor(
private name: string,
private age: number
) {}
}
const person = new Person('Tim', 27);
This code is equivalent to this:
typescriptclass Person {
private name: string;
private age: number;
public constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
}
const person = new Person('Tim', 27);
As you can see, by defining the properties as class parameters, we don't need to initialize them.
Making a class implement an interface
To make a class implement an interface, a developer needs to define the interface and the class AND use the implements keyword on the class definition.
Here is an example:
typescriptinterface IBird {
fly: () => void;
}
class Hummingbird implements IBird {
public constructor() { }
public fly () {
console.log('The hummingbird is flying.');
}
}
const hummingbird = new Hummingbird();
// 'The hummingbird is flying.'
hummingbird.fly();
A class that implements an interface must implement ALL the members defined inside the interface, or the TypeScript compiler will output an error.
Read more: How To Make A Class Implement An Interface In TypeScript?
Overriding a class function
TypeScript allows overriding a parent function inside a child class easily. To override a parent function, a developer must define a function in the child class of the same name.
Here is an example:
typescriptclass Car {
public drive(km: number): void {
console.log(`Your car drove ${km}km.`);
}
}
class Ferrari extends Car {
public override drive(km: number): void {
console.log(`Your Ferrari drove ${km}km.`);
console.log('override method.')
}
}
const ferrari = new Ferrari();
// Output: "Your Ferrari drove 2km."
// Output: "override method."
ferrari.drive(2);
In this example, we override the drive function of the parent Car class.
Read more: How To Override A Method In TypeScript?
Final thoughts
As you can see, defining a class is easy in TypeScript.
Regarding when you should use one, some developers use them a lot, and some prefer functional programming and are against TS classes. It largely depends on your team, your needs, and if you want to use OOP features.
In my day-to-day life, I use TypeScript classes a lot on the back end and rarely on the front end.
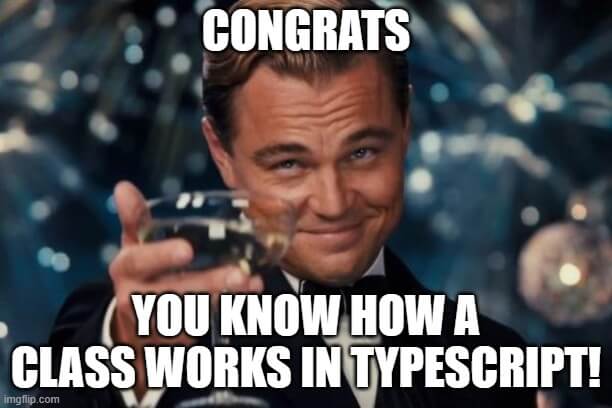
Here are some other TypeScript tutorials for you to enjoy: