How Does The As Keyword Work In TypeScript?
TypeScript adds strong typings to your JavaScript. Everything has its type in TypeScript. However, the compiler is sometimes incorrect and infers the wrong type. Luckily, TypeScript offers a special keyword to cast object types.
The as keyword converts an object's type into a different one.
typescriptconst txt = document.getElementById("txt") as HTMLInputElement;
This article explains everything about the as keyword and shows real-life TypeScript code examples of its uses.
Let's get to it 😎.
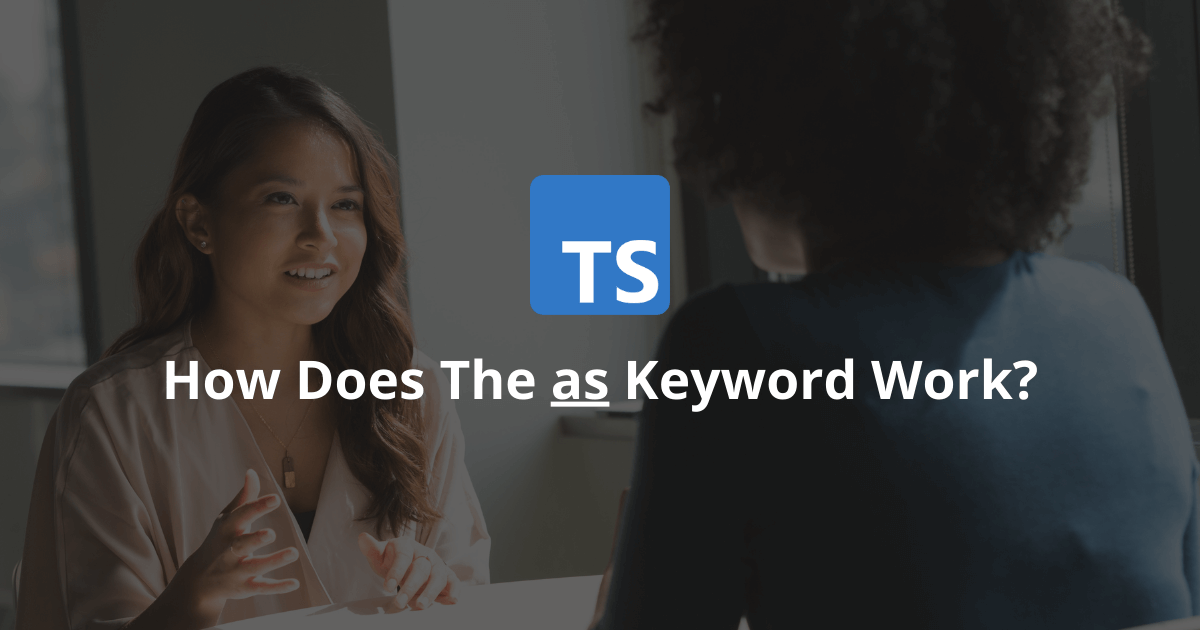
The definition
The as keyword, first introduced in TypeScript version 1.6, is used to:
- Do a type assertion (Cast an object's type, Create a type predicate)
- Do a const assertion (To set object's properties as read-only)
In sum, this keyword casts an object's type to a different type that the compiler inferred. You should note that this keyword will be removed when the code gets compiled down to JavaScript.
Let's have a small example:
typescriptconst txt: unknown = 'text';
console.log((txt as string).length);
In this example, we cast an unknown object to be a string.
Here is another way of writing the same example:
typescriptlet x: unknown = 'hello';
console.log((<string>x).length);
However, casting using the as keyword is the recommended way.
When to use the as keyword?
Use this keyword when you need to cast a type or create a type predicate.
One of the most common examples of where you might need to use the as keyword is when getting DOM elements. Indeed, in TypeScript, the getElementById function returns HTMLElement. But what if you have an input or a checkbox? You need to cast that type to a more specific type.
Here is how to do it:
typescriptconst txt = document.getElementById("txt") as HTMLInputElement;
As you can see, we transform the HTMLElement type into an HTMLInputElement to access functions and properties specific to an input element.
If you try to cast an object's type into an invalid one (not a subset or superset of the expected type), you will get a TypeScript error.
typescriptconst num = document.getElementById("txt") as number;
This code outputs a TypeScript error.
How to force cast a type?
Sometimes, you know better than the TypeScript compiler.
To force cast a type, you must first cast it to be unknown and then to the type you want.
typescriptconst num = document.getElementById("txt") as unknown as number;
⚠️ Only use this method when you know what you are doing. It can cause bugs.
What does as const mean in TypeScript?
Using as const means that you want the object to be fully readonly. This is also known as const assertion.
Let's say we have an array with a few elements:
typescriptconst array = [ 2, 4 ];
// This works.
console.log(array[0]);
// This works.
console.log(array[1]);
// But this also works.
console.log(array[2]);
You can access the array at index two because TypeScript assumes that the array's type is number[].
But what if the array is never going to change? Then, you need to indicate to the compiler that you want to treat it as a readonly tuple.
typescriptconst array = [ 2, 4 ] as const;
// This works.
console.log(array[0]);
// This works.
console.log(array[1]);
// This gives an error.
console.log(array[2]);
That way, TypeScript knows how to validate it correctly.
Note: To make a read-only property use the TypeScript readonly keyword.
Final thoughts
As you can see, casting a type is easy in TypeScript.
However, always triple-check your code when you cast types because introducing bugs becomes simpler.
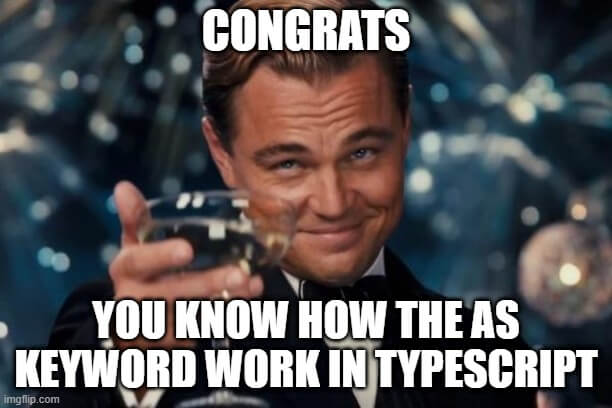
Here are some other TypeScript tutorials for you to enjoy: