How To Add Multiple Constructors In TypeScript?
If you come from a C# background, you may want to add multiple constructors to a TypeScript class. Although TypeScript doesn't support multiple constructors, you can still achieve similar behavior.
In TypeScript, you can achieve a similar behavior to adding multiple constructors by:
- Adding constructor overloads AND implementing a custom type guard.
- Using the static factory method to construct a class.
- Adding a partial class argument to the constructor.
This article explains those solutions with code examples.
Let's get to it 😎.
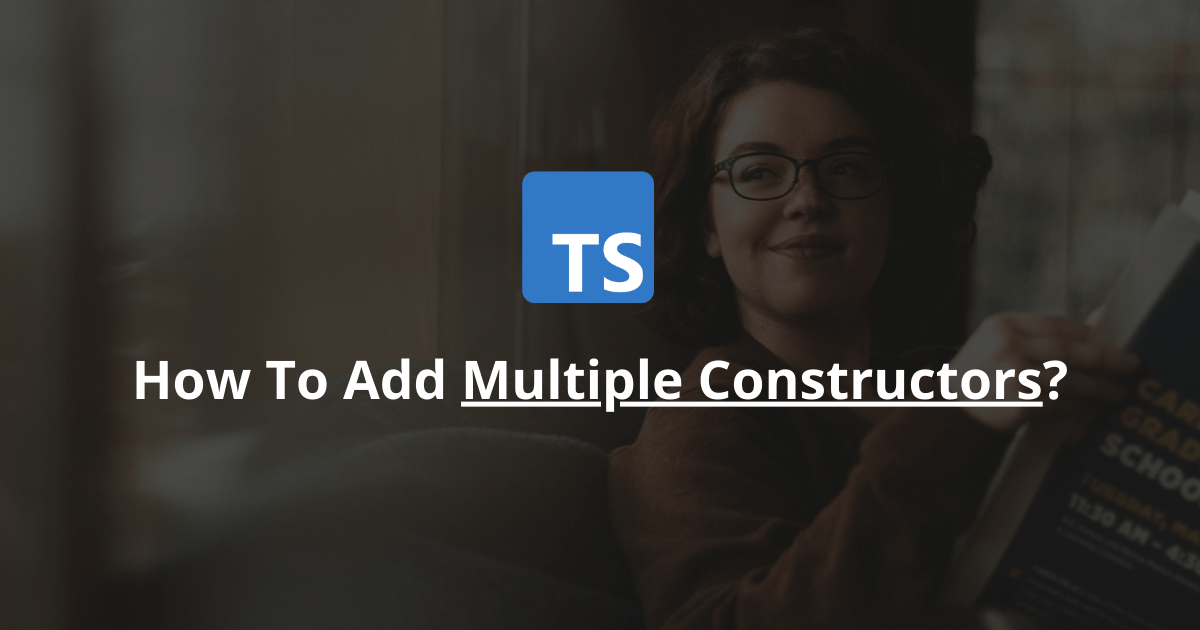
In TypeScript, unlike other languages, you cannot define multiple constructors inside a class. However, you can achieve similar behavior by using one of those alternative methods.
How to implement constructor overloads?
One way to simulate adding multiple constructors involves:
- Defining multiple constructor overloads inside a class.
- Adding the primary constructor implementation, so it supports the other constructor overloads.
- Checking the passed arguments with a custom type guard.
Here is an example:
typescriptclass MyClass {
private p1: string;
private p2: number = 0;
constructor(p1: string);
constructor(p1: string, p2?: number) {
this.p1 = p1;
if (p2) {
this.p2 = p2;
}
}
}
const cls1 = new MyClass('Tim');
const cls2 = new MyClass('Tim', 27);
As you can see, in this example, we declare one constructor overload and make the constructor implementation compatible with it.
And this is a more complex example with three constructor overloads:
typescriptclass MyClass {
public constructor(p1: number);
public constructor(p1: string, p2: string);
public constructor(p1: number, p2: string, p3: string);
public constructor(...arr: any[]) {
if (arr.length === 2) {
console.log('two arguments constructor called.');
} else if (arr.length === 3) {
console.log('three arguments constructor called.');
} else if (arr.length === 1) {
console.log('one argument constructor called.');
}
}
}
As you can see, using constructor overloads in TypeScript can quickly become hard to manage and understand. That's why I prefer the following method better.
How to use the factory method to construct a class?
The factory method to simulate multiple constructors is much simpler in TypeScript. It involves adding static factory functions to the class that will instantiate it with the correct arguments.
Here is an example:
typescriptclass MyClass {
public p1: string = '';
public p2: number = 0;
public static fromOneValue(p1: string): MyClass {
const cls = new MyClass();
cls.p1 = p1;
return cls;
}
public static fromTwoValues(p1: string, p2: number): MyClass {
const cls = new MyClass();
cls.p1 = p1;
cls.p2 = p2;
return cls;
}
}
const cls1 = MyClass.fromOneValue('Tim');
const cls2 = MyClass.fromTwoValues('Tim', 27);
In this example, instead of adding constructor overloads, we add two static factory functions that populate a new class instance with the correct arguments and return it.
This method is much simpler to comprehend and maintain.
How to add a partial class argument to the constructor?
Finally, you can pass a partial class as the constructor's argument. That way, you can pass any argument you want to the class.
Here is an example:
typescriptclass MyClass {
private p1: string = '';
private p2: number = 0;
constructor(params: Partial<MyClass>) {
Object.assign(this, params);
}
}
const cls1 = new MyClass({ p1: 'Tim' });
const cls2 = new MyClass({ p1: 'Tim', p2: 27 });
As you can see, we can pass any class-defined argument to the constructor.
The disadvantage of this method is that since you can pass any argument you want, you can omit a required parameter.
Final thoughts
As you can see, it is easy to simulate multiple constructors in TypeScript.
As for myself, most of the time, I use the factory or the partial argument class method.
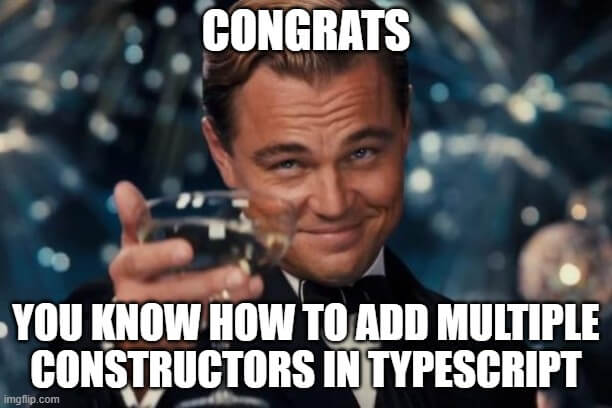
Here are some other TypeScript tutorials for you to enjoy: