What Are Union Types In TypeScript?
TypeScript offers multiple types to compose existing types into new ones, such as the intersection type or the union type.
The union type allows a developer to use more than one data type as a parameter or a variable.
This article will explain union types in TypeScript and give real-life code examples to understand this feature better.
Let's get to it 😎.
The definition
The union type lets a value be of more than one type.
To represent a union type, use the pipe | symbol.
Here is the syntax of a union type in TypeScript:
typescriptlet variable: string | number | boolean;
Let's go through some examples to understand this better.
typescriptlet obj: string | number;
obj = 2805; // OK
obj = "Tim"; // OK
obj = {}; // Not OK
obj = false; // Not OK
The obj variable only accepts strings or numbers as an argument in this example. That's why it gives a compiler error when you try to assign a non-supported data type to it.
You can also use union types with function agruments:
typescriptconst output = (param: number | string): void => {
console.log(param);
};
output(2805); // OK
output('Tim'); // OK
output(true); // Not OK
As you can see, this function accepts a parameter that can be either a number or a string.
When you try to call it with something else, it outputs a TypeScript compiler error.
Union of literal types
Also, TypeScript allows the creation of union types of literals, such as:
- String literal
- Number literal
- Boolean literal
Here is an example of a union of string literals:
typescripttype Animal = 'dog' | 'cat' | 'cow';
In this example, we create a new type that only accepts a limited set of string values.
Union type vs enums
As you may have noticed, the union type and the enum are similar.
The most significant differences between union types and enums are:
- Union types are a compile-time concept. They are not outputted in the final JavaScript.
- Enums are transpiled to JavaScript.
- You can iterate over an enum, but not the union type.
- You can use an enum as a bit flag.
- Enums are less type-safe than union types.
- Enums can have redundant declarations.
How to check a type against a union type?
To check a type against a union type, you need to know more about the object you are checking.
For example, if an object contains a specific property or function, you can check for it to determine the correct object type.
Here is an example of this:
typescriptinterface IDog {
bark: () => void;
}
interface ICat {
meow: () => void;
}
const buy = (animal: IDog | ICat): void => {
if ('meow' in animal) {
console.log('cat');
} else {
console.log('dog');
}
}
Since only the cat contains the meowing function, you can check for its existence to determine the animal's type.
Read more: How do interfaces work in TypeScript?
Final thoughts
As you can see, TypeScript union types are helpful when a developer needs a variable to accept more than one data type.
Union types are different from enums because they only exist at compile-time.
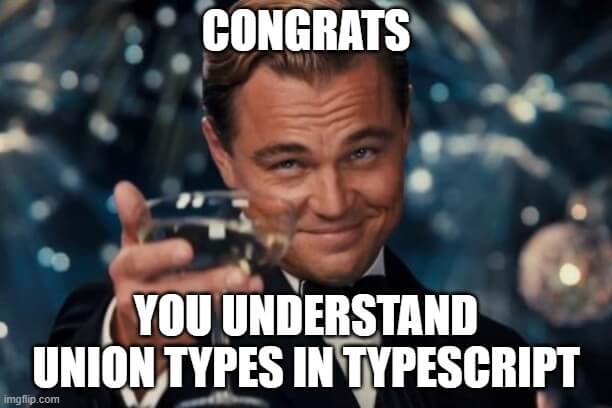
Thank you for reading this article.
Here are other TypeScript tutorials for you to enjoy: