How To Find The Class Name Of An Object In TypeScript?
Sometimes, a TypeScript developer may need to find the class name of an object. Luckily, it is easy to do.
To find the class name of an object at runtime in TypeScript, you can:
- Use the constructor property of the class.
- Add a class constant with the name.
This article analyses both solutions and shows how to code them with real-life TypeScript examples.
Let's get to it 😎.
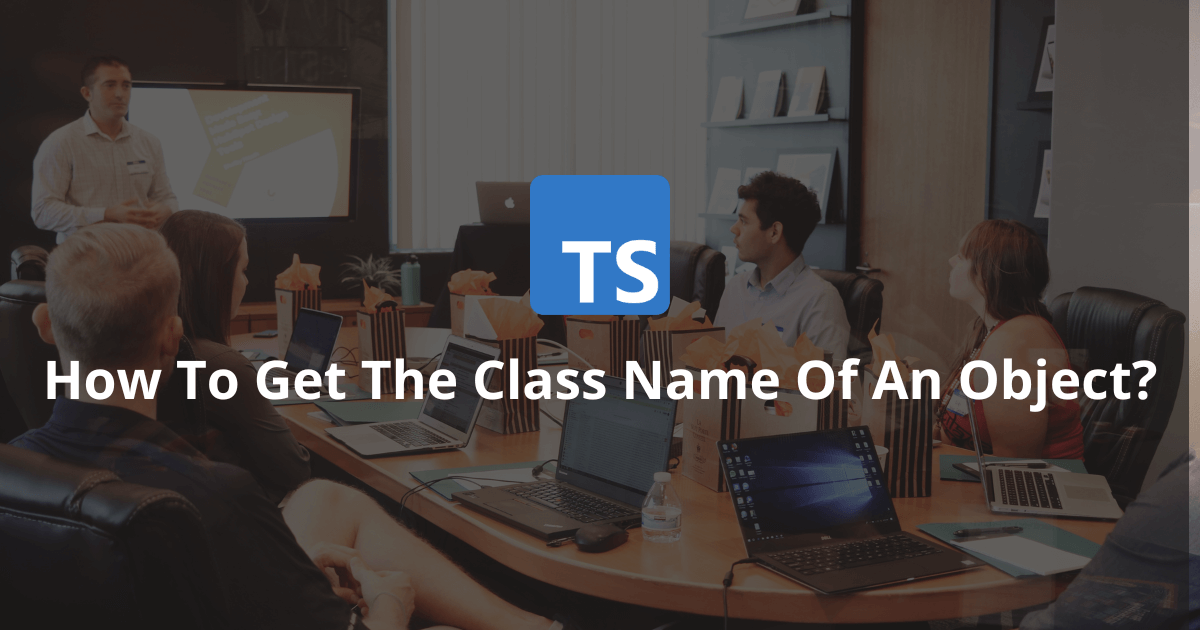
Method #1 - Use the constructor property
The easiest way to get the name of a class in TypeScript involves using the constructor property.
This property returns a reference to the constructor that created the class.
Using the constructor property, we can determine the name of the class, like so:
typescriptclass Animal { }
const animal = new Animal();
// Outputs: Animal
console.log(animal.constructor.name);
Note: This method doesn't work if you have a property named name in your class.
However, this solution has a significant downside.
When you minify this code, the class name can change (each time you run the minification process).
This is where the next solution comes into play.
Method #2 - Add a class constant with the name
A better solution to using the constructor is adding a class constant with the class name.
Here is how you can do it:
typescriptclass Animal {
public readonly NAME = 'Animal';
}
const animal = new Animal();
// Outputs: Animal
console.log(animal.NAME);
In this example, we declare a class called Animal.
Then we add a read-only class constant containing the class's name (we don't want to change the class name, that's why it is read-only).
Finally, we print the class name by accessing the NAME property.
This method has the advantage of being bulletproof to minification and uglification.
Read more: How does the readonly keyword in TypeScript?
Final Thoughts
As you can see, getting the name of a class is easy in TypeScript.
I recommend using the class constant method.
If you want to use the other method with code minification, you must set up an exception for the class name in your configuration file.
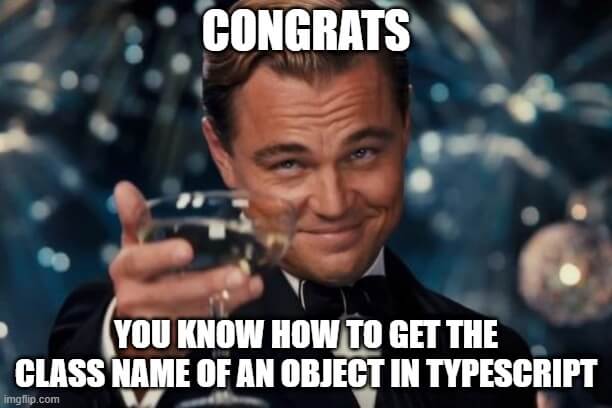
Here are some other TypeScript tutorials for you to enjoy: