How Does The keyof Operator Work In TypeScript?
The keyof operator is one of the two type operators (the other one is the typeof operator) offered by TypeScript to facilitate type management.
The keyof operator is used to extract keys from a specific type into a union type.
In this article, you will learn:
- How to use the keyof operator?
- When to use the keyof operator?
- What are some use cases for the keyof operator?
Let's get to it!
How does the keyof operator work?
The keyof operator extracts the keys of the provided type and returns them in a new union type.
Here is an example of the keyof operator in action.
typescriptinterface IArticle {
content: string;
authorId: number;
category: string;
}
// 'content' | 'authorId' | 'category'
type ArticleKeys = keyof IArticle;
In this example, we have extracted the keys of the IArticle interface into a new type. The new type ArticleKeys is a union type of all IArticle keys.
Did you notice that we are using an interface with type... I've written an extensive article about the difference between a type vs an interface.
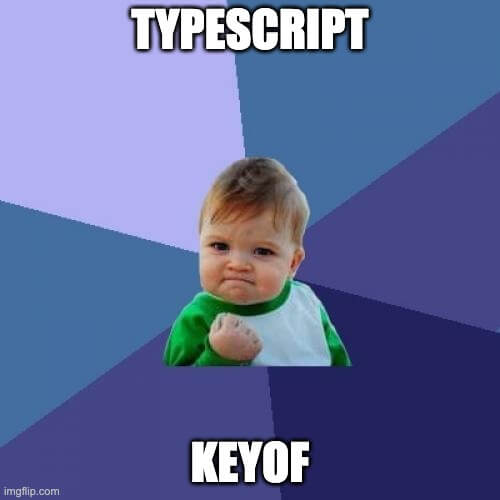
The keys produced by the keyof operator can be either of type string or number, depending on the index of the type.
What does typeof keyof mean?
When used in combination with the typeof operator, the keyof operator returns a union type of all the keys of an object.
typescriptconst article = {
content: 'content',
authorId: 123,
category: 'misc',
};
type ArticleKeys = keyof typeof article;
Since article is not a type but an object, you first need to get the type of the object using typeof and then use keyof to extracts its keys.
What does in keyof mean?
The in keyof keywords are used inside mapped types. Mapped types are a TypeScript feature that helps create a new type based on an old type.
Using mapped types, you can, for example, create a type that will make all properties optional.
typescripttype CustomPartial<T> = {
[P in keyof T]?: T[P];
};
In this example, we have created a new type, that makes all the properties of another type optional.
How to get the keyof values inside an array?
To extract the values from objects contained inside an array you can use a combination of the const assertions and lookup type TypeScript features.
typescriptexport const articleList = [
{ name: 'article1' },
{ name: 'article2' },
{ name: 'article3' }
] as const;
// 'article1' | 'article2' | 'article3'
type Name = typeof articleList[number]['name'];
In this example, we are using a lookup type to first get inside the articleList array and then extract all name properties as a new union type.
The as const means that the constant becomes a fully read-only object. It is also known as const assertion.
How to use the keyof operator on an enum?
To extract all the keys from an enum you will need to use a combination of the keyof operator with the typeof operator.
typescriptenum UserRole {
admin,
manager,
}
// 'admin' | 'manager'
type UserRoleKeys = keyof typeof UserRole;
Is there a valueof operator similar to the keyof operator?
Unfortunately, there is no operator like that in TypeScript. However, you can recreate the desired behavior, by using a combination of the generics and the indexed access types TypeScript features.
typescriptconst Article = {
content: 'content',
authorId: 123,
category: 'misc',
} as const;
type ValueOf<T> = T[keyof T];
// 'content' | 123 | 'misc'
type ArticleValues = ValueOf<typeof Article>;
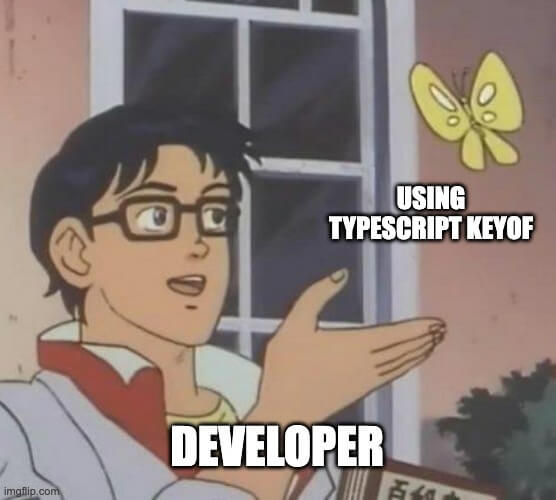
In this example, we create a helper generic new type called ValueOf and we use it to create the ArticleValues type that contains a union of all Article keys.
Conclusion
One of the reasons, that I like TypeScript so much is that it offers a lot of little features that simplify the developer's coding life. One of those features, that I use almost daily, is the keyof operator. It is very useful when you need to extract keys from a type.
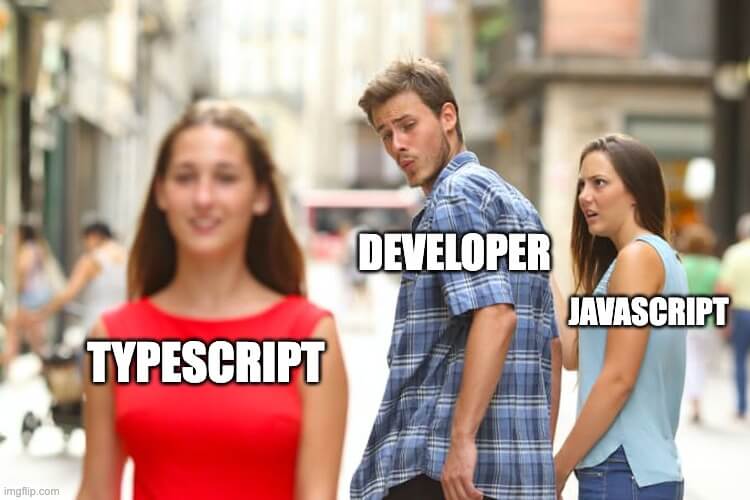
After reading this article, I hope you understand it well and will start to use it more.
I hope you liked the article, please share it with your fellow developers.